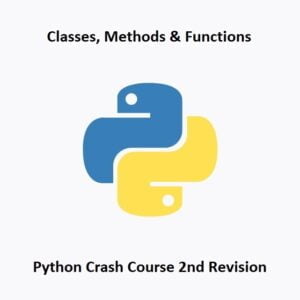
Functions, Classes, and Methods: Building Blocks of Structured Code
In the world of programming, organizing and managing code efficiently is paramount. Python offers powerful tools like functions, classes, and methods to help you structure your code, promote reusability, and create organized, maintainable applications. In this Python crash course, we’ll explore these fundamental building blocks.
Functions
Functions are blocks of reusable code that perform a specific task. They allow you to encapsulate logic and execute it with different inputs, promoting code reusability and readability. Here’s how you can define and use a function in Python:
def greet(name): return f"Hello, {name}!" message = greet("Alice") print(message)
In this example, we define a function greet
that takes a name
parameter and returns a greeting message, allowing us to greet different people with ease.
Classes and Objects
Classes serve as blueprints for creating objects in Python. Objects are instances of classes and encapsulate both data (attributes) and behavior (methods). They help you model real-world entities and their interactions. Here’s how to create a simple class and object:
class Person: def __init__(self, name, age): self.name = name self.age = age def introduce(self): return f"My name is {self.name}, and I am {self.age} years old." alice = Person("Alice", 30) print(alice.introduce())
In this code, we create a Person
class with attributes (name and age) and a method (introduce) to introduce the person.
Methods
Methods are functions defined within a class and are used to perform operations on the attributes of an object. They encapsulate behavior specific to the class. Here’s how you can create and use methods within a class:
class Circle: def __init__(self, radius): self.radius = radius def area(self): return 3.14 * self.radius * self.radius my_circle = Circle(5) print(f"The area of the circle is {my_circle.area()}")
In this example, we define a Circle
class with an area
method to calculate the area of a circle based on its radius.
Inheritance and Encapsulation
Python also supports inheritance and encapsulation, allowing you to create new classes by inheriting properties and methods from existing classes and restricting access to certain attributes and methods for improved data integrity.
By mastering functions, classes, and methods, you’ll be equipped to create well-structured, modular, and efficient Python programs. These concepts form the foundation of object-oriented programming and are crucial for designing complex, real-world applications.
In our next part of this Python crash course, we’ll explore how to work with Dates and Time. Stay tuned for more Python programming knowledge!
That’s All Folks!
You can explore more of our Python guides here: Python Guides