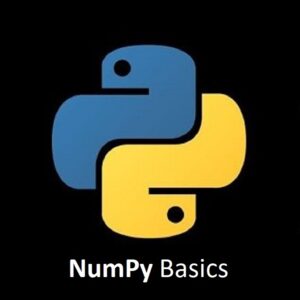
The Foundation of Scientific Computing
Welcome to the realm of scientific computing with Python NumPy, a fundamental library, serves as the backbone for numerical operations and data manipulation. In this beginner-friendly guide, we embark on an exploration of NumPy, delving into its powerful array structures and rich suite of mathematical functions. By the end, you’ll wield the tools to efficiently handle data, perform complex computations, and unlock new possibilities in Python.
What is Python NumPy?
NumPy is a powerful Python library for numerical computing. It stands for “Numerical Python” and provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays efficiently. NumPy is a fundamental package for scientific computing with Python.
Here are some key features of NumPy:
Multi-dimensional Arrays:
NumPy provides a powerful array
object for creating and manipulating multi-dimensional arrays. These arrays can be of different shapes and sizes, and they enable efficient storage and computation of large datasets.
Mathematical Operations:
NumPy includes a wide range of mathematical functions that can be applied to arrays element wise. These functions operate efficiently on arrays without the need for explicit loops, making numerical computations much faster.
Broadcasting:
NumPy allows arrays with different shapes to be operated together smoothly through broadcasting. Broadcasting enables efficient computation on arrays of different sizes without the need for explicitly duplicating data.
Linear Algebra:
NumPy provides a set of linear algebra functions, such as matrix multiplication, eigenvalues, singular value decomposition (SVD), and solving linear equations. These functions make it easy to perform various linear algebra operations efficiently.
Integration with Other Libraries:
NumPy is a foundational library for many other scientific computing libraries in Python, such as SciPy, pandas, and scikit-learn. It provides a common data structure that allows seamless integration and interoperability between these libraries.
Installation
To use NumPy in your Python program, you need to install it first. You can install NumPy using pip, the Python package manager, by running the following command:
pip install numpy
Python NumPy Example Code
Now, you can use NumPy functions and create NumPy arrays using the np
namespace.
Here’s a basic example to create a NumPy array and perform some mathematical operations on it:
import numpy as np # Create a NumPy array arr = np.array([1, 2, 3, 4, 5]) # Perform mathematical operations on the array print(np.mean(arr)) # Calculate the mean print(np.max(arr)) # Find the maximum value print(np.sin(arr)) # Apply the sine function to each element
Python NumPy Example Code
Here’s a simple practical example demonstrating how NumPy can be used to perform basic array operations:
import numpy as np # Creating NumPy arrays array1 = np.array([1, 2, 3, 4, 5]) array2 = np.array([6, 7, 8, 9, 10]) # Basic arithmetic operations result_sum = array1 + array2 # Element-wise addition result_diff = array2 - array1 # Element-wise subtraction result_prod = array1 * array2 # Element-wise multiplication result_div = array2 / array1 # Element-wise division # Displaying results print("Array 1:", array1) print("Array 2:", array2) print("Array 1 + Array 2:", result_sum) print("Array 2 - Array 1:", result_diff) print("Array 1 * Array 2:", result_prod) print("Array 2 / Array 1:", result_div) # Reshaping arrays array3 = np.array([[1, 2, 3], [4, 5, 6]]) reshaped_array = array3.reshape(3, 2) # Reshaping the array # Transposing arrays transposed_array = np.transpose(array3) # Displaying reshaped and transposed arrays print("\nOriginal Array 3:") print(array3) print("Reshaped Array 3:") print(reshaped_array) print("Transposed Array 3:") print(transposed_array)
This example showcases:
- Creating NumPy arrays
- Performing basic arithmetic operations (addition, subtraction, multiplication, division) element-wise between arrays
- Reshaping arrays
- Transposing arrays
This is just a simple demonstration; NumPy offers a wide range of functionalities for array manipulation, mathematical operations, statistics, and more. This example gives a glimpse of how NumPy arrays can be used and manipulated efficiently for various tasks.
What Next?
This is just a brief introduction to NumPy. It offers many more functionalities, such as array slicing, reshaping, random number generation, and more. NumPy has a rich ecosystem of resources, tutorials, and communities that can help beginners get started and provide advanced insights for seasoned users.
Here are some valuable online resources for NumPy:
Documentation: The official documentation is an excellent place to start. It offers comprehensive explanations, tutorials, and examples: NumPy Documentation
User Guide: The user guide provides in-depth explanations and examples of NumPy’s functionalities, making it a great resource for both beginners and experienced users: NumPy User Guide
NumPy GitHub Repository: NumPy’s GitHub repository is where you can find the source code, contribute to development, and report issues: NumPy GitHub Repository
Stack Overflow: There’s an active community on Stack Overflow discussing NumPy-related questions and issues. It’s an excellent place to find solutions and ask questions: NumPy questions on Stack Overflow
NumPy Discourse: The NumPy Discourse forum is a platform for discussing development, usage, and announcements related to NumPy: NumPy Discourse Forum
Exploring these resources will provide you with a wealth of information, tutorials, and avenues to engage with the NumPy community. Whether you’re just starting or looking to enhance your expertise, these platforms offer valuable insights and support.
Conclusion
Congratulations on delving into the world of Python NumPy! You’ve unlocked a crucial tool for scientific computing, equipped with the prowess to handle arrays, perform intricate mathematical operations, and streamline data analysis in Python. As you continue your journey, keep experimenting with NumPy’s functionalities, explore its vast capabilities, and apply its tools to various data-driven tasks. With NumPy, you’ve laid a solid foundation for mastering scientific computing in Python.
That’s All Folks!
You can explore more of our Python guides here: Python Guides