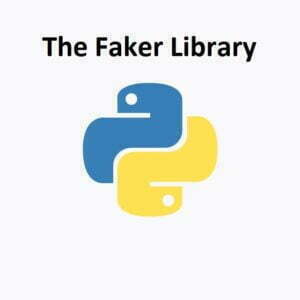
Crafting Realistic Dummy Data!
Generating realistic dummy data is vital for testing, development, and prototyping. This guide explores the capabilities of the Python Faker library, a powerful tool for generating various types of fake data. Learn how to leverage Faker to create mock datasets, test scenarios, and populate databases effortlessly.
The Python Faker library is a popular Python package used for generating fake data. It’s often used in testing, development, and data anonymization scenarios when you need realistic looking but non-sensitive data.
Here’s how you can use the Faker library in Python:
Installation:
You can install the Faker library using pip:
pip install Faker
Basic Usage:
After installation, you can create a Faker instance and use it to generate various types of fake data, such as names, addresses, emails, dates, and more. Here’s a simple example:
from faker import Faker # Create a Faker instance fake = Faker() # Generate fake data fake_name = fake.name() fake_email = fake.email() fake_address = fake.address() print(f"Name: {fake_name}") print(f"Email: {fake_email}") print(f"Address: {fake_address}")
This will generate and print fake name, email, and address values.
Localization:
You can also generate data in different languages and regions by specifying a locale when creating the Faker instance. For example:
from faker import Faker # Create a French Faker instance fake = Faker('fr_FR') # Generate French fake data fake_name = fake.name() fake_address = fake.address() print(f"Name: {fake_name}") print(f"Address: {fake_address}")
In this example, we’ve created a French Faker instance, so the generated data will be in French.
Custom Data:
You can create custom data using the Faker library by defining your own providers or using the built-in ones. For example, you can create a custom provider to generate fake data for a specific use case.
Seed for Reproducibility:
If you need to reproduce the same set of fake data, you can use the seed()
method to set a seed value for the Faker instance:
fake = Faker() fake.seed(1234) # Set a seed value
This will ensure that the generated data remains consistent across runs when the same seed is used.
Populating Databases with Faker:
Creating Faker Instances:
Start by creating an instance of the Faker class corresponding to the language/locale of the data you want to generate. For instance:
from faker import Faker fake = Faker()
Generating and Inserting Data:
Use Faker methods to generate fake data and insert it into your database. This typically involves iterating through your database model and populating each row with generated data.
# Example for SQLite using SQLAlchemy (you may use other database libraries as well) from sqlalchemy import create_engine, Table, Column, Integer, String, MetaData # Define database connection engine = create_engine('sqlite:///mydatabase.db') metadata = MetaData() # Define table structure users = Table('users', metadata, Column('id', Integer, primary_key=True), Column('name', String), Column('email', String), Column('address', String) ) # Create table in database metadata.create_all(engine) # Insert Faker-generated data into the table with engine.connect() as conn: for _ in range(10): # Generating 10 rows of data conn.execute(users.insert().values( name=fake.name(), email=fake.email(), address=fake.address() ))
Customizing Data for Specific Fields:
Faker methods can be tailored to fit specific database field requirements. For example, generating unique emails or formatting dates according to the database’s datetime format.
Considerations for Large Datasets:
When dealing with large datasets, ensure efficient memory usage by batching data insertion or using database-specific bulk insert methods for better performance.
Database Libraries and Adaptation:
SQLAlchemy, Django ORM, or Other ORMs:
Adapt the code according to the ORM or database library you’re using. The principles remain similar, but syntax and specific methods may vary.
Handling Relationships:
Faker can also assist in generating related data for tables with relationships, maintaining referential integrity during database population.
Populating databases with Faker-generated data helps simulate real-world scenarios, test data validity, and assess application performance under different data loads. Remember to consider your database schema, constraints, and relationships while generating and inserting Faker data to ensure coherence and relevance in your test datasets.
Best Practices and Tips with Python Faker:
Data Quantity and Relevance:
Generate an appropriate amount of data that aligns with your testing needs without overwhelming the system. Ensure the generated data is relevant to the scenarios you’re testing.
Data Diversity:
Utilize Faker’s wide range of providers and locales to create diverse data. This diversity aids in testing different edge cases and scenarios within your application.
Data Consistency:
Maintain consistency in the generated data across different test runs. Use Faker’s seed functionality (fake.seed()
) to ensure reproducibility, generating the same set of data for consistent testing.
Validation and Constraints:
Validate the generated data against your application’s constraints and data validation rules. Ensure the generated data fits within the defined boundaries and doesn’t violate any constraints.
Database Integrity:
When populating databases, maintain data integrity by considering relationships between tables. Use Faker to generate related data consistently to preserve referential integrity.
Efficient Memory Usage:
For large datasets, consider efficient memory handling. Use batch processing or database-specific bulk insert methods to optimize memory usage and insertion performance.
Customization and Locale Selection:
Tailor data generation by selecting appropriate locales and customizing providers to match specific data format or language requirements in your application.
Documentation and Comments:
Document complex data generation scenarios with comments or documentation to assist other developers in understanding the purpose and usage of the generated data.
Testing and Validation Iteration:
Iterate through various testing scenarios, adjusting data generation parameters and patterns based on test outcomes to refine data generation for better coverage.
Adhering to these best practices ensures that the generated test data accurately represents real-world scenarios, allowing thorough testing of applications while considering constraints, integrity, and diversity in the generated datasets. This approach results in more comprehensive and reliable testing outcomes.
Online Resource:
Faker provides a wide range of data providers for various data types, including names, addresses, text, numbers, dates, and more. You can explore the available providers in the official documentation.
Conclusion
The Python Faker library is a valuable tool for generating realistic-looking data quickly and easily, which can be useful in a variety of development and testing scenarios. Python Faker simplifies the process of creating realistic dummy data, enabling developers and testers to expedite testing and development tasks effectively. Armed with the knowledge of Faker’s functionalities, users can effortlessly generate diverse mock datasets for various applications.
That’s All Folks!
You can explore more of our Python guides here: Python Guides