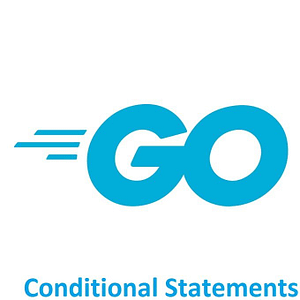
Understanding Conditional Statements in Golang
Conditional statements allow programs to make decisions and execute specific code blocks based on conditions. In this guide, we’ll delve into the world of conditional statements in Golang, empowering you to dynamically control program execution based on different scenarios.
Different Types of Conditional Statements
In Go, conditional statements are used to make decisions and execute different blocks of code based on certain conditions. Go provides the standard if
, else if
, and else
statements for handling conditionals. Here’s how they work:
if
statement:
The basic if
statement is used to execute a block of code if a given condition is true. It has the following syntax:
if condition { // code to execute if condition is true }
Here is a complete example:
package main import "fmt" func main() { x := 10 if x > 5 { fmt.Println("x is greater than 5") } }
if
…else
statement:
You can use the else
clause to execute a different block of code when the condition in the if
statement is false. The syntax is as follows:
if condition { // code to execute if condition is true } else { // code to execute if condition is false }
Here is a complete example:
package main import "fmt" func main() { x := 3 if x > 5 { fmt.Println("x is greater than 5") } else { fmt.Println("x is not greater than 5") } }
if
…else if
…else
statement:
You can use multiple else if
clauses to check for additional conditions. The syntax is:
if condition1 { // code to execute if condition1 is true } else if condition2 { // code to execute if condition2 is true } else { // code to execute if no conditions are true }
Here is a complete example:
package main import "fmt" func main() { x := 7 if x > 10 { fmt.Println("x is greater than 10") } else if x > 5 { fmt.Println("x is greater than 5 but not greater than 10") } else { fmt.Println("x is not greater than 5") } }
These are the basic conditional statements in Go. They allow you to control the flow of your program based on various conditions. Remember that Go’s if
statements don’t require parentheses around the condition and don’t require explicit semicolons at the end of lines, making the syntax clean and simple.
Switch Statements:
We can employ switch
statements for multi-way branching based on different values.
switch expression { case value1: // Code block for value1 case value2: // Code block for value2 default: // Code block if no cases match }
Fallthrough in Switch:
Using fallthrough
to execute the next case in a switch
statement.
switch expression { case value1: // Code block for value1 fallthrough case value2: // Code block for value2 executed after value1 }
Nested Conditional Statements
You can control the flow of nested conditional statements using various control structures like if
, else if
, and else
. Here’s an example of how you can structure, and control nested conditional statements in Go:
package main import "fmt" func main() { age := 25 if age >= 18 { fmt.Println("You are an adult.") if age >= 21 { fmt.Println("You can legally drink.") } else { fmt.Println("You cannot legally drink yet.") } } else { fmt.Println("You are a minor.") } }
In this example, the code checks the value of the age
variable to determine if the person is an adult or a minor. If they are an adult, it then checks if they are old enough to legally drink.
Breaking Down the Code
The outer
if
statement checks ifage
is greater than or equal to 18. If true, it means the person is an adult, and the code enters this block.Inside the adult block, there’s another nested
if
statement. It checks ifage
is greater than or equal to 21. If true, it means the person is old enough to legally drink. If false, theelse
block is executed, indicating that the person cannot legally drink yet.If the outer
if
condition is false, meaning the person is not an adult (age < 18), the code enters theelse
block, which indicates that the person is a minor.
Remember that proper indentation is essential for maintaining the readability of your code, especially when dealing with nested conditional statements.
Conclusion
Conditional statements are fundamental for implementing decision-making logic in Golang. By mastering if
, else
, and switch
constructs, you gain the ability to create dynamic program flow, handle different scenarios, and execute specific code blocks based on varying conditions within your Golang programs.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang