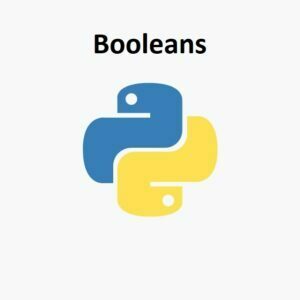
Crafting Logic in Python!
In this guide we will explain the intricacies of Boolean logic helping you to open doors for creating powerful, decision-driven code. Booleans lie at the heart of logical operations, serving as the building blocks for decision-making within programs. From foundational concepts to practical applications, this guide will give you the skills to harness the true potential of Booleans in Python programming.
The Basics to Boolean Logic
Booleans are a data type that represents two values: True
and False
. Booleans are often used in conditional statements and expressions to control the flow of a program and make decisions.
True and False
Python’s Boolean values are case-sensitive, so it’s important to use the capitalization True
and False
.
Boolean Operators
You can use Boolean operators to combine or manipulate values. The primary operators in Python are:
and
: ReturnsTrue
if both operands areTrue
, otherwise returnsFalse
.or
: ReturnsTrue
if at least one operand isTrue
, otherwise returnsFalse
.not
: Returns the opposite boolean value of the operand.
Basic Python Example:
x = True y = False print(x and y) # False print(x or y) # True print(not x) # False
Boolean Comparison Operators
You can also use comparison operators to create Boolean expressions. Common comparison operators include:
==
(equal to): ReturnsTrue
if the operands are equal.!=
(not equal to): ReturnsTrue
if the operands are not equal.<
(less than): ReturnsTrue
if the left operand is less than the right operand.>
(greater than): ReturnsTrue
if the left operand is greater than the right operand.<=
(less than or equal to): ReturnsTrue
if the left operand is less than or equal to the right operand.>=
(greater than or equal to): ReturnsTrue
if the left operand is greater than or equal to the right operand.
Python Example:
a = 5 b = 10 print(a == b) # False print(a < b) # True
Truthy and Falsey Values
In Python, some values are considered “truthy” and others “falsey” when used in Boolean contexts. For example, 0
and empty containers like []
and ''
are considered falsey, while non-zero numbers and non-empty containers are considered truthy. You can use these concepts in conditional statements.
Python Example:
x = 0 if x: print("x is truthy") else: print("x is falsy") # This will be printed
Type Casting
You can also use type casting functions like bool()
, int()
, float()
, etc., to convert values to booleans. In general, empty values and zero are converted to False
, while non-empty values and non-zero numbers are converted to True
.
Python Example:
value = 0 boolean_value = bool(value) print(boolean_value) # False
Conclusion
Booleans are fundamental in programming languages and are used extensively in decision-making processes and control structures like if
statements, while
loops, and more. As you continue your programming journey, let the understanding of Booleans be a guiding light in crafting robust and logical solutions.
That’s All Folks!
You can explore more of our Python guides here: Python Guides