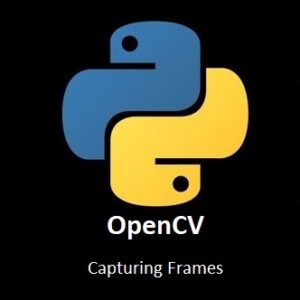
From Pixels to Motion: A Guide to Frame Capture in OpenCV
Step into the captivating world of computer vision as we explore the fundamentals of capturing frames with OpenCV. In this guide, we’ll unravel the essence of frame acquisition, the building block of visual analysis. Whether you’re diving into image processing, video analysis, or motion detection, understanding frame capture is the key to unlocking the potential of OpenCV. Let’s embark on a journey where pixels come to life, and every frame tells a story.
In this guide I am going to show you how to capture a frame from your webcam and save the frame as either an image or video.
Capturing Frames
Open your code-oss program, let’s begin!
If you have not connected your webcam to the Jetson Nano yet, now is a good time to do so.
Create a new Python script file in the Python-AI directory by hovering over the directory in code-oss, and click on the first icon “New File” you can name it however you want but make it something recognizable like “basicCapture.py”. The “.py” is the most important part, as this allows the code-oss Python interpreter to recognize it will be working with a valid Python script.
Now copy and paste the code below to the new Python file you just created.
import cv2 width=640 height=480 camera=cv2.VideoCapture(0) camera.set(cv2.CAP_PROP_FRAME_WIDTH,width) camera.set(cv2.CAP_PROP_FRAME_HEIGHT,height) while True: ret,frame=camera.read() cv2.imshow('Camera Stream',frame) if cv2.waitKey(1)==ord('q'): break camera.release()
cv2.destroyAllWindows()
When you’re ready, right click the code window and select Run Python File in Terminal
Breaking Down the Code
- First we import the OpenCV library so we can use its functions.
import cv2
- Then we set our screen size to 640 x 480.
width=640 height=480
You can play around with different screen resolutions like 1080×720 for instance, but in upcoming projects we will be using the smaller screen size as it will help the code to run quicker. The Larger the screen size, the more pixels are used. Computer Vision processes every single pixel, so more pixels mean more data to process.
- We create the camera object.
camera=cv2.VideoCapture(0)
I have set my camera ID to (0). Remember, your camera ID maybe different.
- Then we set our predefined width and height into the camera object.
camera.set(cv2.CAP_PROP_FRAME_WIDTH,width) camera.set(cv2.CAP_PROP_FRAME_HEIGHT,height)
- We create a while loop to continuously loop the following code which is indented within the while loop block.
- Then we grab a frame from the camera object.
- We display the frame with OpenCV’s imshow function.
- Then we display it in a window I labelled ‘Camera Stream’.
The window can be titled anything that you wish, and we insert the captured frame into that window.
- We use OpenCV’s waitKey function within an if statement, to create a condition so we can end the program, if the ‘q’ key is pressed the code will break out of the loop.
while True: ret,frame=camera.read() cv2.imshow('Camera Stream',frame) if cv2.waitKey(1)==ord('q'): break
- We release the camera.
camera.release()
- We close the window.
cv2.destroyAllWindows()
The functions used above will be used often, please make sure you understand everything explained.
Troubleshooting
If you were unsuccessful then you may need to alter the webcams id number within the fourth line of code.
Edit this line:
camera=cv2.VideoCapture(0)
To this:
camera=cv2.VideoCapture(1)
Now re-run the code again. If you still have a problem, double check you copied the code correctly then try again. If the problem still persists, please send me a message in the comment section below, I’m always happy to help.
Creating and Saving Images
Create a new .py file in the Python-AI directory. remember, you can name it how you want but make it something recognizable like “saveCapture.py”.
Now copy and paste the code below to the new Python script you just created.
When you’re ready, right click the code window and select Run Python File in Terminal
import cv2 import os width=640 height=480 camera=cv2.VideoCapture(0) camera.set(cv2.CAP_PROP_FRAME_WIDTH,width) camera.set(cv2.CAP_PROP_FRAME_HEIGHT,height) ret,frame=camera.read() cv2.imshow('Capture',frame) cv2.imwrite('capture.jpg',frame) while True: if cv2.waitKey(1)==ord('q'): break camera.release() cv2.destroyAllWindows()
Here we have only two new lines of code to breakdown:
- Importing the os library allows us to store the image to our system.
import os
- Creating image of captured frame.
cv2.imwrite('capture.jpg',frame)
Creating and Saving Video
Create a new .py file in the Python-AI directory, label it something like “saveVideoCapture.py”. Now copy and paste the code below to the file you just created. When you’re ready, right click the code window and select Run Python File in Terminal
import cv2
width=640 height=480 camera=cv2.VideoCapture(0) camera.set(cv2.CAP_PROP_FRAME_WIDTH,width) camera.set(cv2.CAP_PROP_FRAME_HEIGHT,height) SaveVideo=cv2.VideoWriter('video.avi',cv2.VideoWriter_fourcc(*'XVID'), 21, (width, height)) while True: ret, frame=camera.read() cv2.imshow('Video Stream',frame) SaveVideo.write(frame) if cv2.waitKey(1)==ord('q'): break camera.release() SaveVideo.release()
Breaking Down the Code
Here we have three new lines of code to breakdown.
The line below is the most important, here we store the video label, the video codec, and the frame size into an object we called SaveVideo.
SaveVideo=cv2.VideoWriter('video.avi',cv2.VideoWriter_fourcc(*'XVID'), 21, (width, height))
Next, we write the captured frame to the SaveVideo object.
SaveVideo.write(frame)
Then we release the SaveVideo object, this makes sure it closed properly and doesn’t corrupt the video file we have just created.
SaveVideo.release()
Conclusion
Congratulations! You’ve mastered the art of frame capture with OpenCV, laying the foundation for countless possibilities in computer vision. From freezing moments in time to analyzing dynamic sequences, the ability to capture and manipulate frames opens doors to a myriad of applications. As you conclude this guide, envision the exciting projects you can now undertake, armed with the knowledge to harness the visual power of OpenCV. The world of frames is yours to explore, what will you create next?
In our next OpenCV for Beginners guide we will dive deeper in using the waitkey() function.
That’s All Folks!
You can find all of our OpenCV guides here: OpenCV for Beginners