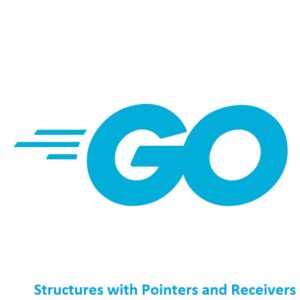
Leveraging Efficient Data Modeling and Method Attachments
Structures, pointers, and receiver functions are core concepts in Golang that enable efficient data modeling and method attachment. This guide elucidates their synergy, showcasing how pointers within structs and receiver functions enhance data handling and method capabilities.
Structs
In Go, pointers and receivers play a crucial role when working with structures (also known as structs). Understanding how pointers and receivers work with structs is essential for effective Go programming.
Let’s explore how they are used:
Structures (Structs):
A struct is a composite data type that groups together variables with different data types under a single name. Fields within a struct can be of any data type, including other structs.
Here’s an example of a simple struct definition:
type Person struct { FirstName string LastName string Age int }
Pointers to Structs:
In Go, you can create pointers to structs just like you can create pointers to other data types. Pointers are useful when you want to modify the original struct within a function or when you want to avoid copying the entire struct when passing it as an argument.
// Creating a pointer to a struct personPtr := &Person{"John", "Doe", 30} // Accessing fields through a pointer fmt.Println(personPtr.FirstName)
Receivers (Methods):
Receivers are special functions associated with a type, including structs. You can attach methods to a struct by defining a receiver function.
- Value Receivers: A receiver that takes a struct by value creates a copy of the struct. Changes made inside the method do not affect the original struct.
func (p Person) ChangeFirstName(newName string) { p.FirstName = newName // This won't modify the original struct }
Pointer Receivers:
A receiver that takes a pointer to a struct can modify the original struct. It operates on the original instance rather than a copy.
func (p *Person) ChangeLastName(newName string) { p.LastName = newName // This will modify the original struct }
Using Methods:
Once you’ve defined receiver functions, you can call them using an instance of the struct. If the method has a value receiver, it operates on a copy of the struct, while a pointer receiver operates on the original struct.
person := Person{"Alice", "Smith", 25} // Calling value receiver method (won't modify the original struct) person.ChangeFirstName("Bob") // Calling pointer receiver method (will modify the original struct) personPtr := &person personPtr.ChangeLastName("Doe")
Conclusion
Structures with pointers and receiver functions provide a powerful paradigm in Go, offering efficient data modeling and method attachment capabilities. By understanding and leveraging these concepts, developers can design more robust and functional Go programs with enhanced data handling and method functionalities.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang