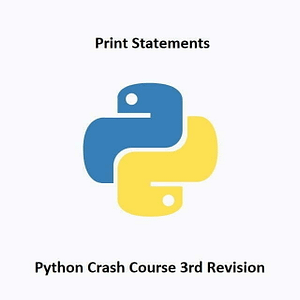
Mastering Print Statements for Beginners
Welcome to the next chapter of our Python Crash Course! In this section, we’re diving into the core of Python programming with the versatile and essential ‘print’ statement. Mastering the ‘print’ command is crucial; it’s your gateway to displaying information, debugging code, and communicating with users.
We’ll cover the basic syntax of the ‘print’ statement, explore its various formatting options, and demonstrate how it can be used creatively to output text, variables, and more. By the end of this section, you’ll have a solid grasp of ‘print’ and its myriad capabilities.
What is a Print Statement?
In Python, the print
statement is a built-in function used to display output to the console or terminal. It allows you to output text, variables, or other data types for debugging, informational purposes, or general display.
Basic Syntax of a Print Statement
The basic syntax of the print
statement in Python is as follows:
print(object(s), sep=' ', end='\n', file=sys.stdout, flush=False)
Let’s break down the components of this syntax:
object(s)
: This is the item or items you want to display. It can be a string, a variable, or multiple objects separated by commas.sep
: This is an optional parameter that specifies the separator between the objects. The default value is a space.end
: This is an optional parameter that defines what character(s) should be printed at the end. The default value is a newline character (\n
), which means a new line will be started after the print statement. You can change it to a different character or an empty string if you want to suppress the newline.file
: This is an optional parameter that specifies the file object where the output will be printed. By default, it is set tosys.stdout
, which represents the console or terminal.flush
: This is an optional parameter that determines if the output should be flushed immediately. By default, it is set toFalse
, meaning the output is buffered and written when necessary.
Hello World!
"Hello, world!"
is a phrase commonly used in programming to illustrate the basic syntax and functionality of a programming language. It serves as a simple starting point for beginners to learn how to display output on the screen or console.
When someone says “Hello, world!” in the context of programming, it usually refers to a program or code snippet that prints this phrase as output. The “Hello, world!” program is often the first program a beginner writes in a new programming language. In Python, a “Hello, world!” program can be written using the print statement, like this: print("Hello World!")
When you run this program, it will output the phrase “Hello, world!” to the console or terminal like in the image shown below.
The “Hello, world!” program is simple but important because it helps beginners understand the basic structure of a program and how to display output. It allows them to verify that their development environment is set up correctly and that they can run a program successfully.
Open your Windows Command Prompt and enter the following command:
python
Now with the Python terminal open enter the following command:
print("Hello World!")
To exit from the Python terminal back to the command prompt enter the following command:
exit()
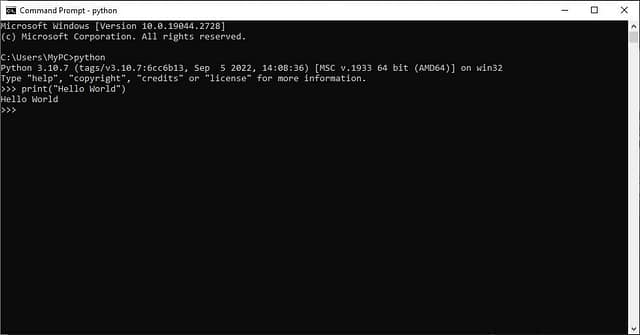
More Examples
Here are a few examples of using the print
statement in Python:
name = "Alice" age = 25 print("My name is", name) # Output: My name is Alice print("I am", age, "years old") # Output: I am 25 years old
print("Hello, world!") # Output: Hello, world!
print("Hello", "world", sep="-") # Output: Hello-world
print("Hello", "world", end="!") # Output: Hello world!
These examples demonstrate the basic usage of the print
statement in Python. You can use it to display any value or expression you want, making it a useful tool for debugging and displaying information during program execution.
Print Statements Quiz
Test Completed
Your final score: 0 / 0
Conclusion
Congratulations! You’ve now grasped the foundational concept of the ‘print’ statement, a cornerstone of Python programming. Armed with this knowledge, you can effortlessly display output, debug code, and communicate effectively within your programs.
Remember, the ‘print’ statement is not just about showing text on the screen; it’s a versatile tool offering various formatting options and possibilities. As you progress in this Python crash course, we’ll experiment with different ways to use ‘print’ creatively. Explore its capabilities to display variables, format output, and make your programs more user-friendly.
In the next installment of this Python Crash Course, we will be learning all about Comments
That’s All Folks!
You can explore more of our Python guides here: Python Guides