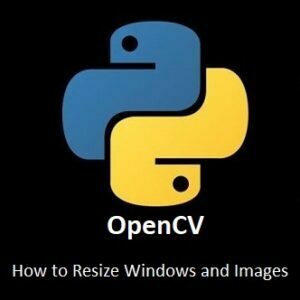
Dynamic Window and Frame Resizing Techniques
Welcome to the next chapter of OpenCV with Python mastery! In this guide, we dive into the art of resizing windows and resizing images, a fundamental skill for any computer vision enthusiast. Whether you’re optimizing display, enhancing performance, or adapting to different screen dimensions, resizing is a key ingredient in the recipe for visual perfection. Join us as we unravel the secrets of dynamic resizing in OpenCV and empower your projects with flexible and responsive visual elements.
What’s Covered:
- Explaining the
resize()
function. - Resizing Images.
- Resizing Windows.
Explaining resize()
In OpenCV, the resize()
function is used to resize images or change their dimensions. It allows you to specify the desired width and height of the output image. The function has the following syntax:
resized_image = cv2.resize(src, dsize[, dst[, fx[, fy[, interpolation]]]])
Here is a breakdown of the parameters:
src
: This is the source image that you want to resize. It can be a NumPy array or an OpenCV Mat object.dsize
: This parameter specifies the desired width and height of the output image. It can be either a tuple(width, height)
or an integer value representing the width. The height is then calculated based on the aspect ratio of the source image.dst
(optional): This is the output image object. If provided, the resized image will be stored in this object. If not provided, a new object will be created.fx
(optional): This parameter is used to scale the width of the source image. It is a floating-point value that represents the scaling factor along the horizontal axis. If bothfx
andfy
are set to 0, thedsize
parameter will be used to specify the output size.fy
(optional): This parameter is used to scale the height of the source image. It works similar tofx
, but it represents the scaling factor along the vertical axis.interpolation
(optional): This parameter specifies the interpolation method to be used for resizing. It can take different values, such ascv2.INTER_LINEAR
,cv2.INTER_NEAREST
,cv2.INTER_CUBIC
, etc. By default,cv2.INTER_LINEAR
is used.
Resizing Images
Here’s a basic Python example how to use the resize()
function to resize an image:
import cv2 # Load the image image = cv2.imread('input.jpg') # Resize the image to a specific width and height resized_image = cv2.resize(image, (400, 300)) # Display the resized image cv2.imshow('Resized Image', resized_image) cv2.waitKey(0) cv2.destroyAllWindows()
In the above example, the resize()
function is used to resize the image to a width of 400 pixels and a height of 300 pixels. The resized image is then displayed using OpenCV’s imshow()
function.
Note that when resizing images, it’s important to maintain the aspect ratio to avoid distortion. If you want to resize an image while preserving the aspect ratio, you can calculate one dimension based on the other and the original aspect ratio.
Resizing Windows
In OpenCV, we can use the resizeWindow()
and namedWindow()
functions to control how windows are created. The resizeWindow()
function takes three parameters, a label for the window and then the height and width you want the window to be. You can use the namedWindow()
function to create a named window with a specific size and then resize the content displayed in that window. The namedWindow()
function has a few options:
cv2.WINDOW_NORMAL
will allow for you to set the window size.cv2.WINDOW_AUTOSIZE
will automatically set the windows size (Default).cv2.WINDOW_FULLSCREEN
will set the window to Fullscreen.
Here’s a basic Python example to resizing windows:
import cv2 # Create a named window cv2.namedWindow('My Window', cv2.WINDOW_NORMAL) # Set the window size cv2.resizeWindow('My Window', 800, 600) # Load and display an image in the window image = cv2.imread('input.jpg') cv2.imshow('My Window', image) cv2.waitKey(0) cv2.destroyAllWindows()
In this example, the namedWindow()
function is used to create a named window called ‘My Window’. The WINDOW_NORMAL
flag is passed to allow resizing of the window. Then, the resizeWindow()
function is used to set the size of the window to 800 pixels wide and 600 pixels high.
Afterward, an image is loaded and displayed in the named window using the imshow()
function.
Keep in mind that not all window managers support manual resizing of OpenCV windows. In some cases, the window may be displayed with a fixed size, ignoring the size set by resizeWindow()
.
Conclusion
Congratulations on mastering the art of resizing in OpenCV! You’ve now equipped yourself with the skills to dynamically adapt frames and windows, opening doors to a world of versatility and optimization. As you integrate these techniques into your projects, remember that resizing is not just about dimensions; it’s about enhancing user experience and optimizing computational resources. Continue to experiment, innovate, and resize your way to visually stunning Python applications!
In our next OpenCV for Beginners guide we will be learning all about Morphological Operations
That’s All Folks!
You can find all of our OpenCV guides here: OpenCV for Beginners