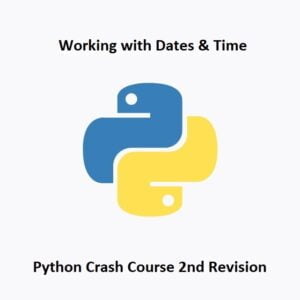
Python Dates and Time
Dealing with dates and time is a fundamental aspect of many programming tasks, from scheduling events to data analysis. Python offers robust libraries and modules to make working with dates and time seamless. In this part of our Python crash course, we’ll explore how to handle, manipulate, and format dates and time in Python.
The time
Module for Delays
Python’s time
module is a valuable tool for creating delays or pausing your program’s execution for a specific duration. You can use the sleep()
function to introduce delays. Here’s an example:
import time print("This is printed immediately.") time.sleep(2) # Pause for 2 seconds print("This is printed after a 2-second delay.")
In this code, we import the time
module and use the sleep()
function to introduce a 2-second delay between two print statements.
The datetime
Module
Python’s datetime
module is a powerful tool for working with dates and times. It provides classes for dates, times, and date-time combinations. Here’s how you can use the datetime
module to work with dates:
from datetime import datetime # Get the current date and time current_datetime = datetime.now() # Format the date and time formatted_datetime = current_datetime.strftime("%Y-%m-%d %H:%M:%S") print(f"Current date and time: {formatted_datetime}")
In this example, we import the datetime
module, obtain the current date and time, and format it to display as a string.
The strftime
Method for Formatting
The strftime
function in Python is used to format date and time objects as strings. It allows you to create custom date and time representations by specifying various format codes as arguments. Here’s a detailed explanation of the most commonly used formatting arguments:
%Y
: Year with century as a decimal number (e.g., “2023”).%y
: Year without century as a decimal number (e.g., “23”).%m
: Month as a zero-padded decimal number (e.g., “04” for April).%B
: Full month name (e.g., “April”).%b
: Abbreviated month name (e.g., “Apr”).%d
: Day of the month as a zero-padded decimal number (e.g., “09”).%A
: Full weekday name (e.g., “Wednesday”).%a
: Abbreviated weekday name (e.g., “Wed”).%H
: Hour (24-hour clock) as a zero-padded decimal number (e.g., “08”).%I
: Hour (12-hour clock) as a zero-padded decimal number (e.g., “03”).%p
: AM or PM designation (e.g., “AM” or “PM”).%M
: Minute as a zero-padded decimal number (e.g., “05”).%S
: Second as a zero-padded decimal number (e.g., “09”).%f
: Microsecond as a decimal number, zero-padded on the left (e.g., “123456”).%j
: Day of the year as a zero-padded decimal number (e.g., “094”).%U
: Week number of the year (Sunday as the first day of the week) as a zero-padded decimal number (e.g., “05”).%W
: Week number of the year (Monday as the first day of the week) as a zero-padded decimal number (e.g., “05”).%c
: Locale’s appropriate date and time representation (e.g., “Wed Apr 12 08:05:09 2023”).%x
: Locale’s appropriate date representation (e.g., “04/12/23” in the US).%X
: Locale’s appropriate time representation (e.g., “08:05:09” in the US).%Z
: Time zone name (e.g., “UTC” or “Eastern Daylight Time”).%%
: A literal “%” character.
These format codes are used within the strftime
method to specify how the date and time should be formatted in the resulting string. For example, to format a date as “Wednesday, April 12, 2023,” you can use the following code:
from datetime import datetime current_datetime = datetime.now() formatted_datetime = current_datetime.strftime("%A, %B %d, %Y") print(formatted_datetime)
The %A
, %B
, and %d
format codes are used to extract the day of the week, full month name, and day of the month from the current_datetime
object and format them accordingly.
You can combine these format codes to create custom date and time representations that meet the requirements of your application.
Date Arithmetic
You can perform arithmetic operations on dates and times, such as adding or subtracting time intervals. Here’s an example of date arithmetic in Python:
from datetime import datetime, timedelta # Get the current date current_date = datetime.now() # Calculate a date in the future (e.g., one week from now) one_week_later = current_date + timedelta(weeks=1) print(f"One week from now: {one_week_later.strftime('%Y-%m-%d')}")
This code demonstrates how to calculate a date in the future by adding a time delta.
Parsing Dates
You can also parse and convert date strings into datetime
objects. This is particularly useful when working with date input from users or external sources. Here’s an example:
from datetime import datetime date_str = "2023-11-15" parsed_date = datetime.strptime(date_str, "%Y-%m-%d") print(f"Parsed date: {parsed_date}")
This code parses a date string and converts it into a datetime
object.
Time Zones
Python’s pytz
library allows you to work with time zones, making it possible to handle dates and times from different regions. You can convert between time zones, calculate time differences, and perform other time zone-related operations.
Handling Timestamps
Timestamps are often used in data analysis and database operations. Python allows you to convert between datetime
objects and Unix timestamps (seconds since January 1, 1970). This enables you to work with time data in different formats.
Conclusion
In this part of our Python crash course, we’ve delved into the world of Python’s date and time handling capabilities. From the versatile datetime
module to the powerful strftime
method, you’ve learned how to manipulate, format, and control dates and times with precision. Whether you’re scheduling tasks, analyzing data, or presenting information to users, these skills will prove invaluable in your programming journey.
By mastering these fundamental concepts, you’ve taken another step towards becoming a proficient Python programmer. In our next installment of the Python crash course, we’ll explore working with Files and Data, error handling and exceptions, equipping you with the knowledge to write robust and error-tolerant code. Stay tuned for more Python programming knowledge!
That’s All Folks!
You can explore more of our Python guides here: Python Guides