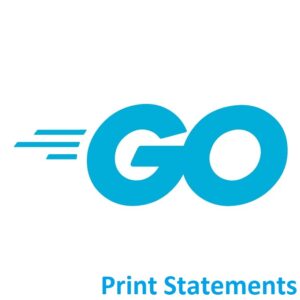
Enhance Debugging and Code Understanding
Welcome to the world of Golang! Print statements serve as invaluable tools for debugging, tracing, and understanding code flow. In this guide we’ll explain the basics of how to write print statements.
Using Print Statements
In the Go programming language (often referred to as Golang), you can use the fmt
package to print statements to the console.
Here’s how you can use it:
Print to Standard Output:
Use the fmt.Print()
function to print text to the standard output without a newline character at the end.
package main import "fmt" func main() { fmt.Print("Hello, ") fmt.Print("Golang!") }
Print with Newline:
Use the fmt.Println()
function to print text to the standard output with a newline character at the end.
Formatted Print:
Use the fmt.Printf()
function to print formatted text. You can use format verbs to specify how values should be formatted and inserted into the string.
package main import "fmt" func main() { name := "Alice" age := 30 fmt.Printf("Name: %s, Age: %d\n", name, age) }
Print to String:
If you want to capture the printed text into a string variable, you can use fmt.Sprintf()
.
package main import "fmt" func main() { greeting := fmt.Sprintf("Hello, %s!", "Gopher") fmt.Println(greeting) }
Debugging with Print Statements
Using print statements for debugging in Golang involves strategically inserting messages or variable values within your code to track its execution flow and identify issues.
Here’s a breakdown:
Understanding Code Flow:
By strategically placing print statements at various checkpoints in your code, you gain visibility into how your program progresses during runtime. This helps understand the sequence of operations and detect any unexpected behavior.
Inspecting Variable Values:
Print statements allow you to display the values of variables at specific points in your code. This is particularly helpful in tracking changes to variable states and identifying any discrepancies between expected and actual values.
Conditional Debugging:
You can use print statements within conditional blocks to verify whether certain conditions are being met or to understand which branch of code is being executed. This aids in pinpointing potential logic errors.
Formatted Output for Clarity:
Utilizing fmt.Printf()
allows you to format the output, making it more readable and informative. This is beneficial when displaying multiple variables or complex messages.
Tracing Loops and Function Execution:
Placing print statements within loops or functions assists in tracking the iteration process or understanding the flow within functions. This helps visualize repetitive processes or the sequence of function calls.
Temporary Debugging Aid:
It’s crucial to remove or comment out excessive debug print statements once you’ve resolved the issue. This ensures that your code remains clean and maintains readability for future development.
In summary, print statements serve as a simple yet effective tool for gaining insights into your code’s behavior during runtime. They allow you to observe and analyze the program flow, variable states, and execution paths, aiding in identifying and resolving issues efficiently.
Conclusion
Congratulations on learning the basics of print statements in Golang! By mastering print statement techniques, you’ve gained a powerful tool to debug, trace, and understand your code more effectively. Thoughtful use of print statements can significantly streamline your development process and enhance code comprehension. Remember to include the import "fmt"
statement at the beginning of your Go source file to use the formatting functions from the fmt
package. Keep experimenting with these techniques to become a debugging maestro in Golang!
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang