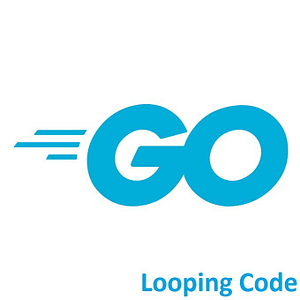
Controlling Program Flow with Iterative Structures
Loops are a crucial aspect of programming that allow executing repetitive tasks efficiently. In this guide, we’ll delve into the looping constructs available in Golang, empowering you to control program flow and perform iterative operations effectively.
Different Loop Constructs
In Go, you can use different types of loops to iterate over data or execute a block of code multiple times. The three main types of loops in Go are:
for loop:
The most common loop type in Go is the for
loop. It can be used in several ways:
Basic for
loop:
for i := 0; i < 5; i++ { // Code to be executed in each iteration }
for
loop as a while loop:
Unlike other coding languages, Golang does not have a separate “while loop” function. Instead, we can achieve the same result like this:
counter := 0 for counter < 5 { // Code to be executed in each iteration counter++ }
Infinite loop:
The for
loop below lacks a condition to terminate, so it will keep running indefinitely.
for { // Code to be executed in each iteration }
range loop:
This loop is used to iterate over elements in an array, slice, string, map, or channel. It automatically iterates over the items in the collection.
numbers := []int{1, 2, 3, 4, 5} for index, value := range numbers { // 'index' is the index of the current element // 'value' is the value of the current element }
Optimizing Loop Performance:
Loop Optimization Techniques:
Understand best practices and optimizing loops for efficiency.
- Reduce Function Calls: Minimize function calls inside loops. Instead of calling a function multiple times within a loop, consider calling it once and storing the result in a variable.
- Precompute Values: Compute values outside the loop that remain constant throughout the iterations.
- Avoid Unnecessary Work: Evaluate conditions or perform operations outside the loop if they don’t change within iterations.
- Use Specific Loop Constructs: Choose the appropriate loop construct (
for
,range
) based on the context and type of iteration to avoid unnecessary overhead. - Reduce Work Inside Loops: Minimize unnecessary work inside loops by moving complex computations or operations outside the loop.
- Benchmark and Profiling: Use Go’s built-in benchmarking and profiling tools (
go test -bench
andgo tool pprof
) to identify performance bottlenecks and optimize loops based on actual benchmarks.
Optimizing loops involves finding a balance between readability and performance. These techniques help improve efficiency by reducing unnecessary computations, function calls, and iterations within loops, resulting in more optimized code. Always prioritize readability and maintainability while considering optimizations.
Avoiding Infinite Loops:
Implementing safeguards and conditions to prevent unintended infinite loops.
- Using Conditional Statements: Always include a condition that allows the loop to exit. Ensure the loop’s condition eventually becomes false to prevent it from running indefinitely.
- Defining Exit Conditions: Ensure loop conditions are based on variables that change within the loop, guaranteeing eventual termination.
- Incorporating Break Statements: Use the
break
statement to exit the loop based on specific conditions. Ensurebreak
is used judiciously and not excessively. - Using Timeouts or Counters: Implement timeouts or counters to prevent the loop from running indefinitely, especially in scenarios involving network operations or user input.
- Testing and Validation: Thoroughly test loops during development to ensure they terminate as expected under various conditions. Use unit tests to validate loop behavior.
- Code Reviews: Conduct code reviews to identify potential issues with loops, especially regarding exit conditions and control flow.
Preventing unintended infinite loops involves enforcing clear exit conditions, testing loop behavior, and using break statements judiciously to ensure the loop executes as intended and terminates when required. Regular code reviews and testing are essential for identifying and rectifying potential issues related to infinite loops.
Code Example
Here’s an example that demonstrates these loop types:
package main import "fmt" func main() { // Example of a basic for loop for i := 0; i < 5; i++ { fmt.Println("Basic for loop:", i) } // Example of a for loop as a while loop counter := 0 for counter < 5 { fmt.Println("While loop:", counter) counter++ } // Example of a range loop numbers := []int{1, 2, 3, 4, 5} for index, value := range numbers { fmt.Printf("Index: %d, Value: %d\n", index, value) } }
Remember that in Go, unlike some other languages, you don’t have the traditional while
loop construct. Instead, you can achieve similar behavior using the for
loop constructs demonstrated above.
Conclusion
Loops are fundamental for executing repetitive tasks and controlling program flow in Golang. By mastering the for
, range
, and simulated while
constructs, you gain the ability to perform iterative operations efficiently and maintain better control over your programs.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang