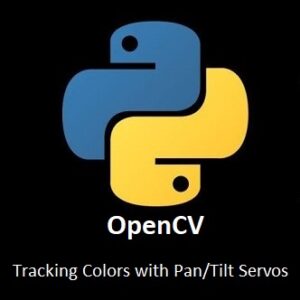
Dynamic Color Recognition and Tracking
Step into the vibrant world of dynamic vision as we explore the intricacies of color tracking using OpenCV with Python and pan/tilt servos. In this guide, we’ll unravel the secrets of recognizing and tracking colors in real-time, empowering your projects with the ability to interact with a spectrum of hues. From setting up the hardware to implementing sophisticated color tracking algorithms, this tutorial is your gateway to injecting life and responsiveness into your vision-based applications. Let’s dive into the fascinating realm of chromatic precision!
What’s Covered?
- What is Color Tracking?
- The Color Tracking Program.
What is Color Tracking?
Color tracking with OpenCV involves using computer vision techniques to track objects of a specific color using OpenCV and controlling servos based on the tracking results. It combines the ability to detect and track colored objects using OpenCV with the capability to physically move servos to follow the detected objects in the real world.
Here’s a general overview of the color tracking process with OpenCV and servos:
Capture a Video Frame:
Use OpenCV’s video capturing functions to acquire a video frame from a camera or a pre-recorded video.
Preprocessing:
Apply any necessary preprocessing steps to the frame, such as resizing, blurring, or converting the color space to improve color detection accuracy.
Color Detection and Object Tracking:
Convert the frame to a suitable color space, such as HSV, and define a color range or threshold for the desired color you want to track. Use OpenCV’s thresholding or masking techniques to create a binary mask of the pixels within the specified color range. Then, apply contour detection algorithms to identify connected regions or blobs representing potential objects of the desired color. Use tracking algorithms like centroid tracking or Kalman filtering to track the movement of these objects over subsequent frames.
Servo Control:
Based on the tracking results, calculate the position or movement required for the servos to follow the detected object. Servos are motorized devices commonly used for controlling the position of mechanical systems. They can be connected to mechanical structures like a pan-tilt mechanism, allowing them to move in two dimensions. Use appropriate libraries or interfaces to control the servos, such as the NVidia Jetson Nano GPIO, Raspberry Pi GPIO or Arduino servo libraries.
Update Servo Positions:
Continuously update the servo positions based on the tracking results. Adjust the position of the servos in real-time to keep the tracked object centered or within a desired region of the camera frame.
Repeat the Process:
Continuously process subsequent frames, detect and track the desired colored object, and adjust the servo positions accordingly.
By integrating OpenCV for color detection and object tracking with servo control mechanisms, you can create a system that automatically follows objects of a specific color. This can be used in various applications like object tracking cameras, robotics, or automated systems that require visually guided servo control.
Sample Code
The Color Tracking Program
I’ll not go into detail about the code as you should know what most of this is doing by now, but i have added comments for the areas you should focus on. Pay special attention to the code within the #IMPORTANT#
code block, this is where you can change the color to track between red, green and blue. The Python code below is preset to track on any object that is red. You may find it necessary to edit the color ranges for each color to suit your environment. If you want to track a different color that is not specified, you will need to create a new color range block.
Open a new file in Visual Studio, then copy and paste the code below. Run the code and your camera will any color that its set for. Be mindful, if you have a lot of color in and around your room the camera may jump from object to object.
Python Code:
from adafruit_servokit import ServoKit import numpy as np import time import cv2 #Servo Settings kit=ServoKit(channels=16) pan=45 tilt=135 kit.servo[0].angle=pan kit.servo[1].angle=tilt #Camera settings width=640 height=480 camera = cv2.VideoCapture(0) camera.set(cv2.CAP_PROP_FRAME_WIDTH,width) camera.set(cv2.CAP_PROP_FRAME_HEIGHT,height) #Defined the color ranges for detection #Red color range redLower = np.array([136, 87, 111], np.uint8) redUpper = np.array([180, 255, 255], np.uint8) redColor = (0,0,255) #Blue color range blueLower = np.array([100, 100, 100], np.uint8) blueUpper = np.array([140, 255, 255], np.uint8) blueColor = (255,0,0) #Green color range greenLower = np.array([36, 25, 100], np.uint8) greenUpper = np.array([70, 255, 255], np.uint8) greenColor = (0,255,0) ####### IMPORTANT ########### #Edit these lines to change color for detection #Keep chosen color consistent #Lower range: redLower, greenLower, blueLower lower=redLower #Upper range: redUpper, greenUpper, blueUpper upper=redUpper #Bounding box: redColor, greenColor, blueColor color=redColor #Label bounding box: 'Red', 'Green', 'Blue' name='Red' ############################### while True: _, frame = camera.read() hsvFrame = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV) mask = cv2.inRange(hsvFrame, lower, upper) kernel = np.ones((5, 5), "uint8") colorMask = cv2.dilate(mask, kernel) res_color = cv2.bitwise_and(frame, frame, mask = colorMask) contours, hierarchy = cv2.findContours(mask, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE) for contour in contours: area = cv2.contourArea(contour) if(area > 300): x, y, w, h = cv2.boundingRect(contour) frame = cv2.rectangle(frame, (x, y),(x + w, y + h),color, 2) cv2.putText(frame, name+" Detected", (x, y), cv2.FONT_HERSHEY_SIMPLEX, 1,color,2) #Move Servo Tracking Position to Follow Color objX=x+w/2 objY=y+h/2 errorPan=objX-width/2 errorTilt=objY-height/2 if abs(errorPan)>15: pan=pan-errorPan/50 if abs(errorTilt)>15: tilt=tilt+errorTilt/50 if pan>180: pan=180 print('pan out of range') if pan<0: pan=0 print('pan out of range') if tilt>180: tilt=180 print('tilt out of range') if tilt<0: tilt=0 print('tilt out of range') kit.servo[0].angle=pan kit.servo[1].angle=tilt break cv2.imshow("Color Detection", frame) if cv2.waitKey(1)== ord('q'): break camera.release() cv2.destroyAllWindows()
Conclusion
Congratulations, color maestro! You’ve successfully ventured into the captivating world of color tracking using OpenCV with Python. By mastering the nuances of dynamic color recognition, your projects are now equipped to respond intelligently to a spectrum of hues. This marks a pivotal step in creating interactive, vibrant applications.
As we conclude this color-tracking expedition, stay tuned for upcoming posts where we’ll explore even more dimensions of dynamic vision.
In the next installment of our OpenCV for Beginners guide we will be learning how to Manually Control the Cameras Field of View
That’s All Folks!
You can find all of our OpenCV guides here: OpenCV for Beginners