Month: September 2023

Mastering Golang: Using Multiple Interfaces
Harnessing the Power of Multiple Interfaces! Welcome to the world of Golang! You can have a struct implement multiple interfaces…
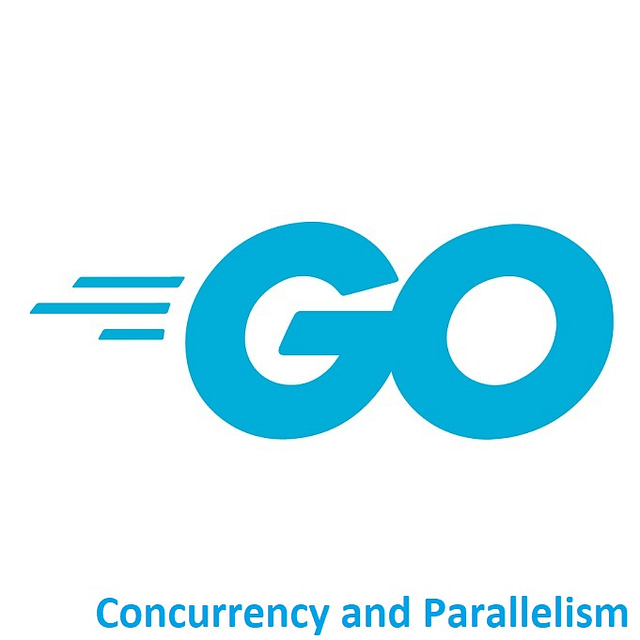
Mastering Golang: Concurrency and Parallelism
Concurrent Operations and Parallel Execution! Welcome to the world of Golang! Concurrency and parallelism are key features of Go that…
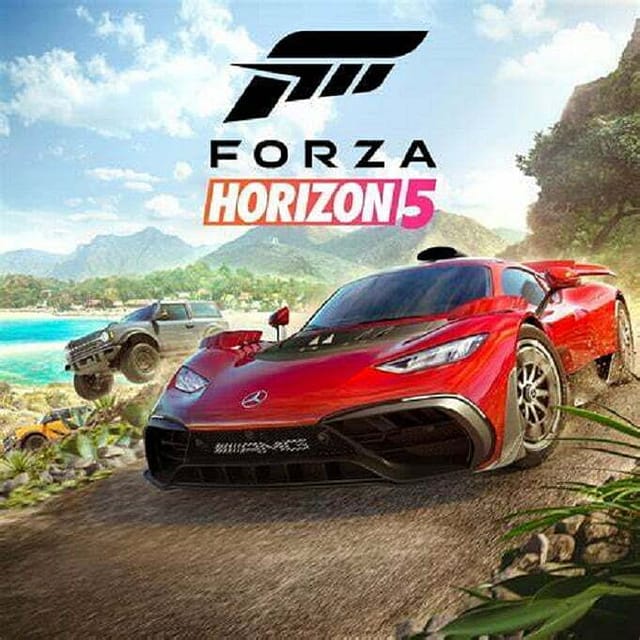
Random Race Generator for Forza Horizon 5
Optimizing Race Nights! Thursday nights is game night with my friends and me. We play Forza Horizon 5 and complete…
Mastering Golang: Interfaces Part 3
Leveraging Interfaces with Pointers and Receivers! Welcome to the world of Golang! Interfaces play a significant role in achieving polymorphism…
Mastering Golang: Interfaces Part 2
Advanced Interface Implementation and Usage! Welcome to the world of Golang! Interfaces are a fundamental concept that enable you to…
Mastering Golang: Understanding Timeouts
Utilizing Timeout Strategies for Reliable and Resilient Applications! Welcome to the world of Golang! Timeouts are a common mechanism used…
Mastering Golang: Basic Testing
Ensuring Code Quality with Effective Testing! Welcome to the world of Golang! Testing is an integral part of the language’s…
Mastering Golang: Inline Structures
Unnamed Structures for Streamlined Data Handling! Welcome to the world of Golang! Inline structs in Go enable the creation of…
Mastering Golang: Understanding Recursion
Understanding the Power and Limitations of Recursive Algorithms! Welcome to the world of Golang! Recursion is a powerful programming technique…
Mastering Golang: Structs as Function Arguments
Efficient Data Handling! Welcome to the world of Golang! Passing structures as function arguments in Go allows for streamlined data…
Mastering Golang: Iterating Data Sent to Channels
Maximizing Concurrency with Channel Data Iteration! Welcome to the world of Golang! You can use the range keyword to iterate…
Mastering Golang: Struct Field Exporting
Navigating Struct Field Visibility and Access Control! Welcome to the world of Golang! Struct fields can be exported or un-exported,…