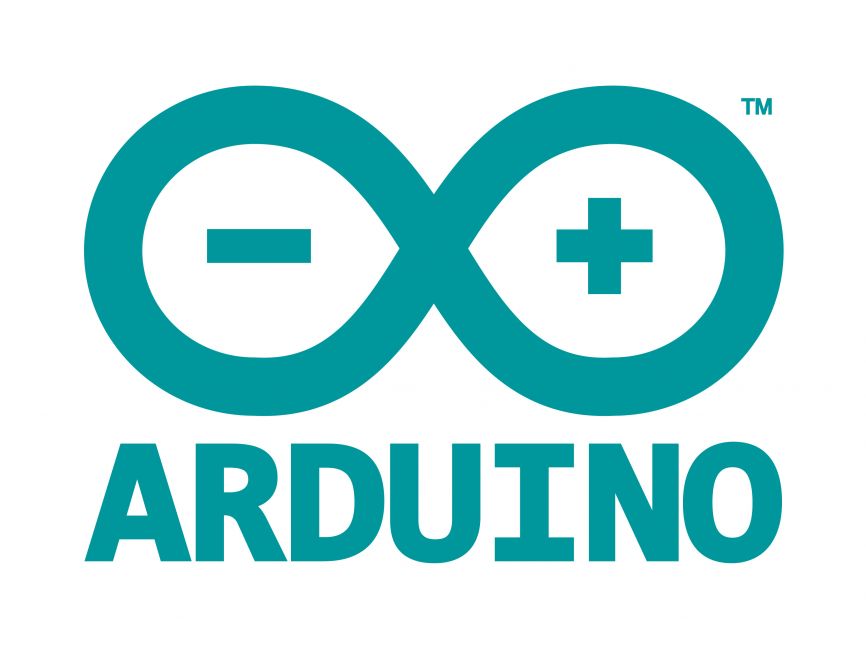
Welcome to the World of Sensors and Modules with Arduino!
The SMD RGB LED Module brings vibrant illumination to your Arduino projects, allowing you to paint your creations with an array of dazzling colors. Whether you’re designing mood lighting for your room, crafting interactive displays, or adding visual feedback to your projects, RGB LED Modules offer endless possibilities for creative expression. In this sensors and modules guide, we’ll explore its working principle, key features, applications, and how it can enhance your Arduino projects.
If you’re new to Arduino, why not take a look at our Getting Started with Arduino guides. These guides are designed for beginners to learn the fundamental basics of Arduino programming.
What is SMD?
“SMD” stands for “Surface Mount Device.” It refers to electronic components that are designed to be mounted directly onto the surface of a printed circuit board (PCB), rather than being inserted through holes in the board like traditional through-hole components. SMD components are smaller, lighter, and more suitable for automated assembly processes, making them widely used in modern electronics manufacturing. In the context of the “SMD RGB Module” you mentioned earlier, it indicates that the RGB LEDs on the module are surface-mount components.
How the SMD RGB LED Works
The SMD RGB LED Module operates on the principle of additive color mixing, where different combinations of red, green, and blue light produce a wide spectrum of colors. By varying the intensity of each color channel using PWM signals, users can create millions of color combinations. The common cathode pin is connected to ground (GND), while the individual color pins are connected to digital PWM pins on the Arduino.
Features and Specifications:
- Functionality: The SMD RGB LED Module consists of surface-mount LEDs (SMD LEDs) capable of emitting red, green, and blue light. It enables users to produce a wide range of colors by mixing these primary colors.
- Operating Voltage: 3.3V ~ 5V.
- Control: The module can be controlled through pulse-width modulation (PWM) signals from the Arduino, allowing for precise control over the intensity of each color channel.
- Connection: The module typically has four pins: one for the common cathode (negative) and three pins for the individual red, green, and blue color channels.
- Compatibility: This module is also compatible with other devices like the Raspberry Pi, ESP32, and ESP8266 etc…
The operating voltage for each color of the LEDs in an RGB LED module typically depends on the specific type of LEDs used. However, common RGB LEDs usually have similar voltage requirements for each color channel. In most cases, the forward voltage drop for each color LED is around 2 to 2.5 volts.
Here’s a general guideline for each color channel in RGB LEDs:
- Red LED:
- Operating Voltage: 1.8 to 2.1V
- Color: 620-625 nm
- Green LED:
- Operating Voltage: 3.0 to 3.2V
- Color: 520-525 nm
- Blue LED:
- Operating Voltage: 3.0 to 3.2V
- Color: 465-470 nm
It’s important to note that these are values are from the SMD RGB LED Module supplied with the Elegoo 37 Sensor Kit V2 datasheet. The actual operating voltage of your SMD RGB LED may vary depending on the specific LED model, manufacturer.
Necessary Equipment:
- Arduino (e.g., Arduino Uno)
- SMD RGB LED Module
- 220Ω Resistor x3
- Jumper wires
- Breadboard
Pin Configuration
Connecting the SMD RGB LED Module to an Arduino is fairly simple. The connections are as follows:
- B on the RGB LED Module to Digital pin 11 on the Arduino.
- G on the RGB LED Module to Digital pin 10 on the Arduino.
- R on the RGB LED Module to Digital pin 9 on the Arduino.
- – on the RGB LED Module to GND on the Arduino.
Pin labels may vary.
We connect the SMD RGB LED Module to PWM pins on the Arduino. These pins can be identified by the (~) symbol next to their corresponding numbers. This is so we can adjust the intensity level (0-255) for each color.
Important: We use current limiting resistors between each of the color pins and the Arduino pins to avoid permanent damage to the LEDs. The operating voltage of each color is slightly different, we can use different value resistors to make the color intensity the same across the colors or you can adjust the intensity values in the code. I have kept the resistors the same value in this guide to keep it as simple as possible.
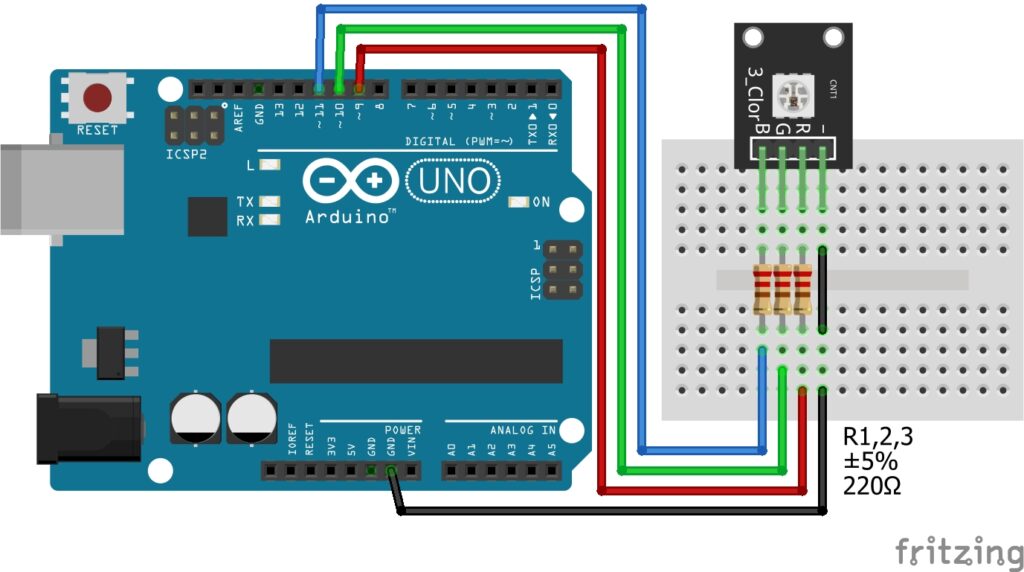
KY-009 Full Color RGB LED SMD Module Fritzing Part is created by arduinomodules.info and published under Creative Commons Attribution-ShareAlike 4.0 International license
Arduino Code Example
// Define the pins for the SMD RGB Module const int redPin = 9; // Connect the red pin to this PWM pin const int greenPin = 10; // Connect the green pin to this PWM pin const int bluePin = 11; // Connect the blue pin to this PWM pin void setup() { // Set the RGB pins as outputs pinMode(redPin, OUTPUT); pinMode(greenPin, OUTPUT); pinMode(bluePin, OUTPUT); } void loop() { // Set RGB LED to red color setColor(255, 0, 0); // Full intensity red, no green, no blue delay(1000); // Stay red for 1 second // Set RGB LED to green color setColor(0, 255, 0); // No red, full intensity green, no blue delay(1000); // Stay green for 1 second // Set RGB LED to blue color setColor(0, 0, 255); // No red, no green, full intensity blue delay(1000); // Stay blue for 1 second } // Function to set the RGB LED color void setColor(int red, int green, int blue) { analogWrite(redPin, red); // Set intensity of red LED analogWrite(greenPin, green); // Set intensity of green LED analogWrite(bluePin, blue); // Set intensity of blue LED }
Breaking Down the Code
Pin Definitions
- The code begins by defining three integer constants
redPin
,greenPin
, andbluePin
, which represent the PWM pins connected to the red, green, and blue channels of the RGB LED Module, respectively. - These pins are where the Arduino sends PWM signals to control the intensity of each color channel.
Setup Function
- The
pinMode()
function is used to configure theredPin
,greenPin
, andbluePin
as output pins. This prepares them to receive PWM signals to control the RGB LED.
Loop Function
- The code repeatedly sets the RGB LED to three different colors: red, green, and blue, with each color displayed for 1 second.
- For each color:
- The
setColor()
function is called with specific intensity values for red, green, and blue, determined by the arguments passed to the function. - A
delay()
of 1000 milliseconds (1 second) is added to keep the LED in that color for the specified duration.
- The
Color Function
- The
setColor()
function is responsible for setting the intensity of each color channel of the RGB LED. - It takes three integer arguments:
red
,green
, andblue
, representing the intensity of each color channel. The values range from 0 (off) to 255 (full intensity). - Inside the function:
analogWrite()
is used to send PWM signals to theredPin
,greenPin
, andbluePin
to control the intensity of each color channel.- The intensity values passed to
analogWrite()
determine the brightness level of each color.
Applications and Usage Scenarios
Decorative Lighting
The RGB is commonly used for creating colorful lighting effects in DIY projects, artworks, and decorative displays.
Indicators and Signage
It can serve as indicators or status lights in various applications, such as signage, control panels, or user interfaces.
Visual Feedback
By changing colors based on sensor inputs or system status, the module provides visual feedback to users in interactive projects.
Mood Lighting
It’s ideal for mood lighting setups, allowing users to adjust the ambiance and atmosphere by selecting different colors.
Conclusion
In this sensors and modules guide, we explain how the SMD RGB LED Module offers a compact, and versatile solution for adding vibrant and dynamic lighting effects to Arduino projects. By understanding its features, operation, and integration with Arduino, users can unleash their creativity and illuminate their projects with an array of captivating colors.
Discover the endless possibilities for Arduino projects with more of our Sensors and Modules guides.
I could not refrain from commenting. Very well written!