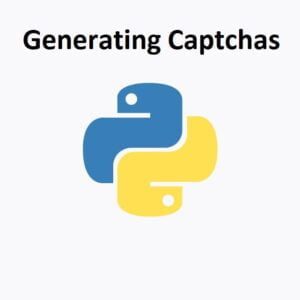
Enhancing Security with Custom Verification Tests
Welcome to the world of CAPTCHA generation in Python! CAPTCHAs are pivotal in distinguishing humans from automated bots. In this guide, we’ll explore CAPTCHA generation techniques in Python, learning how to create custom verification tests to secure web applications. By the end, you’ll be equipped to implement customized CAPTCHA solutions, enhancing the security of your web platforms.
Creating CAPTCHAs with Python
Generating CAPTCHAs (Completely Automated Public Turing test to tell Computers and Humans Apart) in Python can be accomplished using various libraries and techniques. CAPTCHAs are used to prevent automated bots from accessing certain web services or performing actions. Below, I’ll outline a basic example of how to generate a simple text-based CAPTCHA using Python.
There are several Python libraries that you can use to generate CAPTCHA images in your web applications or projects. CAPTCHAs are often used to prevent automated bots from accessing or submitting forms on websites.
Here are a few popular Python libraries for generating CAPTCHAs:
Captcha
The captcha
library provides a simple way to generate CAPTCHAs with random characters and background noise.
Installation
You can install it using pip:
pip install captcha
Python Code
Here’s a basic example of how you can use it:
from captcha.image import ImageCaptcha import random def generate_captcha(text): captcha = ImageCaptcha() image = captcha.generate_image(text) image.save("captcha.png") image.show() text = ''.join(random.choice('0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ') for _ in range(6)) generate_captcha(text)
Pillow
Pillow is a powerful Python Imaging Library that allows you to create and manipulate images, making it suitable for generating CAPTCHA images. You can use its drawing functions to create text-based CAPTCHAs on a blank image canvas.
Installation
You can install it using pip:
pip install Pillow
Python Code
Here’s an example of generating a simple text-based CAPTCHA:
from PIL import Image, ImageDraw, ImageFont import random import string # Define CAPTCHA parameters width, height = 200, 100 font_size = 36 captcha_length = 6 # Create a blank image image = Image.new('RGB', (width, height), color = (255, 255, 255)) draw = ImageDraw.Draw(image) # Define a font font = ImageFont.truetype("arial.ttf", font_size) # Generate a random CAPTCHA string captcha_string = ''.join(random.choices(string.ascii_uppercase + string.digits, k=captcha_length)) # Draw the text on the image text_color = (0, 0, 0) draw.text((20, 30), captcha_string, fill=text_color, font=font) # Add some noise (optional) for _ in range(200): x = random.randint(0, width - 1) y = random.randint(0, height - 1) draw.point((x, y), fill=(0, 0, 0)) # Save or display the CAPTCHA image image.save("captcha.png") image.show()
In this code:
- We create a blank image using Pillow.
- We define a font and generate a random CAPTCHA string composed of uppercase letters and digits.
- We draw the CAPTCHA text on the image.
- Optionally, we add some noise by randomly placing black pixels on the image.
- Finally, we save the CAPTCHA image and display it.
You can customize the CAPTCHA generation by changing the parameters like font_size
, captcha_length
, and the font file. Remember to adjust the image dimensions accordingly if you change the font size or text length. Also, consider making the CAPTCHA more challenging by adding distortion or using more complex fonts if you want to increase security.
Flask-SeaSurf
If you’re building a web application with Flask, you can use Flask-SeaSurf, a Flask extension for CAPTCHA generation and CSRF protection. It combines CAPTCHA generation with CSRF tokens, making it a good choice for form submissions.
Installation
You can install it using pip:
pip install Flask-SeaSurf
Python Code
from flask import Flask, request from flask_seasurf import SeaSurf app = Flask(__name__) csrf = SeaSurf(app) @app.route("/your_form", methods=["GET", "POST"]) @csrf.csrf def your_form(): if request.method == "POST": # Verify the CAPTCHA and process the form data if csrf.verify(): # CAPTCHA is valid # Process the form data else: # CAPTCHA is invalid # Handle the error return render_template("your_form_template.html")
Conclusion
Congratulations on learning the basics of CAPTCHA generation in Python! You’ve acquired the knowledge to develop custom CAPTCHA tests, fortifying web applications against automated threats. These are just a few options for generating CAPTCHAs in Python. You can choose the one that best fits your project’s requirements and integrate it into your application accordingly. As you continue developing web solutions, implement custom CAPTCHAs tailored to your applications, ensuring heightened security against bots and malicious activities.
That’s All Folks!
You can explore more of our Python guides here: Python Guides
I do agree with all the ideas you’ve presented in your post. They are really convincing and will certainly work. Still, the posts are too short for beginners. Could you please extend them a bit from next time? Thanks for the post.