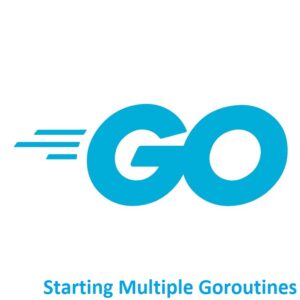
Initiating Multiple Goroutines in Golang!
Welcome to the world of Golang! Starting multiple goroutines is a cornerstone of concurrent programming in Golang, enabling programs to execute tasks concurrently, thereby improving efficiency and responsiveness.
Let’s discuss goroutines and worker pools:
Starting Multiple Goroutines
- In Go, a goroutine is a lightweight thread of execution that can run concurrently with other goroutines within the same program.
- You can start multiple goroutines by using the
go
keyword followed by a function or method call. This will execute the specified function concurrently in a new goroutine. - Starting multiple goroutines is a common way to achieve concurrency and parallelism in Go programs. Each goroutine can perform a specific task, and they can communicate with each other using channels or other synchronization mechanisms.
Here’s an example of starting multiple goroutines:
package main import ( "fmt" "time" ) func worker(id int) { for i := 0; i < 5; i++ { fmt.Printf("Worker %d: Working on task %d\n", id, i) time.Sleep(time.Second) } } func main() { for i := 0; i < 3; i++ { go worker(i) } // Ensure the main goroutine doesn't exit before other goroutines finish. time.Sleep(5 * time.Second) }
In this example, we start three goroutines representing worker tasks concurrently.
Worker Pools
- A worker pool is a design pattern used for managing and reusing a fixed number of worker goroutines to process tasks or jobs.
- Instead of starting a new goroutine for each task, you create a pool of goroutines at the beginning of your program and feed tasks to these workers from a queue or channel.
- Worker pools are beneficial when you want to limit the concurrency level or manage resources efficiently. For example, in scenarios where you want to control how many tasks are processed concurrently, such as in web servers or concurrent data processing pipelines.
Here’s an example of a simple worker pool:
package main import ( "fmt" "sync" ) func worker(id int, jobs <-chan int, results chan<- int) { for job := range jobs { fmt.Printf("Worker %d: Processing job %d\n", id, job) results <- job * 2 // Simulated work } } func main() { numWorkers := 3 numJobs := 10 jobs := make(chan int, numJobs) results := make(chan int, numJobs) var wg sync.WaitGroup // Create and start worker goroutines for i := 1; i <= numWorkers; i++ { wg.Add(1) go func(workerID int) { defer wg.Done() worker(workerID, jobs, results) }(i) } // Feed jobs to the worker pool for i := 1; i <= numJobs; i++ { jobs <- i } close(jobs) // Wait for all workers to finish wg.Wait() // Close the results channel after all jobs are done close(results) // Collect and print results for result := range results { fmt.Printf("Received result: %d\n", result) } }
In this example, we create a worker pool with three worker goroutines that process jobs concurrently.
Conclusion
So, while both starting multiple goroutines and using a worker pool involve goroutines, they serve different purposes and are used in different scenarios. Starting multiple goroutines is more suitable when you want to start many tasks concurrently without limiting their number, while a worker pool is suitable for managing a fixed number of workers to process tasks efficiently.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang