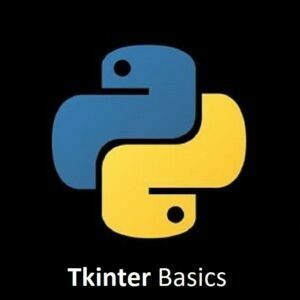
A Quick Guide to GUI Development!
Welcome to the world of graphical user interface development in Python! Tkinter stands as a robust and accessible toolkit for building intuitive and responsive GUIs. In this comprehensive guide, we’ll explore Tkinter’s functionalities, learning how to design and implement interactive user interfaces. By the end, you’ll be equipped to craft dynamic GUI applications in Python using Tkinter’s versatile features.
What is Tkinter?
Tkinter is a standard GUI (Graphical User Interface) toolkit in Python. It’s commonly used for creating desktop applications with graphical interfaces in Python. Tkinter is based on the Tk GUI toolkit, which is originally developed for the Tcl programming language.
Here are some key features of Tkinter:
Simple and Easy to Use:
Tkinter provides an intuitive and straightforward interface for creating GUI applications in Python, making it accessible for beginners.
Cross-Platform:
It is available on most Unix platforms, including macOS, Linux, and Windows, providing consistent behavior across different operating systems.
Widgets:
Tkinter offers a variety of widgets (buttons, labels, entry fields, etc.) that can be used to create interactive elements in the graphical interface.
Event-Driven Programming:
Tkinter applications are event-driven, meaning they respond to user actions (like button clicks or keypresses) by triggering functions or actions.
Customizable Layouts:
It provides different layout managers (pack, grid, place) to arrange widgets within the application window.
Integration with Python:
Being part of the Python standard library, Tkinter doesn’t require any separate installation.
Overall, Tkinter serves as a versatile and accessible toolkit for developers aiming to create graphical interfaces for their Python applications, ranging from simple tools to more complex desktop software.
How to use Tkinter
Here’s a step-by-step guide on how to use Tkinter:
Import the Tkinter module:
import tkinter as tk
Create an instance of the main Tkinter window:
window = tk.Tk()
Add Widgets
Add buttons, labels, text boxes, etc… to the window using various Tkinter classes such as Button
, Label
, Entry
, etc.:
label = tk.Label(window, text="Hello, Tkinter!") button = tk.Button(window, text="Click Me!")
Use Grid or Pack Geometry
Use the grid or pack geometry manager to position the widgets within the window:
- Grid manager example:
label.grid(row=0, column=0) button.grid(row=1, column=0)
- Pack manager example:
label.pack() button.pack()
Choose either grid or pack manager based on your layout requirements.
Add Functionality to the Widgets
Add functionality to the widgets by defining functions that will be called when events occur (e.g., button clicks)
def button_click(): print("Button clicked!") button.config(command=button_click)
Run the Program
Run the Tkinter event loop, which listens for user input and updates the GUI accordingly:
window.mainloop()
This method will keep the window open until the user closes it.
Optional
You can perform any cleanup or additional operations after the main loop exits.
Summary
That’s the basic structure of a Tkinter program. You can expand upon this foundation to create more complex GUIs with additional widgets, layout managers, and event-driven behavior. Tkinter offers many other classes and options for customization, which you can explore further in the official documentation or various online resources.
Below are some of the Python projects I used Tkinter with:
Conclusion
Congratulations on exploring the realm of Python GUI development with Tkinter! You’ve acquired the fundamental knowledge necessary to design interactive and visually engaging applications. As you continue your exploration, delve deeper into Tkinter’s widgets, event handling, and layout management. With Tkinter, you possess the tools to create compelling GUIs and elevate your Python applications.
That’s All Folks!
You can explore more of our Python guides here: Python Guides