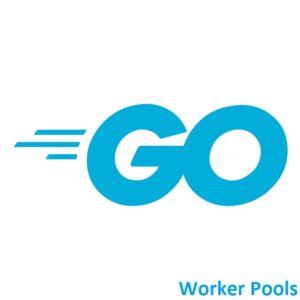
Optimizing Parallel Processing!
Welcome to the world of Golang! A Worker pool is a common concurrency pattern used to manage a fixed number of goroutines (worker goroutines) to process a queue of tasks concurrently. Worker pools are especially useful when you have a large number of tasks to execute concurrently, and you want to limit the number of goroutines running at any given time to control resource usage.
Here’s a step-by-step guide on how to create a worker pool in Go:
Define the Task Structure
First, define a struct that represents the task you want to execute. This structure should include all the necessary data for a worker to perform the task. For example:
type Task struct { ID int Data interface{} }
Create a Pool of Worker Goroutines
You’ll need to create a fixed number of worker goroutines that will process tasks from a channel. You can use a goroutine and a for loop to accomplish this:
func worker(id int, tasks <-chan Task) { for task := range tasks { // Perform the task here fmt.Printf("Worker %d processing task %d\n", id, task.ID) // Process task.Data as needed } }
Start the Worker Pool
In your main function or where you want to use the worker pool, create a channel to pass tasks to the worker pool, start the worker goroutines, and start sending tasks to the channel:
func main() { numWorkers := 3 // Adjust the number of worker goroutines as needed tasks := make(chan Task, numWorkers) // Start worker goroutines for i := 1; i <= numWorkers; i++ { go worker(i, tasks) } // Add tasks to the channel for i := 1; i <= 10; i++ { task := Task{ID: i, Data: "Some data for task " + strconv.Itoa(i)} tasks <- task } // Close the tasks channel to signal that no more tasks will be added close(tasks) // Wait for all worker goroutines to finish waitGroup := sync.WaitGroup{} waitGroup.Add(numWorkers) for i := 0; i < numWorkers; i++ { go func() { defer waitGroup.Done() for range tasks { // Worker goroutine cleanup, if needed } }() } waitGroup.Wait() }
Breaking Down the Code
- We create a
tasks
channel to send tasks to worker goroutines. - We start the worker goroutines in a loop, each calling the
worker
function. - We add tasks to the
tasks
channel. - We close the
tasks
channel to signal that no more tasks will be added. - We use a
sync.WaitGroup
to wait for all worker goroutines to finish processing tasks.
The code creates a simple worker pool with three worker goroutines that process ten tasks concurrently. You can adjust the number of worker goroutines and tasks as per your requirements.
Conclusion
Worker pools in GoLang provide a robust framework for concurrent task execution, enhancing performance and scalability in various applications. Leveraging their capabilities can significantly improve the efficiency of parallel processing in Go programs.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang