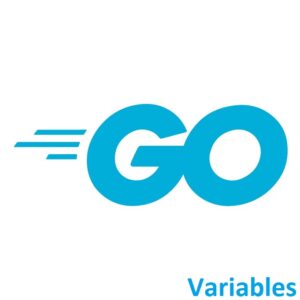
The Building Blocks of Dynamic Programs
Welcome to the world of Golang! In this guide we’ll delve into the fundamentals of variables, covering their types, and best practices for effective variable management in Golang.
Variable Types
In Go programming language, variables are used to store and manage data values. Variables have explicit types that are determined at compile-time. This enhances code clarity, performance, and safety. Here are some of the basic variable types in Go:
Numeric Types:
int
andint64
: Signed integers.uint
anduint64
: Unsigned integers.float32
andfloat64
: Floating-point numbers.complex64
andcomplex128
: Complex numbers.
String Types:
string
: A sequence of bytes (characters).
Boolean Type:
bool
: Represents true or false values.
Derived Types:
byte
: Alias foruint8
.rune
: Alias forint32
, represents a Unicode code point.
Composite Types:
array
: Fixed-size collection of items with the same type.slice
: Dynamic-size view into an underlying array.map
: Unordered collection of key-value pairs.struct
: Composite data type grouping together fields of different types.interface
: Specifies a method set that a concrete type must have.
Pointer Types:
*T
: A pointer to a value of typeT
.
Function Types:
func
: Represents functions as first-class citizens.
Channel Types:
chan T
: A channel for communicating values of typeT
between goroutines.
Error Type:
error
: Represents an error condition.
It’s important to note that Go is statically typed, which means that you must declare the type of a variable when you define it.
Variable Declaration:
Variables are declared using the var
keyword, followed by the variable name and its type.
var age int // Declaration of an integer variable named 'age' var name string // Declaration of a string variable named 'name'
You can also declare and initialize variables in a single line:
var score int = 100 // Declare and initialize 'score' with value 100
Go also supports type inference, where the variable’s type is automatically determined based on the value you assign:
var count = 5 // 'count' is automatically inferred as int
Short Variable Declaration:
The :=
operator allows you to declare and initialize a variable without explicitly specifying the type:
message := "Hello, Go!" // Short declaration of 'message' with inferred type string
Constants:
Constants are declared using the const
keyword and cannot be changed after they are defined:
const pi = 3.14159 const daysInWeek = 7
Variable Naming Conventions:
Variable names in Go follow the convention of using camelCase (first letter lowercase for private variables, uppercase for public variables) and should be descriptive.
var firstName string var lastName string var isRunning bool
Type Aliases:
Type aliases allow you to create alternative names for existing types. They are especially useful when you want to give a more descriptive name to a type or for backward compatibility:
type MyInt int // Creating a type alias for int called 'MyInt' var value MyInt = 42
Multiple Variable Declaration:
You can declare multiple variables in a single var
block:
var x, y, z int // Declaring multiple integer variables
Zero Values:
Variables that are declared without an explicit value are assigned a default “zero” value based on their type. For example, numeric types are assigned 0, strings are assigned an empty string (""
), and booleans are assigned false
.
var num int // num is assigned 0 var flag bool // flag is assigned false var word string // word is assigned ""
Important: Variables must be used after they are declared, otherwise, your code will not compile. Unused variables will result in a compilation error in Go.
Here’s a simple example illustrating variable usage:
package main import "fmt" func main() { var age int age = 30 name := "Alice" fmt.Printf("Name: %s, Age: %d\n", name, age) }
In the example above, the program declares and initializes variables for a person’s age and name, and then prints them out using the fmt.Printf
function.
Conclusion
Congratulations on mastering the fundamentals of variables in Golang! Variables serve as the bedrock of dynamic programming, enabling data storage, manipulation, and flexible code execution. By understanding their types, and adopting best practices, you’re equipped to write more robust and maintainable Golang programs.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang