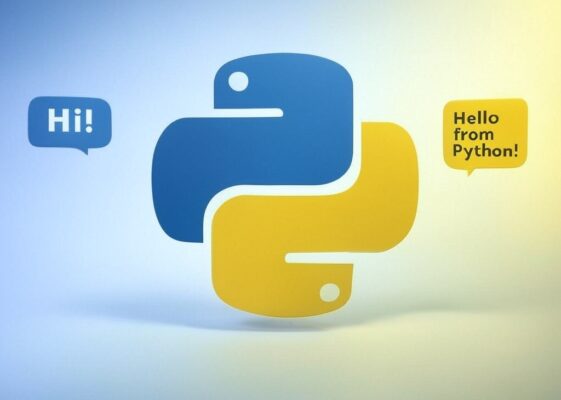
Really Simple Chatbot
Building a chatbot might sound complex, but with Python and just the random
library, you can create a simple, functional bot in no time! This quick guide will walk you through the process step-by-step, showing how easy it is to get started. Whether you’re a beginner or just looking for a fun project, this chatbot is a great way to dip your toes into Python programming.
In this guide, we’ll build a chatbot with three key components:
- A
greet()
function to start the conversation with a random greeting. - A
get_response()
function to reply to user input based on a dictionary of predefined responses. - A main loop to keep the conversation flowing until the user decides to exit.
By the end, you’ll have a working chatbot, and ideas for how to make it even better!
How the Chatbot Works
Step 1: The Greeting Function
The greet()
function is the chatbot’s friendly hello. It uses Python’s random.choice()
to pick a greeting from a list, making the bot feel a bit more dynamic. Here’s the code:
import random def greet(): greetings = ["Hello!", "Hi!", "Hey there!", "Greetings!"] return random.choice(greetings)
Try running this alone, you’ll get a different greeting each time!
Step 2: The Response Function
The get_response()
function is the brain of the bot. It takes what the user types and matches it to a response from a dictionary. If the input isn’t recognized, it gives a fallback reply. Here’s how it looks:
def get_response(user_input): responses = { "hello": "Hello! How can I assist you?", "how are you?": "I'm doing well, thank you!", "bye": "Goodbye! Have a great day!", "what’s your name?": "I’m Grok, your friendly chatbot!", "tell me a joke": "Why don’t skeletons fight each other? Because they don’t have the guts!" } # Convert input to lowercase to make it case-insensitive user_input = user_input.lower() # Check if the input matches a key in the dictionary if user_input in responses: return responses[user_input] else: return "I'm sorry, but I don’t understand. Could you rephrase or ask something else?"
I’ve added a few extra responses (e.g., a name and a joke) to make it more interesting. You can expand this dictionary with as many entries as you like, think of it as teaching your bot new tricks!
Step 3: The Conversation Loop
The main loop ties everything together. It starts with a greeting, then keeps chatting until the user types "exit". Here’s the full code:
import random def greet(): greetings = ["Hello!", "Hi!", "Hey there!", "Greetings!"] return random.choice(greetings) def get_response(user_input): responses = { "hello": "Hello! How can I assist you?", "how are you?": "I'm doing well, thank you!", "bye": "Goodbye! Have a great day!", "what’s your name?": "I’m Chit Chat, your friendly chatbot!", "tell me a joke": "Why don’t skeletons fight each other? Because they don’t have the guts!" } user_input = user_input.lower() if user_input in responses: return responses[user_input] else: return "I'm sorry, but I don’t understand. Could you rephrase or ask something else?" # Start the conversation print("AI Bot: " + greet()) while True: user_input = input("User: ") if user_input.lower() == "exit": print("AI Bot: Goodbye!") break bot_response = get_response(user_input) print("AI Bot:", bot_response)
When you run this, the bot greets you, waits for your input, and responds accordingly. Type "exit" to end the chat.
Making the Chatbot Your Own
This is just the beginning! Here are some ideas to level up your chatbot:
- Add More Responses: Expand the
responses
dictionary with fun or useful replies (e.g., "what’s the weather?" or "sing a song"). - Handle Variations: Use
if "hello" in user_input
to catch phrases like "hello there" instead of exact matches. - Add Randomness: Make some responses a list and use
random.choice()
to vary the replies (e.g., multiple jokes). - Save Conversations: Use a file to log the chat history for later review.
Try tweaking the code and see what you can create!
You’re a Chatbot Creator, What’s Next?
Great work, you’re now building Python chatbots! Next, try adding more features like those suggested above or explore libraries like nltk
for natural language processing. Want more? Visit our Python Guides. Also, share your coolest chatbot idea on Twitter/X. See you soon!