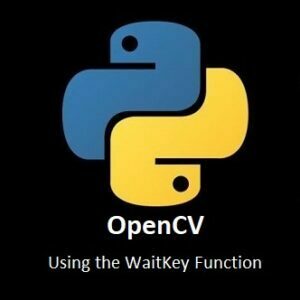
Step into the realm of real-time interaction with OpenCV
Let’s dive deeper into the indispensable waitKey()
function. In this OpenCV for Beginners guide, we unlock the power of synchronization, understanding how this function empowers us to control the pace of our computer vision applications. Whether you’re navigating through video streams, handling user input, or implementing dynamic image processing, waitKey()
is your gateway to seamless interactivity. Let’s embark on another OpenCV with Python journey where time becomes a variable, and each keystroke orchestrates the symphony of visual computation.
What is the waitKey()
Function?
The waitKey()
function in OpenCV is used to introduce a delay in the program and also to capture and process keyboard events. It waits for a specified amount of time for a key event to occur and returns the key code of the pressed key.
Here is the general syntax of the waitKey()
function:
key = cv2.waitKey(delay)
The delay
parameter specifies the amount of time, in milliseconds, to wait for a key event. A value of 0 indicates that the function should wait indefinitely until a key is pressed.
The waitKey()
function returns an integer value representing the key code of the pressed key. If no key is pressed within the specified time, it returns -1.
Basic Example
Here’s an example usage of waitKey()
in a simple OpenCV program:
import cv2
# Read an image image = cv2.imread('image.jpg') # Display the image cv2.imshow('Image', image) # Wait for a key event for 3000 milliseconds (3 seconds) key = cv2.waitKey(3000) # Check if a key was pressed if key == ord('q'): # Check if 'q' key was pressed print("You pressed the 'q' key.") # Close the OpenCV windows cv2.destroyAllWindows()
In the above example, the program displays an image and waits for 3 seconds for a key event. If the ‘q’ key is pressed within that time, it prints a message. The ord()
function is used to convert the character ‘q’ to its corresponding key code.
Note that it’s important to call cv2.destroyAllWindows()
to close any open OpenCV windows after you’re done processing and displaying your images.
Key Mapping
We can easily find a keys corresponding key code with a simple Python script:
import cv2 print('Starting Key Test') camera=cv2.VideoCapture(0) while(1): ret,frame=camera.read() cv2.imshow('Camera',frame) k = cv2.waitKey(33) if k==27: # Esc key to stop break elif k==-1: # normally -1 returned,so don't print it continue else: print (k) # else print its value
In the above example, when the code is run, the camera stream will open. While the camera stream is open, if any key on your keyboard is pressed, the corresponding key value will be printed to the terminal console.
Conclusion
Congratulations on mastering the orchestration of time in your OpenCV projects! With a deep understanding of the waitKey()
function, you’ve gained the ability to synchronize user interactions, control frame rates, and shape the temporal flow of your computer vision applications. As you conclude this guide, envision the interactive possibilities that await—where each keypress unfolds new dimensions in visual computation. Your journey with OpenCV has just entered a realm of dynamic engagement. What innovative projects will you create next?
Finding a keys corresponding key value will be very useful for the upcoming OpenCV guides, where we need to know the key values to have greater control of our project.
In our next OpenCV for Beginners guide we will be learning how to Add Shapes and Text to Frames.
That’s All Folks!
You can find all of our OpenCV guides here: OpenCV for Beginners