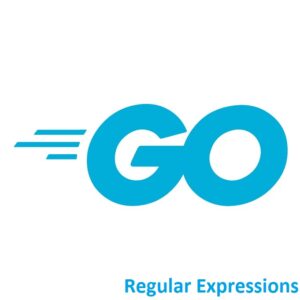
Unleashing Pattern-Matching Magic!
Welcome to the world of Golang! Regular expressions (regex) serve as a versatile tool for pattern matching and text manipulation in Golang. This guide dives into the world of regular expressions, exploring their syntax, functions, and practical applications. Learn how to wield regex effectively to search, validate, and extract data from text effortlessly in your Go programs.
Regular expressions are supported through the regexp
package, which allows you to work with regular expressions to match and manipulate strings.
Here’s an overview of how to use regular expressions in Go:
Import the regexp
Package:
You need to import the regexp
package at the beginning of your Go program to use regular expressions.
import "regexp"
Compile a Regular Expression:
You can create a regular expression object by using the regexp.Compile()
function, which compiles the regex pattern into a *regexp.Regexp
object. For example:
regex, err := regexp.Compile(`\d{3}-\d{2}-\d{4}`) if err != nil { // Handle error }
In this example, we are compiling a regex pattern that matches a Social Security Number (SSN) format.
Match a String Against a Regular Expression:
You can use the MatchString()
method of the compiled regular expression to check if a string matches the pattern:
text := "123-45-6789" if regex.MatchString(text) { fmt.Println("Match found!") } else { fmt.Println("No match found.") }
Find all Matches in a String:
To find all occurrences of a pattern in a string, you can use the FindAllString()
method:
text := "John: 123-45-6789, Jane: 987-65-4321" matches := regex.FindAllString(text, -1) // -1 means find all matches for _, match := range matches { fmt.Println("Found match:", match) }
Find and Replace with Regular Expressions:
The ReplaceAllString()
method can be used to replace matches in a string with a replacement string:
text := "John: 123-45-6789, Jane: 987-65-4321" replaced := regex.ReplaceAllString(text, "[REDACTED]") fmt.Println(replaced)
Capturing Groups:
You can use capturing groups to extract specific parts of a matched string. To create a capturing group, enclose the part of the pattern you want to capture in parentheses. Then, you can use the FindAllStringSubmatch()
method to access captured groups:
regex := regexp.MustCompile(`(\d{3})-(\d{2})-(\d{4})`) text := "John: 123-45-6789, Jane: 987-65-4321" matches := regex.FindAllStringSubmatch(text, -1) for _, match := range matches { fmt.Println("Full Match:", match[0]) fmt.Println("Group 1:", match[1]) fmt.Println("Group 2:", match[2]) fmt.Println("Group 3:", match[3]) }
Regex Best Practices:
Readability and Clarity:
Write regex patterns that are easy to understand and maintain. Use comments ((?#...)
) to document complex patterns and improve readability for yourself and other developers.
Avoid Over-Complexity:
Break down complex patterns into smaller, more manageable components. This makes patterns easier to comprehend and debug.
Specificity and Precision:
Craft regex patterns that are as specific as possible to match the intended content accurately. Avoid overly broad patterns that might unintentionally match unwanted text.
Testing and Validation:
Thoroughly test your regex patterns against various sample texts to ensure they produce the expected results. Consider edge cases and corner scenarios to verify the pattern’s robustness.
Escape Special Characters:
Escape special characters that should be treated literally. For example, if you’re searching for a dot (.
) in the text, use \.
to match the actual dot character.
Optimize Performance:
Use compiled regex patterns (regexp.Compile
) for patterns that will be used multiple times. Compiling regex patterns ahead of time improves performance by avoiding recompilation on each use.
Use Case-Specific Flags:
Utilize regex flags ((?i)
, (?m)
, etc.) as needed based on the specific requirements of the pattern. For instance, (?i)
makes the pattern case insensitive.
Avoid Catastrophic Backtracking:
Be cautious with nested quantifiers and complex alternations that can cause exponential backtracking, leading to poor performance. Refactor such patterns to be more efficient.
Following these best practices helps create maintainable, robust, and efficient regular expressions. By focusing on readability, specificity, and performance optimization, you can ensure that your regex patterns effectively serve their intended purpose without unintended side effects or performance bottlenecks.
Conclusion
These are the basics of working with regular expressions using the regexp
package. Remember to handle errors that may occur during regex compilation or other operations for robust code. Regular expressions are a powerful tool for manipulating and processing text in Golang. With a solid understanding of regex syntax, functions, and best practices, you’re equipped to leverage this versatile tool to its fullest potential in your programs.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang