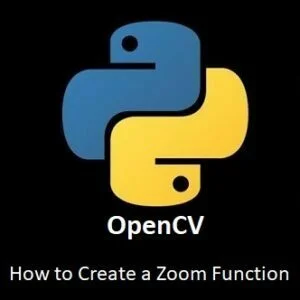
Zoom In, Zoom Out: Crafting a Zoom Function in OpenCV
Embark on the final leg of our dynamic vision journey as we unveil the power of zoom control using OpenCV with Python. In this guide, we’ll dive deep into crafting a custom zoom function to enhance your vision setup. With the ability to magnify and explore details, your projects are set to reach new heights of precision. Join us as we explore the intricacies of zoom control, providing you with the tools to dynamically adjust your view and bring your vision-based applications to life. Let’s zoom into the future of computer vision!
What’s Covered
- Zoom Images.
- Zoom Live Video.
- Manually Control the Zoom Strength.
- Final Project.
Zoom Images
Starting simple, we are going to use an image to zoom in and out on.
Open Visual Studio then copy and paste the code below to a new file.
In this example, the zoom_image
function takes an input image and a zoom_factor
. It calculates the new dimensions for the zoomed image, resizes the image using cv2.resize
, and then crops the resized image to the region of interest (centered on the original image). Finally, it returns the resulting zoomed image. You can adjust the zoom_factor
to control the level of zoom. A value greater than 1 will zoom in, while a value less than 1 will zoom out.
Python Code:
import cv2 def zoom_image(image, zoom_factor): height, width = image.shape[:2] new_height = int(height * zoom_factor) new_width = int(width * zoom_factor) # Resize the image resized_image = cv2.resize(image, (new_width, new_height)) # Calculate the region of interest (ROI) roi_x = int((new_width - width) / 2) roi_y = int((new_height - height) / 2) roi_width = width roi_height = height # Crop the image to the ROI zoomed_image = resized_image[roi_y:roi_y+roi_height, roi_x:roi_x+roi_width] return zoomed_image # Load the image image = cv2.imread('input.jpg') # Zoom in the image by a factor of 1.5 zoom_factor = 1.5 zoomed_image = zoom_image(image, zoom_factor) # Display the original and zoomed images cv2.imshow('Original Image', image) cv2.imshow('Zoomed Image', zoomed_image) cv2.waitKey(0) cv2.destroyAllWindows()
Zoom Live Video
To apply a zoom effect on live video using OpenCV, you can modify the previous code snippet to read frames from a video stream instead of a static image.
In this example, the zoom_frame
function is the same as before, but it now takes a frame from the video stream as input instead of an image. The video capture object is initialized using cv2.VideoCapture(0)
to read frames from the default camera. The while loop continuously reads frames from the video stream, applies the zoom effect using the zoom_frame
function, and displays the zoomed frame using cv2.imshow
. The loop breaks if the ‘q’ key is pressed.
Please note that the zoom factor and the camera index (0
in this case) can be adjusted to suit your specific requirements.
Open a new file in Visual Studio, then copy and paste the code below.
Python Code:
import cv2 def zoom_frame(frame, zoom_factor): height, width = frame.shape[:2] new_height = int(height * zoom_factor) new_width = int(width * zoom_factor) # Resize the frame resized_frame = cv2.resize(frame, (new_width, new_height)) # Calculate the region of interest (ROI) roi_x = int((new_width - width) / 2) roi_y = int((new_height - height) / 2) roi_width = width roi_height = height # Crop the frame to the ROI zoomed_frame = resized_frame[roi_y:roi_y+roi_height, roi_x:roi_x+roi_width] return zoomed_frame # Open a video capture object video_capture = cv2.VideoCapture(0) # Check if the video capture is opened successfully if not video_capture.isOpened(): raise Exception("Could not open video capture") # Initialize the zoom factor zoom_factor = 1.5 # Start reading and processing frames from the video stream while True: # Read a frame from the video stream ret, frame = video_capture.read() # Check if a frame was successfully read if not ret: break # Apply zoom to the frame zoomed_frame = zoom_frame(frame, zoom_factor) # Display the zoomed frame cv2.imshow('Zoomed Video', zoomed_frame) # Break the loop if 'q' is pressed if cv2.waitKey(1) & 0xFF == ord('q'): break # Release the video capture object and close any open windows video_capture.release() cv2.destroyAllWindows()
Manually Control the Zoom Strength
Now, with the code below I’m going to show you how you can control the “zoom” strength during live capture to zoom in and zoom back out again using your keyboard. In this example we are able to zoom in and out of the captured frames using the"+"
and "-"
keys on your keyboard.Python Code:
import cv2 # Initialize the zoom factor zoom_factor = .2 def zoom_frame(frame, zoom_factor): height, width = frame.shape[:2] new_height = int(height * zoom_factor) new_width = int(width * zoom_factor) # Resize the frame resized_frame = cv2.resize(frame, (new_width, new_height)) # Calculate the region of interest (ROI) roi_x = int((new_width - width) / 2) roi_y = int((new_height - height) / 2) roi_width = width roi_height = height # Crop the frame to the ROI zoomed_frame = resized_frame[roi_y:roi_y+roi_height, roi_x:roi_x+roi_width] return zoomed_frame # Open a video capture object camera = cv2.VideoCapture(0) cv2.namedWindow('Zoomed Video',cv2.WINDOW_NORMAL) while True: ret, frame=camera.read() # Apply zoom to the frame zoomed_frame=zoom_frame(frame, zoom_factor) # Display the zoomed frame cv2.imshow('Zoomed Video', zoomed_frame) cv2.resizeWindow('Zoomed Video',1920,1080) #Use OpenCV waitkey function for user controls k = cv2.waitKey(33) #Terminate program if k==27: #27 = ESC key break #Zoom Control if k==45: #down zoom_factor-=.05 if zoom_factor<=.1: zoom_factor=.1 print('Zoom ', zoom_factor) if k==61: #up zoom_factor+=.05 if zoom_factor>5: zoom_factor=5 print('Zoom ', zoom_factor) # Break the loop if 'q' is pressed if cv2.waitKey(1) & 0xFF == ord('q'): break # Release the video capture object and close any open windows camera.release() cv2.destroyAllWindows()
Final Project
Now, we are going to manually control the zoom with the"+"
and "-"
keys but also, we will include control of the cameras field-of-view, using the keyboards cursor keys.Being able to control the camera’s field-of-view and include zooming capabilities can be very useful in camera projects such as a security camera or a nature camera. You could easily add a “take snapshot” function to the #User Controls waitKey()
list of commands.Python Code:
import cv2 from adafruit_servokit import ServoKit #Set Servos pan=100 tilt=150 kit=ServoKit(channels=16) kit.servo[0].angle=pan kit.servo[1].angle=tilt # Initialize the zoom factor zoom_factor = .3 def zoom_frame(frame, zoom_factor): height, width = frame.shape[:2] new_height = int(height * zoom_factor) new_width = int(width * zoom_factor) # Resize the frame resized_frame = cv2.resize(frame, (new_width, new_height)) # Calculate the region of interest (ROI) roi_x = int((new_width - width) / 2) roi_y = int((new_height - height) / 2) roi_width = width roi_height = height # Crop the frame to the ROI zoomed_frame = resized_frame[roi_y:roi_y+roi_height, roi_x:roi_x+roi_width] return zoomed_frame #Set display resolution dispW=1920 dispH=1080 # Open a video capture object camera = cv2.VideoCapture(0) camera.set(cv2.CAP_PROP_FRAME_WIDTH,dispW) camera.set(cv2.CAP_PROP_FRAME_HEIGHT,dispH) cv2.namedWindow('Zoomed Video',cv2.WINDOW_NORMAL) while True: ret, frame = camera.read() #Position servos kit.servo[0].angle=pan kit.servo[1].angle=tilt # Apply zoom to the frame zoomed_frame = zoom_frame(frame, zoom_factor) # Display the zoomed frame cv2.imshow('Zoomed Video', zoomed_frame) #Set window size cv2.resizeWindow('Zoomed Video',1920,1080) #User Controls with OpenCV waitkey function k = cv2.waitKey(1) #Quit program using ESC key if k==27: #27 = ESC key break #Zoom Control if k==45: #45 is - key for zoom out zoom_factor-=.05 if zoom_factor<= .1: zoom_factor=.1 print('Zoom ', zoom_factor) if k==61: #61 is + key for zoom in zoom_factor+=.05 if zoom_factor> 5: zoom_factor=5 print('Zoom ',zoom_factor) #Servo Control if k==81: #81 = cursor left key pan+=5 print('Left',pan) if k==83: #83 = cursor right key pan=pan-5 print('Right',pan) if k==84: #84 = cursor down key tilt+=5 print('Down',tilt) if k==82: #82 = cursor up key tilt=tilt-5 print('Up',tilt) #Set pan tilt out of range corrections if pan>180: pan=180 print('pan out of range') if pan<0: pan=0 print('pan out of range') if tilt>180: tilt=180 print('tilt out of range') if tilt<0: tilt=0 print('tilt out of range') camera.release() cv2.destroyAllWindows() #Reset Servos kit.servo[0].angle=100 kit.servo[1].angle=150 print('Program Terminated')
Conclusion
Congratulations, visionary architect! You’ve reached the pinnacle of our dynamic vision guide by crafting a custom zoom function in OpenCV. With the ability to magnify and explore details, your vision setup is now equipped for a new level of precision and dynamic control.
As we conclude this guide, remember that your journey in computer vision is an ongoing exploration. Stay tuned for future advancements, challenges, and innovations in the dynamic field of vision. Your projects are now capable of so much exploration into the intricate details of the world.
That’s All Folks!
You can explore more of our Python guides here: Python Guides