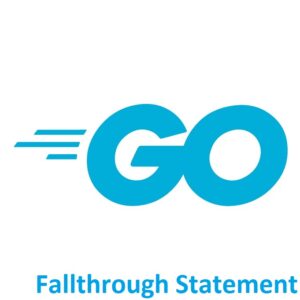
Exploring Go's Fallthrough for Enhanced Control Flow!
Welcome to the world of Golang! The fallthrough
statement in Go provides a unique mechanism for controlling the flow of execution within switch statements. Understanding how fallthrough
works and its implications is essential for precise control of program flow in Go programming. This guide delves into the functionalities and usage of fallthrough
, enabling you to harness this statement effectively in your code.
The fallthrough
statement is used within a switch
statement to transfer control to the next case block, regardless of whether the condition for that case is true or not. This behavior is different from most other programming languages, where a switch
statement typically exits after the first matching case is found.
Here’s a basic example to illustrate the usage of fallthrough
in Go:
package main import "fmt" func main() { num := 2 switch num { case 1: fmt.Println("Case 1") fallthrough case 2: fmt.Println("Case 2") fallthrough case 3: fmt.Println("Case 3") } }
In this example, if num
is set to 2
, the output will be:
Case 2 Case 3
Even though the condition for case 2
is true, the fallthrough
statement causes the execution to proceed to case 3
without checking its condition.
Important
Use fallthrough
statement judiciously, as it can sometimes lead to code that is less readable and more difficult to understand. In most cases, you’ll want to explicitly state each step in the switch
logic without relying on fallthrough
. Also, remember that a fallthrough
statement can only be used in a switch
statement; it’s not valid in other control structures like if
or loops.
Conclusion
The fallthrough
statement in Go offers a unique way to control the flow of execution within switch statements, enabling sequential execution of case blocks. Understanding its usage and limitations empowers Go developers to write more expressive and controlled code.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang