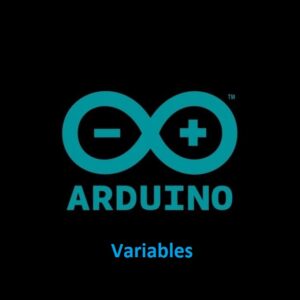
In Arduino programming, variables are used to store and manipulate data. Arduino variables are fundamental to writing code that controls various hardware components and responds to input from sensors or user interactions.
Here are some key points about Arduino variables:
Data Types:
Arduino supports several data types for variables, including:
- int: Represents signed integers (whole numbers).
- float: Represents floating-point numbers (numbers with decimal points).
- char: Represents a single character.
- boolean: Represents a binary value (either true or false).
You can also create user-defined data types using struct
.
Variable Declaration:
Typically, you declare variables at the beginning of your sketch (program) before the setup()
and loop()
functions. For example:
int ledPin = 13; // Declare an integer variable and assign it the value 13
float temperature; // Declare a float variable without assigning an initial value
char myChar = 'A'; // Declare a character variable and initialize it with the character 'A'
Scope:
The scope of a variable determines where in the code it can be used. Variables declared within a function or block of code are local variables and can only be accessed within that function or block. When variables are declared outside of any function, they are global variables and can be accessed throughout the entire sketch.
Constants:
In addition to variables, Arduino also supports constants, which are values that don’t change during the execution of the program. Constants are declared using the const
keyword. For example:
const int ledPin = 13; // Declare a constant integer variable
Variable Manipulation:
You can perform various operations on variables, such as addition, subtraction, multiplication, and division, depending on the data type. For example:
int x = 5;
int y = 3;
int sum = x + y; // Adds x and y, storing the result in 'sum'
Type Casting:
When you need to convert a variable from one data type to another, you can use type casting. For example, you can cast a float
to an int
or vice versa to perform specific calculations.
float temperature = 25.5;
int tempInt = (int)temperature; // Cast the float 'temperature' to an integer
Variable Naming:
Variable names should start with a letter, followed by letters, digits, or underscores. They are case-sensitive, so myVar
and myvar
are considered different variables.
Memory Usage:
Keep in mind that Arduino boards have limited memory (RAM and Flash), so efficient use of variables is important to avoid running out of resources.
Conclusion
Understanding how to use variables effectively is crucial for programming Arduino projects, as they allow you to store and manipulate data, making your sketches responsive and functional.
Happy Tinkering!
Recommendations:
If you don’t already own any Arduino hardware, we highly recommend purchasing the Elegoo Super Starter Kit. This kit has everything you need to start programming with Arduino.
You can find out more about this kit here: Elegoo Super Starter Kit