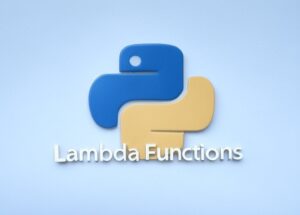
Understanding Python Lambda Functions
In Python, a lambda function, also known as an anonymous function or a lambda expression, is a small, anonymous function defined using the lambda
keyword. Lambda functions are typically used when you need a simple function for a short period of time, often as an argument to higher-order functions like map
, filter
, and sorted
. For simplicity, we’ll refer to them as lambda functions throughout this guide.
Basic Lambda Functions Syntax
The basic syntax of a lambda function is:
lambda arguments: expression
Here’s a breakdown of each part:
lambda
: This keyword is used to define a lambda function.arguments
: These are the input parameters or arguments that the lambda function takes. You can have zero or more arguments, separated by commas.expression
: This is a single expression that gets evaluated when the lambda function is called. The result of this expression is returned as the function’s output. Note that this must be an expression (likex + y
), not a statement (likeif x > 0:
orx = 5
).
Examples of Lambda Functions
Here are some examples to help you understand how to use lambda functions:
Simple Lambda Function
add = lambda x, y: x + y result = add(3, 5) print(result) # Output: 8
Using Lambda with Higher-Order Functions
Lambda functions are often used with functions like map
, filter
, and sorted
. For example:
- Using
map
:
numbers = [1, 2, 3, 4, 5] doubled = list(map(lambda x: x * 2, numbers)) print(doubled) # Output: [2, 4, 6, 8, 10]
filter
:numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) print(even_numbers) # Output: [2, 4, 6, 8, 10]
sorted
:names = ["Alice", "Bob", "Charlie", "David", "Eve"] sorted_names = sorted(names, key=lambda x: len(x)) print(sorted_names) # Output: ['Bob', 'Alice', 'David', 'Eve', 'Charlie']
Lambda Functions in Key Argument
Lambda functions are often used as the key
argument in sorting functions. They allow you to specify custom sorting criteria. For instance, you can sort a list of dictionaries based on a specific key:
people = [ {"name": "Alice", "age": 30}, {"name": "Bob", "age": 25}, {"name": "Charlie", "age": 35} ] sorted_people = sorted(people, key=lambda x: x["age"]) print(sorted_people) # Output: [{'name': 'Bob', 'age': 25}, {'name': 'Alice', 'age': 30}, {'name': 'Charlie', 'age': 35}]
When to Use Lambda vs. Regular Functions
Remember that while lambda functions are concise and useful for simple operations, they are limited in complexity compared to regular named functions defined with def
. For example, if you need a function with multiple lines or statements (like conditionals or loops), use def
instead:
def check_even(x): if x % 2 == 0: print(f"{x} is even") return x * 2
You’re a Lambda Pro, What’s Next?
Great work, you’re getting good at Python lambda functions! Next, try experimenting with more complex sorting or combining lambdas with other Python features. Want more? Check out our Python Guides. Also, share your favorite lambda trick on Twitter/X. See you soon!