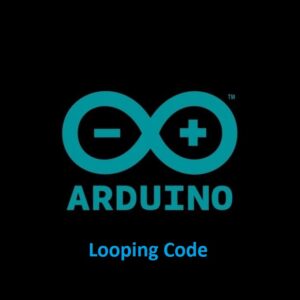
Understanding The Different Types of Loops
In Arduino programming, looping code is commonly used to execute a block of code repeatedly. They are a fundamental control structure for iterating through sequences of code, making them ideal for tasks like reading sensors, controlling actuators, or performing any repetitive operation.
In coding languages, we have the for loop
, while loop
, and do-while loop
. The for loop, loops a specified known number of times. The while loop loops an unknown number of times until a condition is met. The do-while loop works in the same way as the while loop except the do while loop executes the code before the loop begins this way the code is always executed at least once.
For Loops
The basic syntax of a for loop in Arduino is as follows:
for (initialization; condition; increment/decrement) {
// Code to be executed repeatedly
}
Here’s an in-depth explanation of each part of the for loop:
-
Initialization: This is where you initialize a variable and set its initial value. This step is executed only once at the beginning of the loop. It’s typically used to declare a loop control variable (e.g.,
int i = 0;
) that keeps track of the loop’s progress. -
Condition: The condition is a Boolean expression that is checked before each iteration of the loop. If the condition evaluates to
true
, the loop continues to execute; otherwise, it exits. If the condition isfalse
initially, the loop won’t execute at all. -
Increment/Decrement: This part specifies how the loop control variable is modified after each iteration. You can use operators like
++
(increment by one) or--
(decrement by one), or you can perform more complex operations. This step is executed at the end of each iteration, just before re-evaluating the condition.
Here’s an example of a simple for loop in Arduino that blinks an LED 5 times:
const int ledPin = 13; // Pin connected to the LED void setup() { pinMode(ledPin, OUTPUT); // Set the LED pin as an output } void loop() { for (int i = 0; i < 5; i++) { digitalWrite(ledPin, HIGH); // Turn the LED on delay(1000); // Wait for 1 second digitalWrite(ledPin, LOW); // Turn the LED off delay(1000); // Wait for 1 second } // The loop will repeat until i is no longer less than 5. }
In this example:
int i = 0
initializes a variablei
to 0.i < 5
is the condition. The loop will continue executing as long asi
is less than 5.i++
incrementsi
by 1 after each iteration.
So, the LED will blink on and off 5 times, thanks to the for loop.
While Loops
While loops in Arduino, like for loops, are used for repetitive execution of a block of code. However, unlike for loops, while loops repeat as long as a specified condition is true.
The basic syntax of a while loop in Arduino is as follows:
while (condition) { // Code to be executed repeatedly }
- Condition: This is a Boolean expression that is checked before each iteration of the loop. If the condition evaluates to
true
, the loop continues to execute; otherwise, it exits. If the condition isfalse
initially, the loop won’t execute at all.
Here’s an example of a simple while loop in Arduino that blinks an LED while a button is pressed:
const int ledPin = 13; // Pin connected to the LED const int buttonPin = 2; // Pin connected to the button void setup() { pinMode(ledPin, OUTPUT); // Set the LED pin as an output pinMode(buttonPin, INPUT); // Set the button pin as an input } void loop() { while (digitalRead(buttonPin) == HIGH) { digitalWrite(ledPin, HIGH); // Turn the LED on while the button is pressed } digitalWrite(ledPin, LOW); // Turn the LED off when the button is released }
In this example:
digitalRead(buttonPin) == HIGH
is the condition. The while loop will keep executing as long as the button is pressed (i.e., the condition is true).digitalWrite(ledPin, HIGH)
turns the LED on while the button is pressed.- When the button is released and the condition becomes false, the loop exits, and
digitalWrite(ledPin, LOW)
turns the LED off.
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Do-While Loop
A do-while
loop in Arduino is similar to a while
loop, but it guarantees that the code block will execute at least once before checking the loop condition.
The basic syntax of a do-while
loop in Arduino is as follows:
do { // Code to be executed at least once } while (condition);
Here’s an explanation of each part of the do-while
loop:
-
Code Block: This is the block of code that you want to execute repeatedly. It is executed at least once, regardless of the condition.
-
Condition: After the code block is executed, the loop checks the specified condition. If the condition evaluates to
true
, the loop continues to execute; otherwise, it exits. If the condition isfalse
initially, the loop still runs once.
Here’s an example of a simple do-while
loop in Arduino that blinks an LED for a specified duration:
const int ledPin = 13; // Pin connected to the LED const int duration = 5000; // Duration of LED blinking in milliseconds (5 seconds) void setup() { pinMode(ledPin, OUTPUT); // Set the LED pin as an output } void loop() { unsigned long startTime = millis(); // Get the current time do { digitalWrite(ledPin, HIGH); // Turn the LED on delay(500); // Wait for 500 milliseconds (0.5 seconds) digitalWrite(ledPin, LOW); // Turn the LED off delay(500); // Wait for 500 milliseconds (0.5 seconds) } while (millis() - startTime < duration); // The loop will repeat until the specified duration has passed. }
In this example:
- The code block inside the
do-while
loop blinks the LED on and off repeatedly. - The condition
millis() - startTime < duration
checks if the specified duration has passed since the loop started. millis()
is a function that returns the current time in milliseconds since the Arduino started running.millis() - startTime
calculates the elapsed time.- The loop continues as long as the elapsed time is less than the specified duration.
Conclusion
Remember that you can modify the initialization, condition, and increment/decrement parts to suit your specific needs when working with Arduino for loops. They are versatile and can be adapted to various applications in your Arduino projects.
While loops are useful when you want to repeat a block of code indefinitely or as long as a particular condition remains true. However, be cautious with while loops to avoid unintentional infinite loops that can freeze your Arduino if the condition is never met.
The do-while
loop is useful when you want to ensure that a certain block of code executes at least once, regardless of the initial condition. It’s commonly used for tasks where initialization or setup is required before entering a loop.
Recommendations:
If you don’t already own any Arduino hardware, we highly recommend purchasing the Elegoo Super Starter Kit. This kit has everything you need to start programming with Arduino.
You can find out more about this kit here: Elegoo Super Starter Kit