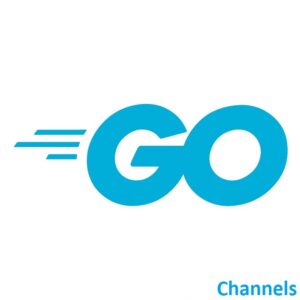
Synchronized Communication Between Goroutines
Welcome to the world of concurrent programming in Go! Channels play a critical role in synchronizing communication between goroutines, enabling efficient concurrency. In this comprehensive guide, we’ll explore channels in Golang, understanding their functionality and significance in orchestrating synchronized communication. By the end, you’ll be adept at utilizing channels for synchronized communication between concurrent goroutines in Go.
What are Channels?
In Go, channels are a fundamental concept for concurrent programming. They provide a way for goroutines (lightweight threads in Go) to communicate and synchronize their execution. Channels are used to send and receive data between goroutines and can help coordinate concurrent operations.
Here are some key points about Go channels:
Channel Creation:
You can create a channel using the make
function with the chan
keyword followed by the data type of the values you want to send through the channel.
For example:
ch := make(chan int) // Create an integer channel
Sending Data to a Channel:
You can send data into a channel using the <-
operator.
For example:
ch <- 42 // Send the value 42 into the channel
Receiving Data from a Channel:
You can receive data from a channel using the <-
operator on the left side of an assignment.
For example:
value := <-ch // Receive a value from the channel and store it in the 'value' variable
Blocking:
Sending to a channel will block until there is a receiver ready to receive the data, and receiving from a channel will block until there is data available to be received. This blocking behavior helps synchronize goroutines.
Closing Channels:
You can close a channel using the close
function. Once a channel is closed, you can’t send data into it anymore, but you can still receive any remaining data in the channel. Attempting to send on a closed channel will panic, and receiving from a closed channel will return the zero value for the channel’s type.
close(ch)
Channel Direction:
You can specify the direction of a channel when defining function parameters to restrict whether a function can send, receive, or both on a channel.
For example:
func sendOnly(ch chan<- int) { // This function can only send data to the channel } func receiveOnly(ch <-chan int) { // This function can only receive data from the channel }
Buffered Channels:
Channels can be buffered, meaning they can hold a certain number of values before blocking. You can specify the buffer size when creating a channel:
ch := make(chan int, 3) // Create a buffered channel with a capacity of 3
Select Statement: The select
statement allows you to choose which channel to send or receive from when multiple channels are involved in your program. It’s like a switch
statement for channels.
select { case value := <-ch1: // Handle data from ch1 case ch2 <- 42: // Send data to ch2 }
Golang Code
Here’s a simple example of using Go channels to calculate the sum of numbers concurrently:
package main import ( "fmt" "sync" ) func main() { // Create a channel to send and receive integers ch := make(chan int) // Create a WaitGroup to wait for all goroutines to finish var wg sync.WaitGroup // Number of goroutines to create numGoroutines := 4 // Start goroutines to generate numbers and send them to the channel for i := 0; i < numGoroutines; i++ { wg.Add(1) go generateNumbers(i, ch, &wg) } // Start a goroutine to receive and sum the numbers go sumNumbers(ch, &wg) // Wait for all goroutines to finish wg.Wait() // Close the channel to signal that no more data will be sent close(ch) } func generateNumbers(id int, ch chan int, wg *sync.WaitGroup) { defer wg.Done() for i := 1; i <= 5; i++ { ch <- i fmt.Printf("Generator %d sent: %d\n", id, i) } } func sumNumbers(ch chan int, wg *sync.WaitGroup) { defer wg.Done() sum := 0 for num := range ch { sum += num } fmt.Printf("Sum of numbers: %d\n", sum) }
In this example:
- We create an integer channel
ch
for communication. - We start four goroutines (
generateNumbers
) that generate numbers and send them to the channel. Each generator sends five numbers. - We start another goroutine (
sumNumbers
) to receive the numbers from the channel and calculate their sum. - We use a
sync.WaitGroup
to wait for all goroutines to finish. - Finally, we close the channel to signal that no more data will be sent.
When you run this program, you’ll see output indicating that numbers are being generated and sent to the channel, and then the sum of all the generated numbers will be printed at the end. The use of channels ensures that the generators and the summing goroutine work concurrently and safely.
Conclusion
Congratulations on mastering channels in Go! You’ve gained essential knowledge in orchestrating synchronized communication between goroutines, fostering efficient concurrent programming. Go channels are a powerful tool for managing concurrency and communication between goroutines, making it easier to write concurrent and parallel programs. They are a core feature of the Go language, and mastering their usage is essential for effective Go programming. As you continue your Go programming journey, practice utilizing channels for coordinating concurrent tasks, unlocking the full potential of concurrent programming in Go.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang