Tag: Golang
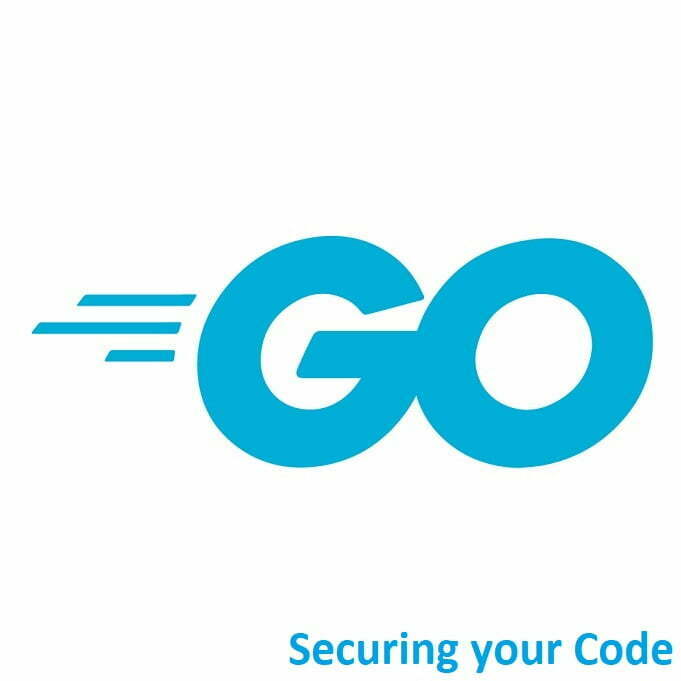
Mastering Golang: Securing Your Code
Best Practices for Robust Software Security! Welcome to the world of Golang! Securing your code is essential to protect your…
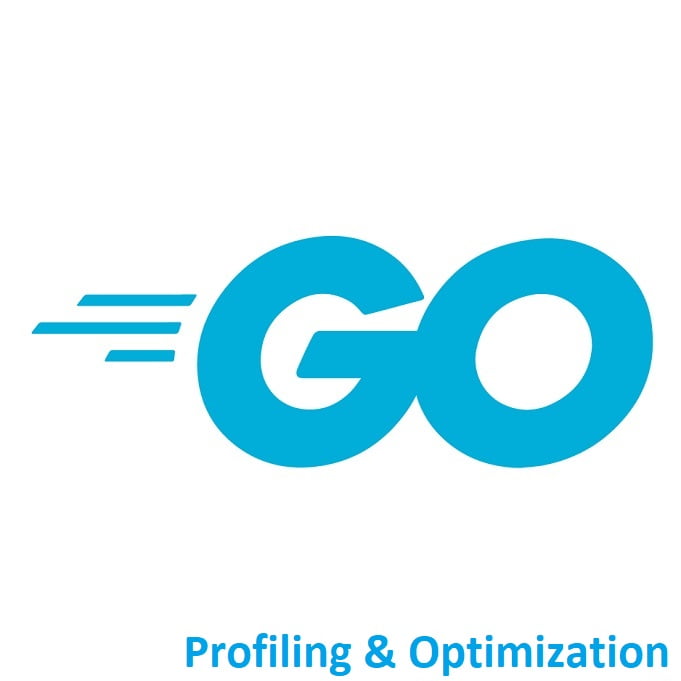
Mastering Golang: Profiling and Optimization
Enhancing Performance! Welcome to the world of Golang! Profiling and optimization are critical for maximizing the efficiency and performance of…
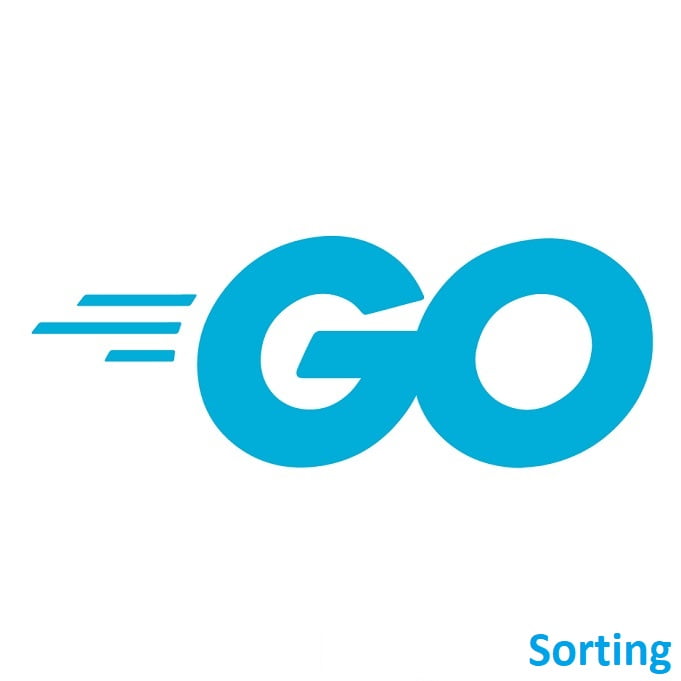
Mastering Golang: Sorting Algorithms
Efficient Element Arrangement! Welcome to the world of Golang! You can sort data easily using the built-in sort package, which…
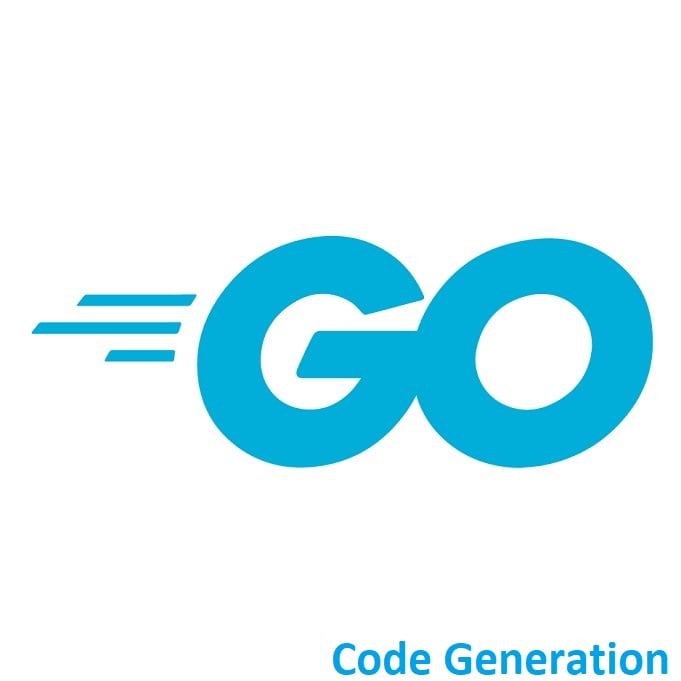
Mastering Golang: Code Generation
Automated Code Generation Techniques! Welcome to the world of Golang! Code generation is a technique where you write code to…
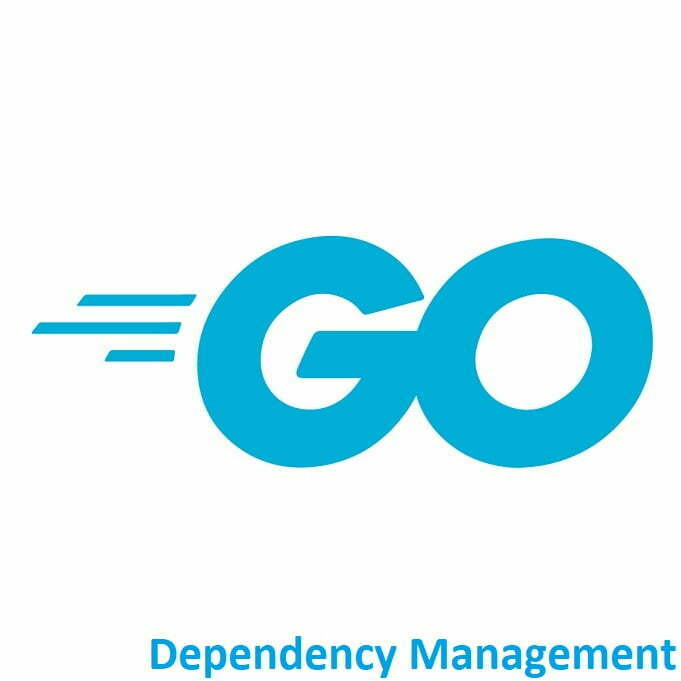
Mastering Golang: Dependency Management
Dependdency Managemnet with Go Modules! Welcome to the world of Golang! In Go, there is a built-in dependency management system…
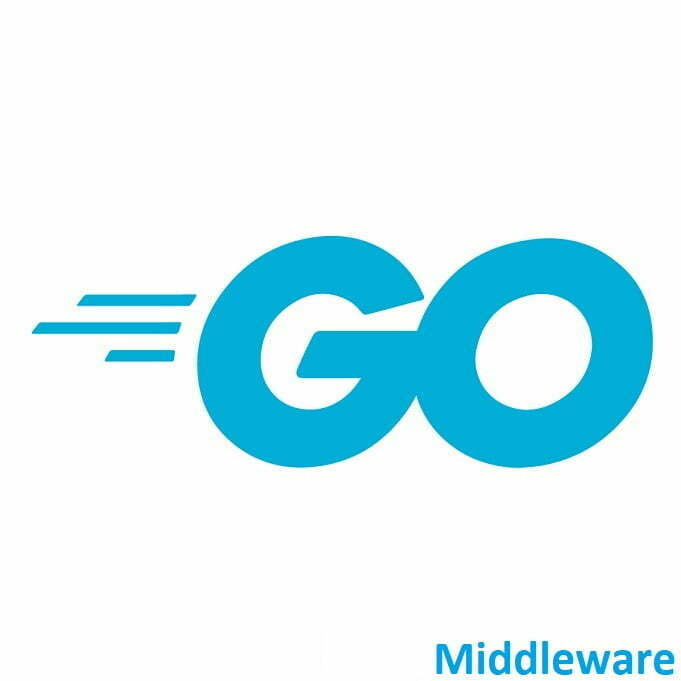
Mastering Golang: Understanding Middleware
Enhancing HTTP Request Handling! Welcome to the world of Golang! Middleware is a common pattern used for handling HTTP requests…
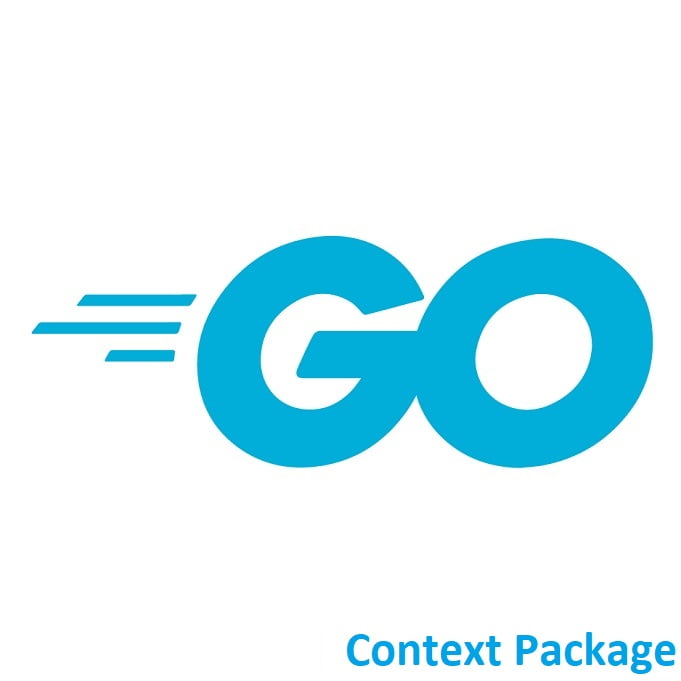
Mastering Golang: Context Package
Managing Timeouts, Cancellations, and Values! Welcome to the world of Golang! The context package is a part of the standard…
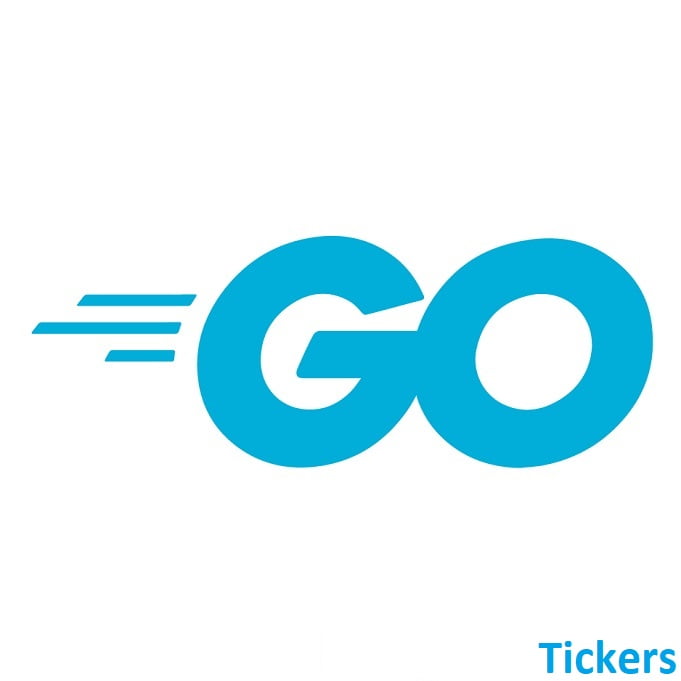
Mastering Golang: Understanding Tickers
Automating Tasks at Regular Intervals! Welcome to the world of Golang! A ticker is a built-in mechanism that allows you…
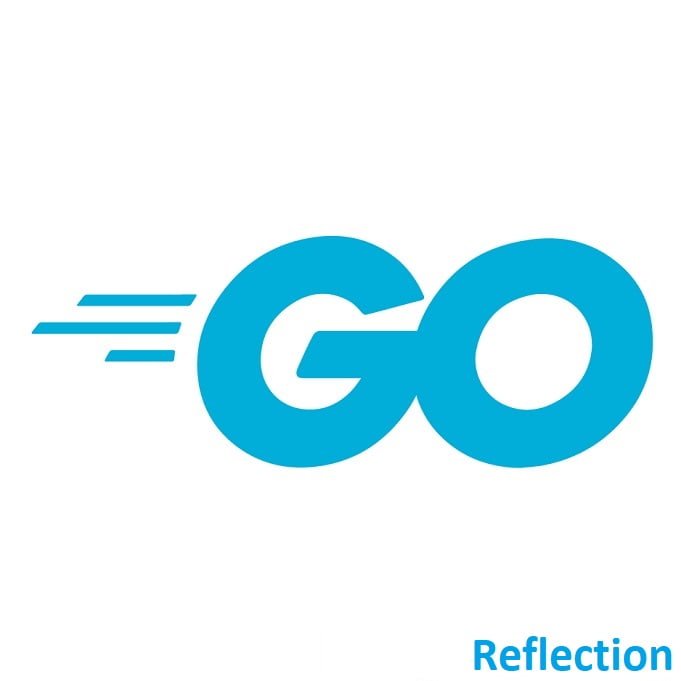
Mastering Golang: Understanding Reflection
Runtime Introspection and Dynamism! Welcome to the world of Golang! Reflection is a powerful but often discouraged feature because it…
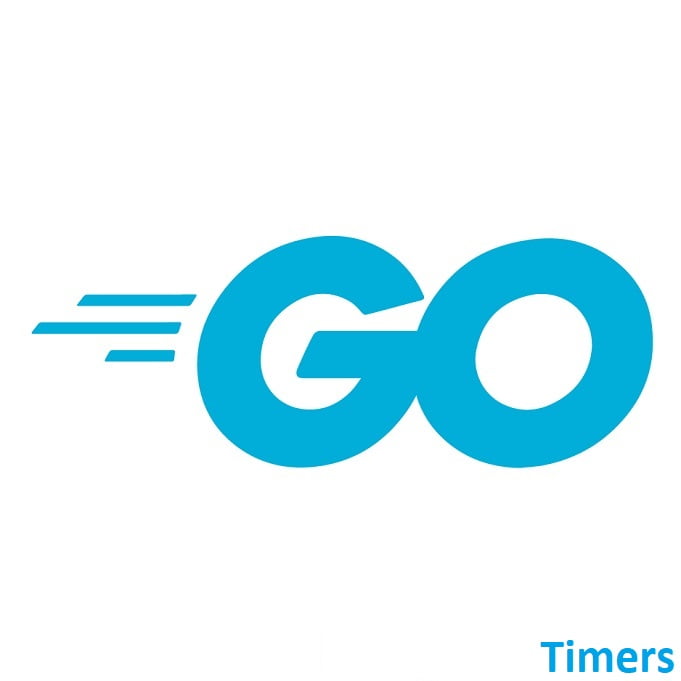
Mastering Golang: Understanding Timers
Precision Timing for Efficient Code Execution Welcome to the world of Golang! Timers are a way to schedule the execution…
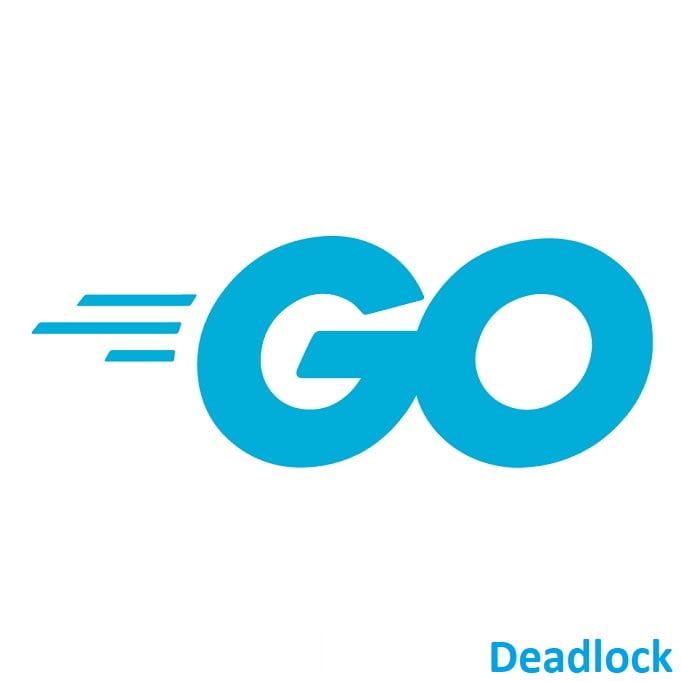
Mastering Golang: Understanding Deadlock
Navigating the Perils of Deadlocks for Reliable Concurrent Systems! Welcome to the world of Golang! A deadlock in a program occurs…
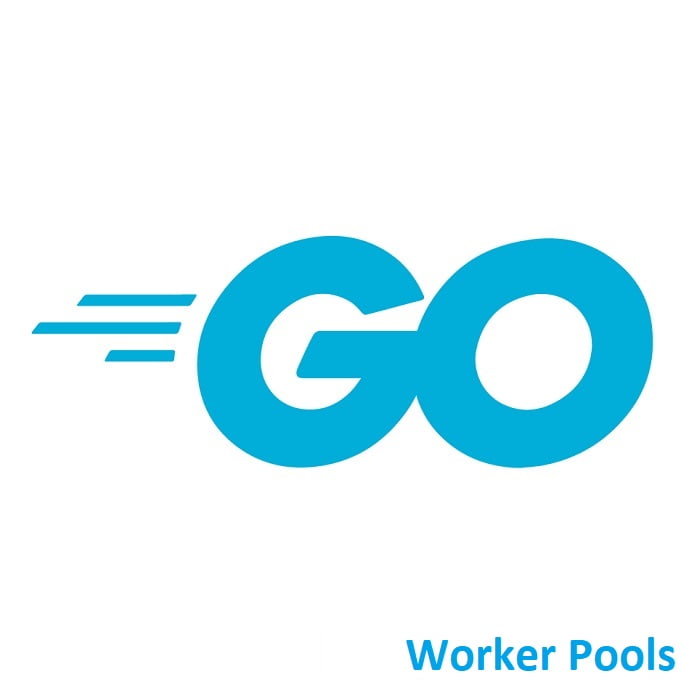
Mastering Golang: Worker Pools
Optimizing Parallel Processing! Welcome to the world of Golang! A Worker pool is a common concurrency pattern used to manage…
- 1
- 2
- 3
- …
- 7
- Next Page »