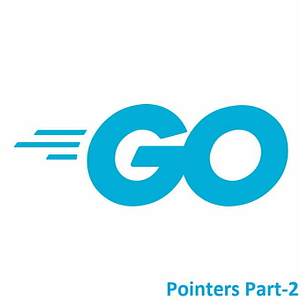
Understanding Advanced Pointer Operations
Welcome back to the world of Go pointers! Building on our previous exploration, this comprehensive guide delves deeper into the nuances of pointers in Golang. We’ll further explore memory addresses, pointer arithmetic, and advanced operations, empowering developers to utilize pointers for optimized memory management and efficient data handling in Go programs.
Why Pointers?
Go has pointers for several reasons, including:
Efficient Memory Management:
Pointers allow Go to efficiently manage memory by referencing the memory address of a value instead of creating copies of that value. This is particularly important for large data structures and when you want to modify data in place without incurring the overhead of copying.
Pass by Reference:
Go uses a “pass by value” approach, which means that when you pass an argument to a function, a copy of the argument is made. Pointers allow you to pass the memory address of a value instead, enabling functions to modify the original value. This is useful for avoiding unnecessary data copying and improving performance.
Data Sharing:
Pointers enable multiple parts of a program to share and manipulate the same data in memory. This is important for efficient communication and synchronization between concurrent Goroutines, which is a key feature of Go’s concurrency model.
Low-Level Memory Manipulation:
Go provides a degree of low-level memory manipulation through pointers, which can be useful in scenarios like working with C libraries or handling certain types of system-level tasks.
Data Structures:
Pointers are essential for building complex data structures like linked lists, trees, and graphs, where each element needs to reference other elements using pointers.
Despite the benefits, Go’s approach to pointers is designed to be safer and more straightforward than some other languages like C and C++. For example, Go does not support pointer arithmetic, which helps prevent common memory-related errors like buffer overflows and null pointer dereferences. Additionally, Go’s garbage collector simplifies memory management by automatically deallocating memory when it’s no longer needed, reducing the risk of memory leaks.
Diving Deeper into Pointers
Go pointers, can also work with hex values, and binary representations.
Let’s explore how you can connect these concepts in Go:
Pointers:
Pointers in Go are variables that store the memory address of another variable. You can use pointers to access or modify the value of the variable they point to.
Here’s an example of using pointers in Go:
package main import "fmt" func main() { var x int = 42 var ptr *int = &x // ptr is a pointer to the integer variable x fmt.Printf("Value of x: %d\n", x) fmt.Printf("Value of x through pointer: %d\n", *ptr) // Accessing the value through the pointer }
Hexadecimal Representation:
In Go, you can represent hexadecimal values using the 0x
prefix followed by the hexadecimal digits (0-9, A-F). You can also use the fmt
package to format and print hexadecimal values.
Here’s an example of hexadecimal representation in Go:
package main import "fmt" func main() { hexValue := 0x1A // Hexadecimal value fmt.Printf("Hexadecimal: 0x%X\n", hexValue) }
Binary Representation:
Go does not have a built-in binary literal representation like it does for hexadecimal (0x), but you can convert decimal or hexadecimal values to binary using the strconv
package and custom functions.
Here’s an example of converting a decimal value to binary:
package main import ( "fmt" "strconv" ) func decimalToBinary(decimal int) string { return strconv.FormatInt(int64(decimal), 2) } func main() { decimalValue := 42 binaryValue := decimalToBinary(decimalValue) fmt.Printf("Decimal: %d\n", decimalValue) fmt.Printf("Binary: %s\n", binaryValue) }
In the last example, we define a function decimalToBinary
that converts a decimal value to its binary representation using the strconv
package’s FormatInt
function with base 2.
You can combine these concepts as needed in your Go programs to work with pointers, hexadecimal values, and binary representations, depending on your requirements.
Conclusion
Congratulations on expanding your knowledge of pointers in Go! You’ve explored advanced pointer operations, enhancing your ability to manage memory efficiently. Go includes pointers to provide control over memory management, pass data efficiently, enable data sharing, support low-level operations, and facilitate the creation of complex data structures while maintaining safety and simplicity in the language. As you continue your Go programming journey, practice utilizing pointers for memory-sensitive tasks and complex data structures. With a deeper understanding of pointers, you’re better equipped to optimize memory usage and handle data more efficiently in Go.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang