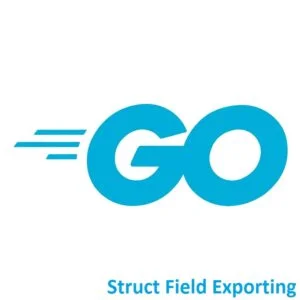
Navigating Struct Field Visibility and Access Control!
Welcome to the world of Golang! Struct fields can be exported or un-exported, influencing their visibility and accessibility within and outside the package. This guide delves into the concept of exported and un-exported fields, emphasizing the importance of encapsulation and access control for struct fields in Go programs. The visibility of a struct field (or any identifier) is determined by whether its name starts with an uppercase or lowercase letter.
Here’s how it works:
Uppercase first letter:
If a struct field’s name starts with an uppercase letter, it is exported, meaning it can be accessed from outside the package in which it is defined.
Lowercase first letter:
If a struct field’s name starts with a lowercase letter, it is unexported, meaning it can only be accessed within the same package where it is defined.
Here’s an example to illustrate this:
package mypackage // Person is a struct with exported and unexported fields type Person struct { // Exported field - can be accessed from outside the package FirstName string // Unexported field - can only be accessed within the package lastName string } // NewPerson creates a new Person instance func NewPerson(firstName, lastName string) Person { return Person{ FirstName: firstName, lastName: lastName, } }
In the example above, FirstName
is an exported field and can be accessed from outside the mypackage
package, whereas lastName
is an unexported field and can only be accessed within the same mypackage
package.
Golang Code Example
Here’s how you might use this struct:
package main import ( "fmt" "mypackage" ) func main() { person := mypackage.NewPerson("John", "Doe") // Accessing the exported field FirstName is allowed fmt.Println(person.FirstName) // Attempting to access the unexported field lastName will result in a compile-time error // fmt.Println(person.lastName) // This line will not compile }
Attempting to access the lastName
field from outside the mypackage
package will result in a compile-time error because it is unexported.
Conclusion
Understanding struct field exporting and visibility is essential for designing well-encapsulated and maintainable Go code. Proper use of exported and unexported fields ensures data integrity and controlled access within and across packages.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang