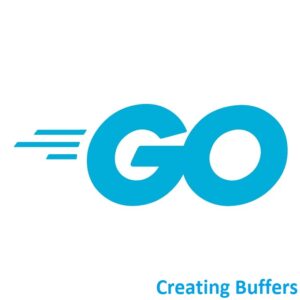
Unlocking Performance with Efficient Buffer Management
Welcome to the world of Golang! Buffers play a vital role in optimizing I/O operations and memory management. In this guide, we’ll explore buffer creation techniques in Go, understanding how to efficiently allocate memory buffers, enhancing performance, and reducing overhead in I/O-intensive operations.
Explaining Buffers
Buffers:
Buffers serve as temporary storage areas in programming. They hold data while it’s being transferred from one place to another. In Go, buffers are crucial for optimizing I/O operations by reducing the overhead associated with frequent read and write system calls. They act as an intermediary, allowing data to be collected and processed in larger, more efficient chunks.
Buffer Creation Methods:
- Using
bytes
Package: Go’sbytes.Buffer
is a powerful structure that efficiently manages byte slices. It provides methods for appending, reading, and manipulating byte data. To create a buffer with thebytes
package, you typically instantiate abytes.Buffer
usingbytes.NewBuffer()
orbytes.Buffer{}
and then use its methods to handle data. - Custom Buffer Allocation: Custom buffer allocation involves initializing arrays or slices as buffers. For instance, creating a slice with a predefined capacity and using it to handle data can be an efficient method. This approach allows for more control over buffer size and data management.
Optimizing I/O Operations:
- File I/O: When dealing with file operations, using buffers significantly reduces the number of read or write system calls by aggregating data. Reading or writing data in larger, buffered chunks enhances performance.
- Network Operations: Buffers are instrumental in managing data from network connections. They collect smaller chunks of data before transmission, reducing the number of network calls and improving efficiency.
- Stream Processing: In scenarios involving streams of continuous data, buffers aid in handling and processing incoming data, allowing for optimized parsing or decoding operations.
Memory Efficiency:
- Buffer Management and Reuse: Proper buffer management involves reusing buffers to avoid unnecessary allocations and deallocations. Reusing existing buffers helps minimize memory overhead.
- Optimizing Buffer Size: Choosing an appropriate buffer size based on the amount of data being processed and the available memory is crucial. Oversized buffers can lead to wastage, while undersized buffers might cause frequent reallocations.
- Reducing Allocation Overhead: Efficient buffer usage reduces the strain on memory allocation mechanisms, leading to better memory management and lower allocation overhead.
Understanding these aspects of creating buffers in Go will enable you to efficiently manage data, optimize I/O operations, and handle memory more effectively in your Go programs.
Creating Buffers
In Go, you can create buffers using slices. Slices are a built-in data structure in Go that provide a flexible way to work with sequences of elements, such as arrays or buffers. Here’s how you can create and work with buffers using slices in Go:
Creating an Empty Buffer:
You can create an empty buffer (slice) of a specific type using the make
function or by using a slice literal.
Here’s an example using make
:
// Create an empty byte buffer with a capacity of 10 buffer := make([]byte, 0, 10)
In the above example, make([]byte, 0, 10)
creates an empty byte slice (buffer) with an initial length of 0 and a capacity of 10. The capacity determines how much data the buffer can hold before it needs to be resized.
Appending Data to a Buffer:
To add data to the buffer, you can use the append
function.
For example:
// Append data to the buffer buffer = append(buffer, 'H', 'e', 'l', 'l', 'o')
In this case, we are appending individual bytes to the buffer. You can also append slices or other buffers to combine data.
Reading and Manipulating Data:
You can read and manipulate data in the buffer just like you would with any other slice.
For example:
// Reading data from the buffer firstByte := buffer[0] // Accessing the first byte in the buffer // Modifying data in the buffer buffer[1] = 'E' // Changing the second byte to 'E'
Resizing the Buffer:
If the buffer reaches its capacity and you need to add more data, Go will automatically resize the buffer to accommodate the new data. You don’t need to worry about managing the buffer’s size manually. However, resizing operations can be relatively expensive, so it’s a good practice to pre-allocate a reasonable capacity if you know the expected size of your buffer in advance.
Clearing the Buffer:
If you want to reset the buffer and make it empty again, you can do so by creating a new empty slice or by using the buffer = buffer[:0]
slice operation.
Golang Code Example
Here’s a simple example that puts it all together:
package main import "fmt" func main() { // Create an empty byte buffer with a capacity of 10 buffer := make([]byte, 0, 10) // Append data to the buffer buffer = append(buffer, 'H', 'e', 'l', 'l', 'o') // Read and manipulate data in the buffer fmt.Printf("Buffer: %s\n", buffer) // Output: Buffer: Hello // Clear the buffer buffer = buffer[:0] fmt.Printf("Buffer after clearing: %s\n", buffer) // Output: Buffer after clearing: }
This is a basic example of working with buffers in Go. Buffers are often used for I/O operations, like reading from or writing to files or network connections. Depending on your use case, you may want to use different types, such as bytes.Buffer
, which provides more advanced functionality for working with byte buffers.
Conclusion
Congratulations on mastering buffer creation in Go! You’ve gained insights into optimizing I/O operations and memory efficiency using buffers. As you continue your journey in Go programming, leverage buffer creation techniques to enhance performance, improve I/O operations, and efficiently manage memory.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang