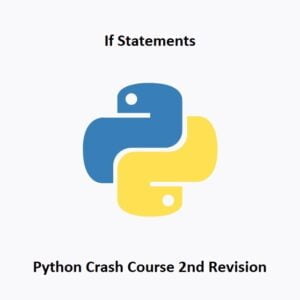
Making Decisions in Your Code
Conditional statements are essential tools in programming, allowing you to create dynamic and responsive code that makes decisions based on certain conditions. In Python, you can use if
, elif
, and else
to control the flow of your program. In this part of our Python crash course, we’ll explore how conditional statements work and how to use them effectively.
The if
Statement
The if
statement is the most basic form of a conditional statement. It checks whether a given condition is True
and, if so, executes a block of code. Here’s the syntax:
if condition: # Code to execute if the condition is True
Example: Using an if
Statement
age = 18 if age >= 18: print("You are an adult.")
In this example, the code inside the if
block is executed because the condition (age >= 18
) is true.
The elif
Statement
Sometimes, you need to check multiple conditions in a specific order. This is where the elif
(short for “else if”) statement comes into play. It allows you to evaluate multiple conditions until one of them is true. Here’s the syntax:
if condition1: # Code to execute if condition1 is True elif condition2: # Code to execute if condition2 is True
Example: Using an elif
Statement
score = 85 if score >= 90: print("A") elif score >= 80: print("B")
In this example, the code checks both conditions, but only the first one that’s true is executed.
The else
Statement
The else
statement is used to provide a default action when none of the conditions in the if
or elif
statements is true. Here’s the syntax:
if condition: # Code to execute if the condition is True else: # Code to execute if the condition is False
Example: Using an else
Statement
num = 5 if num % 2 == 0: print("Even") else: print("Odd")
In this example, the code checks if num
is even or odd and provides an appropriate message based on the result.
Combining Conditional Statements
You can also combine multiple conditional statements to create complex decision structures. Logical operators like and
, or
, and not
help you build intricate conditions.
Example: Combining Conditional Statements
age = 25 income = 45000 if age >= 18: if income >= 30000: print("You qualify for a premium loan.") else: print("You qualify for a standard loan.") elif age < 18: print("You are too young to apply for a loan.") else: print("You do not meet the age requirement for a loan.")
This code combines the conditions of age and income using the and
operator.
Conclusion
Conditional statements are the building blocks of decision-making in your Python programs. They enable you to create dynamic applications that respond to user input and changing data. In the next part of our Python crash course, we’ll dive into Python’s Data Structures, including lists, dictionaries, and more, to handle and manipulate data effectively. Stay tuned for more Python programming knowledge!
That’s All Folks!
You can explore more of our Python guides here: Python Guides