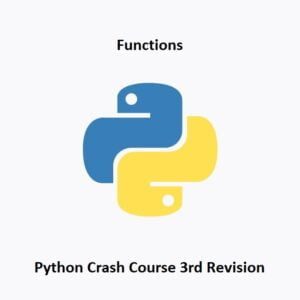
The Power of Functions for Code Organization and Reusability
Functions in Python serve as modular blocks of code designed to perform specific tasks. They encapsulate logic, promote code reuse, and enhance the maintainability of programs. Understanding functions is key to writing structured, efficient, and scalable code. Let’s explore the world of functions with this Python Crash Course. Learn their syntax, parameters, return values, and their pivotal role in code organization and reuse.
Functions
In Python, def
is a keyword used to define functions. Functions are blocks of reusable code that perform a specific task. Defining functions allows you to break down your code into smaller, more manageable pieces and promote code reusability.
Basic Syntax:
def function_name(parameter1, parameter2, ...): # Function body # Code statements # Return statement (optional)
Let’s break down each part of the function definition:
def
: It is the keyword used to start the function definition.function_name
: It is the name you choose for your function. The name should be descriptive and follow the Python naming conventions.(parameter1, parameter2, ...)
: These are optional parameters or arguments that the function can accept. Parameters are like placeholders that can be used within the function to work with data.:
: It denotes the end of the function header and the start of the function body.Function body
: It consists of the code statements that define what the function does. This block of code is indented under thedef
statement.Return statement
(optional): It is used to specify the value that the function should return. If no return statement is present, the function returnsNone
by default.
Here’s an example of a simple function that calculates the square of a number:
def square(number): result = number ** 2 return result
Now, you can call the square
function and pass a number as an argument to get the square of that number:
result = square(5) print(result) # Output: 25
In this example, the function square
takes a parameter number
, calculates the square of the number using the exponentiation operator **
, and returns the result. The returned value is then assigned to the variable result
and printed to the console.
You can define functions with multiple parameters, default parameter values, variable-length arguments, and more, depending on your specific requirements.
Functions Quiz
Test Completed
Your final score: 0 / 0
Conclusion
Functions play a crucial role in Python programming, offering a structured way to encapsulate code and promote reusability. Their ability to streamline complex logic, handle different types of arguments, and return values make them fundamental building blocks for writing efficient and organized code. Mastery of functions equips programmers with the skills to create modular and scalable applications, enhancing code readability, maintainability, and promoting a more efficient development process.
In the next installment of this Python Crash Course, we will be learning all about Classes and Methods
That’s All Folks!
You can explore more of our Python guides here: Python Guides