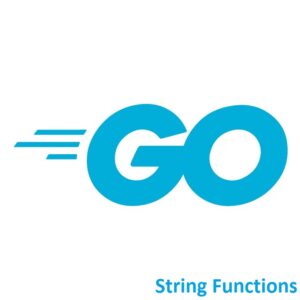
Exploring Essential String Functions!
Welcome to the world of Golang! String manipulation is a fundamental aspect of text processing in Go programming. Understanding and utilizing string functions can significantly enhance text handling capabilities. This guide explores a variety of essential string functions available in Go, empowering developers to manipulate and process strings effectively.
Here are some commonly used string functions and methods in Go:
len(str)
int
Returns the length (number of bytes) of the string.
str := "Hello, World!" length := len(str) // length will be 13
str[index]
byte
Accesses a specific byte in the string at the given index.
str := "Hello, World!" char := str[0] // char will be 'H'
strings.Contains(s, substr string)
bool
Checks if a string contains a specific substring and returns a boolean.str := "Hello, World!" contains := strings.Contains(str, "World") // contains will be true
strings.Index(s, substr string)
int
Returns the index of the first occurrence of a substring in a string, or -1 if not found.str := "Hello, World!" index := strings.Index(str, "World") // index will be 7
strings.Split(s, sep string) []
string
Splits a string into a slice of substrings based on a separator.str := "apple,banana,cherry" fruits := strings.Split(str, ",") // fruits will be []string{"apple", "banana", "cherry"}
strings.Join(strs []string, sep string)
string
Joins a slice of strings into a single string using a separator.fruits := []string{"apple", "banana", "cherry"} str := strings.Join(fruits, ",") // str will be "apple,banana,cherry"
strings.ToLower(str)
string
Converts a string to lowercase.str := "Hello, World!" lower := strings.ToLower(str) // lower will be "hello, world!"
strings.ToUpper(str)
string
Converts a string to uppercase.str := "Hello, World!" upper := strings.ToUpper(str) // upper will be "HELLO, WORLD!"
strings.TrimSpace(str)
string
Removes leading and trailing whitespace from a string.str := " Trim Me " trimmed := strings.TrimSpace(str) // trimmed will be "Trim Me"
strconv.Itoa(int)
string
Converts an integer to its decimal string representation.num := 42 str := strconv.Itoa(num) // str will be "42"
strings.Repeat(s string, count int)
string
Repeats a string count
times and returns the resulting string.
str := "abc" repeated := strings.Repeat(str, 3) // repeated will be "abcabcabc"
strings.Replace(s, old, new string, n int)
string
Replaces all occurrences of a substring old
with a new substring new
, up to n
replacements. If n
is negative, it replaces all occurrences.str := "Hello, World!" newStr := strings.Replace(str, "World", "Golang", -1) // newStr will be "Hello, Golang!"
strings.Count(s, substr string)
int
Counts the number of non-overlapping occurrences of a substring in a string.str := "abaababa" count := strings.Count(str, "aba") // count will be 2
Conclusion
These are some of the most commonly used string functions and methods in Go. However, Go’s standard library provides many more string manipulation functions and methods to handle various string operations efficiently. You can explore the Go documentation for more details on working with strings in Go: https://pkg.go.dev/strings
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang