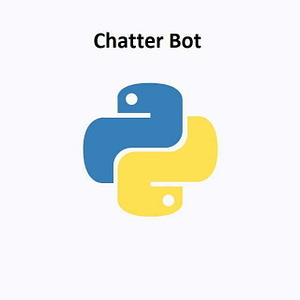
Creating Intelligent Chatbots
Welcome to the world of conversational AI! ChatterBot in Python offers a seamless way to create chatbots that can engage in intelligent conversations. In this comprehensive guide, we’ll explore ChatterBot’s functionalities, showcasing its ease of use and flexibility in designing chatbots. By the end, you’ll be equipped to build responsive and interactive conversational AI applications using ChatterBot.
What is ChatterBot?
The Python ChatterBot library is an open-source conversational AI library that allows developers to build chatbot applications. It provides a straightforward and easy-to-use interface for creating chatbots that can engage in natural language conversations with users.
How Does ChatterBot Work?
ChatterBot uses a combination of machine learning algorithms and predefined conversational patterns to generate responses to user inputs. It leverages a language-independent algorithm for training and can be easily adapted to support different languages.
The library offers various components and features, including:
Corpus Training Data:
ChatterBot comes with a built-in corpus of conversational data that can be used to train the chatbot. This corpus consists of various categories, such as greetings, jokes, and conversations on different topics.
Training:
Developers can train the chatbot using their custom datasets or the provided corpus data. ChatterBot utilizes a machine learning approach to learn from the training data and improve its conversational abilities over time.
Text Preprocessing:
ChatterBot preprocesses the input text by tokenizing and cleaning it before generating a response. This helps in normalizing the input and improving the accuracy of the chatbot’s responses.
Response Generation:
The library uses a combination of machine learning algorithms, including an implementation of the TF-IDF (Term Frequency-Inverse Document Frequency) algorithm, to generate responses based on the input text. It selects the most appropriate response from its knowledge base, considering the context of the conversation.
Training Adapters:
ChatterBot supports training adapters that allow integration with various machine learning libraries, such as TensorFlow and PyTorch. This enables developers to leverage advanced techniques and models for training their chatbots.
Language Support:
ChatterBot supports multiple languages and can be trained to understand and generate responses in different languages.
Overall, the ChatterBot library simplifies the process of creating chatbots by providing a high-level API and built-in features for training and generating responses. It has gained popularity among developers due to its ease of use and flexibility in building conversational agents.
Python Code
In this example, we use the ChatterBot library, which is a Python library for building chatbots.
from chatterbot import ChatBot from chatterbot.trainers import ChatterBotCorpusTrainer # Create a ChatBot instance chatbot = ChatBot('MyBot') # Create a new trainer for the chatbot trainer = ChatterBotCorpusTrainer(chatbot) # Train the chatbot on the English corpus trainer.train('chatterbot.corpus.english') # Main loop for chatting with the bot print("Chat with the AI Bot! Type 'exit' to end the conversation.") while True: user_input = input("User: ") if user_input.lower() == 'exit': break bot_reply = chatbot.get_response(user_input) print("Bot:", bot_reply)
Breaking Down the Code
- We create a
ChatBot
instance named ‘MyBot’. Then, we create aChatterBotCorpusTrainer
to train the chatbot using the pre-existing English corpus included in ChatterBot. - The
train()
method is used to train the chatbot on the English corpus. This step is optional, and you can add your own custom training data or additional language corpora to improve the bot’s responses. - The main loop continuously prompts the user for input and gets a response from the chatbot using the
get_response()
method. Typing “exit” as the user input will break the loop and end the conversation.
Keep in mind that this is a simplified example, and you can further customize and enhance the chatbot according to your specific requirements. ChatterBot provides various options for customization, including training with additional datasets, configuring different storage backends, and more.
Conclusion
Congratulations on exploring ChatterBot! You’ve discovered a powerful tool to create conversational AI effortlessly. As you continue your journey, experiment with different training datasets, customization options, and conversation flows within ChatterBot. With its capabilities, you’re empowered to develop engaging and intelligent chatbot applications in Python.
That’s All Folks!
You can explore more of our Python guides here: Python Guides