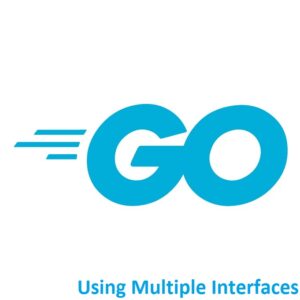
Harnessing the Power of Multiple Interfaces!
Welcome to the world of Golang! You can have a struct implement multiple interfaces by simply listing the interfaces in the structs declaration. This is a powerful feature that allows you to create versatile and reusable code.
Understanding Interface Composition in Go
Combining Multiple Interfaces
- In Go, an interface can include other interfaces as part of its definition, effectively merging their method sets.
Syntax of Interface Composition
- Go allows for interface composition using a simple syntax where one interface is embedded within another interface definition.
- The embedded interface’s methods become part of the larger interface, providing a unified set of methods.
Creating a Composite Interface
- By embedding multiple interfaces within a new interface definition, a composite interface is formed, inheriting all the methods from the embedded interfaces.
Advantages of Interface Composition
- Modularity: It facilitates the creation of modular and reusable code by allowing the aggregation of behavior from different interfaces into a single interface.
- Flexibility: Developers can compose interfaces to meet specific requirements, tailoring the functionalities needed for a particular context.
- Readability and Clarity: Interface composition enhances code readability by clearly defining and grouping related methods into a single entity.
Interface Embedding
- Interface embedding involves embedding one interface within another interface, inheriting its methods and behaviors.
- The embedded interface’s methods are accessible through the embedding interface, promoting code reuse and abstraction.
Composition Over Inheritance
- Interface composition follows the principle of “composition over inheritance,” allowing for more flexible and maintainable code by assembling functionalities through interfaces rather than relying solely on inheritance.
Example of Interface Composition
type Reader interface { Read() byte } type Writer interface { Write(byte) error } type ReadWriter interface { Reader Writer }
Here, ReadWriter
is a composite interface that combines Reader
and Writer
interfaces into a single interface containing both Read()
and Write()
methods.
Use Cases
- Interface composition is useful for creating higher-level interfaces from smaller, more specialized interfaces, allowing for better code organization and reuse in various scenarios.
Understanding interface composition in Go enables developers to create flexible, modular, and expressive interfaces by combining the behaviors of multiple smaller interfaces, leading to more manageable and maintainable codebases.
Implementing Multiple Interfaces in Go
Structs Implementing Interfaces
- In Go, a struct can implement one or more interfaces by defining all the methods required by each interface.
Interface Method Requirements
- To satisfy an interface, a struct needs to implement all the methods declared within that interface. If a struct implements all the methods specified in an interface, it automatically satisfies that interface.
Implementing Multiple Interfaces
- When a struct implements multiple interfaces, it must provide implementations for all the methods defined by each interface.
- By fulfilling the method requirements of multiple interfaces, the struct can be used wherever any of those interfaces are expected.
Example of Implementing Multiple Interfaces
type Reader interface { Read() byte } type Writer interface { Write(byte) error } type ReadWriter struct { // Fields, if any } func (rw *ReadWriter) Read() byte { // Implementation of Read method } func (rw *ReadWriter) Write(b byte) error { // Implementation of Write method }
In this example, ReadWriter
struct implements both Reader
and Writer
interfaces by providing implementations for Read()
and Write()
methods.
Implicit Interface Satisfaction
- Go uses implicit interface satisfaction. If a struct implements all the methods declared in an interface, it automatically satisfies that interface without explicitly stating it.
Flexibility and Usage
- By implementing multiple interfaces, a struct gains the flexibility to be used in contexts where any of those interfaces are expected.
- This approach allows for more adaptable code that can seamlessly work with various interface-based functionalities.
Benefits of Implementing Multiple Interfaces
- Reusability: A single struct implementing multiple interfaces leads to reusable code, as it can cater to diverse functionality requirements.
- Adaptability: It provides adaptability to different scenarios or systems that expect specific interfaces, offering a more general-purpose solution.
Implementing multiple interfaces in Go enables a struct to provide functionality required by various interface contracts simultaneously. This capability enhances code reusability, flexibility, and adaptability, allowing structs to be utilized in diverse contexts requiring different sets of behaviors.
Golang Code Example
Here’s a complete example of a struct that implements multiple interfaces:
package main import "fmt" // Define two interfaces type Reader interface { Read() string } type Writer interface { Write(string) } // Define a struct that implements both interfaces type ReadWriter struct { data string } // Implement the Read method for Reader interface func (rw *ReadWriter) Read() string { return rw.data } // Implement the Write method for Writer interface func (rw *ReadWriter) Write(s string) { rw.data = s } func main() { // Create an instance of ReadWriter rw := &ReadWriter{} // Use it as a Reader rw.Write("Hello, World!") fmt.Println(rw.Read()) // Output: Hello, World! }
In the above example, we have defined two interfaces, Reader
and Writer
, each with a single method. Then, we have a struct ReadWriter
that implements both Reader
and Writer
interfaces by providing implementations for the Read
and Write
methods.
Conclusion
Creating instances of the ReadWriter
struct and using them as both Reader
and Writer
objects, demonstrating how you can implement and use multiple interfaces. This is a fundamental feature of Go that allows you to write clean and modular code.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang
I love how you’ve broken down this topic into easy-to-understand sections. The step-by-step approach you’ve taken is very helpful.