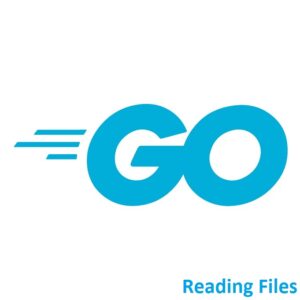
Unveiling File Handling and I/O Operations
Reading files is a fundamental operation in programming. This guide delves into file handling in Golang, uncovering methods and techniques to efficiently read and process data from files, empowering developers to manipulate external data seamlessly.
Using Packages for File Handling
In Go, you can read files using the os
and bufio
packages to efficiently handle file I/O. Here’s a step-by-step guide on how to read files in Go:
Import the necessary packages:
import ( "bufio" "fmt" "os" )
Open the file for reading using os.Open()
:
file, err := os.Open("file.txt") if err != nil { fmt.Println("Error opening file:", err) return } defer file.Close() // Make sure to close the file when you're done
Create a Scanner
to read the file line by line (if it’s a text file):
scanner := bufio.NewScanner(file) for scanner.Scan() { line := scanner.Text() // Process the line as needed fmt.Println(line) } if err := scanner.Err(); err != nil { fmt.Println("Error reading file:", err) }
If you want to read the entire file at once, you can use ioutil.ReadFile()
:
data, err := ioutil.ReadFile("file.txt") if err != nil { fmt.Println("Error reading file:", err) return } content := string(data) fmt.Println(content)
Handling File Read Errors and Best Practices:
Error Checking:
Error checking during file reading operations is crucial to ensure the reliability and stability of the application. It involves verifying the success of file open, read, or seek operations and handling potential errors gracefully.
Error Handling Example:
file, err := os.Open("filename.txt") if err != nil { // Handle error, log, or return }
Resource Management:
Proper resource management includes closing files after reading to release system resources and prevent memory leaks. Utilizing defer
for file closure ensures that resources are cleaned up regardless of how the function exits.
Defer and Close Example:
file, err := os.Open("filename.txt") if err != nil { // Handle error } defer file.Close() // Defer file closure // Read and process file content
Efficiency Considerations:
For optimized file reading, especially with large files or streams, considerations include using buffered reading techniques (bufio
package), avoiding unnecessary reads, and minimizing seek operations for enhanced performance.
Buffered Reading Example:
file, err := os.Open("filename.txt") if err != nil { // Handle error } defer file.Close() reader := bufio.NewReader(file) // Buffered reader // Use reader for efficient reading
Conclusion
File handling and reading in Go are essential skills for effective data processing and manipulation. By mastering file reading techniques, developers gain the ability to efficiently extract, process, and manage data from external files within their Go applications.
Remember to handle errors appropriately when reading files to ensure your program behaves gracefully when encountering issues like file not found or permission problems.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang