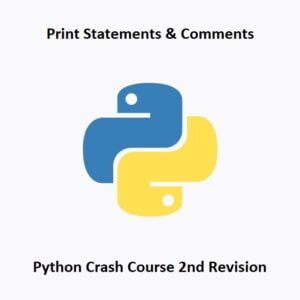
Print Statements and Comments
In the second part of our Python Crash Course, we’ll dive deeper into Python’s fundamentals. We’ll explore the power of print statements and the importance of comments in your code. These are essential skills for every Python programmer, regardless of your experience level. Let’s get started!
Printing Output with print()
:
The print()
function in Python is a versatile tool for displaying information on the screen. It’s an essential way to communicate with your code and check the results of your programs. Here’s how it works:
print("Hello, World!")
In this example, the print()
function displays the text “Hello, World!” on the screen. You can print text, numbers, variables, and the results of expressions.
Using Variables with print()
:
name = "Alice"
age = 30
print("My name is", name, "and I am", age, "years old.")
You can include variables in print()
statements to create dynamic output. Python automatically inserts spaces between the items you’re printing.
Comments: Why They Matter:
Comments are essential for documenting your code. They help you and others understand the purpose of your code, how it works, and why specific decisions were made. Comments are not executed by the Python interpreter and are only for human readers. Here’s how to add comments to your code:
# This is a single-line comment
Single-line comments start with a #
symbol and continue until the end of the line. They’re useful for brief explanations and annotations.
"""
This is a multi-line comment or docstring.
You can use it to provide more extensive documentation
for your code.
"""
Multi-line comments, or docstrings, are enclosed in triple quotes and are often used to document entire functions, classes, or modules.
Practical Tips:
Use comments to explain complex code or logic, making it easier to maintain and collaborate on projects.
Write clear and concise comments. A good comment explains “why” something is done, not just “what” it does.
Conclusion
In this second part of the Python Crash Course, you’ve learned about print statements and the importance of comments. These are foundational skills for any Python programmer and will be invaluable as you tackle more complex coding tasks. In the upcoming posts, we’ll explore variables, data types, and the power of control structures in Python. In the next part of our Python crash course, we will be learning about Variables. Stay tuned for more Python insights!
That’s All Folks!
You can explore more of our Python guides here: Python Guides