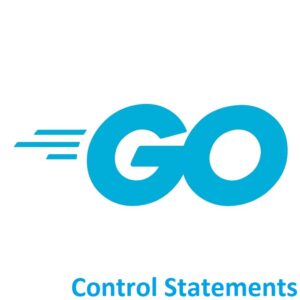
Orchestrating Program Flow with Precision!
Welcome to the world of Golang! Control statements are fundamental to directing program flow and making decisions in Go programming. Understanding the nuances and usage of control structures is pivotal for efficient code execution and logic implementation. This comprehensive guide navigates through the concepts and applications of control statements in Go, empowering developers to wield these structures effectively.
Go has several control statements that allow you to control how your code is executed.
Here are some of the key control statements in Go:
If Statement:
The if
statement is used to execute a block of code conditionally. It follows this format:
if condition { // code to be executed if condition is true } else { // code to be executed if condition is false }
For Loop:
Go has only one type of loop, the for
loop, which can be used in various ways:
// Basic for loop for i := 0; i < 10; i++ { // code to be executed in each iteration } // for loop used as a while loop for condition { // code to be executed as long as condition is true } // Infinite loop for { // code to be executed repeatedly }
Switch Statement:
The switch
statement allows you to evaluate an expression against multiple possible values:
switch expression { case value1: // code to be executed if expression == value1 case value2: // code to be executed if expression == value2 default: // code to be executed if none of the cases match }
Defer Statement:
The defer
statement is used to schedule a function call to be executed when the surrounding function returns, regardless of how it returns (whether normally or with an error):
func main() { defer fmt.Println("This will be printed last") fmt.Println("This will be printed first") }
Break Statement:
The break
statement is used to immediately exit the innermost loop (for loop, switch statement, or select statement) it’s placed within. It allows you to prematurely terminate the loop’s execution.
for i := 0; i < 10; i++ { if i == 5 { break // This will exit the loop when i is 5 } fmt.Println(i) }
Continue Statement:
The continue
statement is used to skip the rest of the current iteration of a loop and move on to the next iteration. It doesn’t terminate the loop entirely, but rather skips the remaining code within the loop for the current iteration.
for i := 0; i < 10; i++ { if i == 5 { continue // This will skip the iteration when i is 5 } fmt.Println(i) }
Return Statement:
The return
statement is used to exit a function and return a value (if the function has a return type). It can be used to pass a result back from a function to the caller. When a return
statement is encountered, the function’s execution is terminated.
func add(a, b int) int { return a + b } func main() { result := add(3, 5) // This will return 8 and assign it to the "result" variable fmt.Println(result) }
Remember that break
and continue
are used within loops, while return
is used within functions.
Goto Statement:
The goto
statement allows you to jump to a labeled statement within the same function. However, the use of the goto
statement is generally discouraged in modern programming because it can make code harder to understand and maintain. Go’s designers purposely limited its use to prevent excessive and confusing use of goto
.
Here’s the basic syntax for using goto
in Go:
package main import "fmt" func main() { goto label fmt.Println("This won't be printed") label: fmt.Println("Jumped to label") }
In this example, the program will immediately jump to the label
using the goto
statement, bypassing the fmt.Println
statement above it.
Again, it’s worth noting that using goto
can lead to less readable and maintainable code, and it’s recommended to use more structured control flow constructs like if
, for
, and switch
instead. These constructs make your code easier to understand and reason about.
Conclusion
These are some of the fundamental control statements in the Go programming language. They allow you to control the flow of your program and make it more flexible and dynamic. Control statements form the backbone of decision-making and flow control in Go programming. Mastery of these structures equips developers with the tools to orchestrate logic, make decisions, and manage program flow efficiently.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang