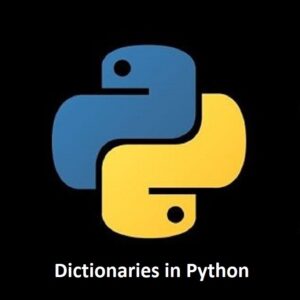
Mastering Key-Value Pairs and Efficient Data Mapping
Welcome to the world of Python! Dictionaries serve as a powerful tool for data storage and retrieval. In this comprehensive guide, we’ll explore Python dictionaries, delving into their functionality and versatility in mapping key-value pairs. By the end, you’ll wield the capabilities of dictionaries, empowering you to handle dynamic data structures effectively in Python.
What are Dictionaries?
Python dictionaries are a fundamental data structure used to store and manage collections of data in a way that allows for efficient retrieval and modification. Dictionaries are also known as associative arrays or hash maps in other programming languages.
Here are the key aspects of Python dictionaries you should know:
Definition:
A dictionary in Python is defined using curly braces {}
or the dict()
constructor. It consists of key-value pairs, where each key is unique and associated with a value. The key is used to look up the corresponding value.
# Creating an empty dictionary my_dict = {} # Creating a dictionary with key-value pairs student = { "name": "Alice", "age": 25, "grade": "A" }
Accessing Values:
You can access the values in a dictionary using square brackets []
and providing the key.
print(student["name"]) # Output: "Alice" print(student["age"]) # Output: 25
Modifying Values:
You can change the value associated with a key in a dictionary by assigning a new value to it.
student["age"] = 26
Adding New Key-Value Pairs:
You can add new key-value pairs to a dictionary by simply assigning a value to a new key.
student["city"] = "New York"
Removing Key-Value Pairs:
You can remove a key-value pair from a dictionary using the del
keyword or the pop()
method.
del student["grade"] # Deletes the "grade" key and its value popped_value = student.pop("city") # Removes the "city" key and returns its value
Checking for Key Existence:
You can check if a key exists in a dictionary using the in
keyword.
if "name" in student: print("Name exists in the dictionary")
Iterating Over a Dictionary:
You can loop through the keys, values, or key-value pairs in a dictionary using for
loops.
for key in student: print(key, student[key]) # Using items() to iterate through key-value pairs for key, value in student.items(): print(key, value)
Dictionary Methods:
Python dictionaries come with several useful methods such as keys()
, values()
, items()
, get()
, clear()
, copy()
, and more. These methods help you manipulate and work with dictionaries efficiently.
keys_list = student.keys() # Returns a list of keys values_list = student.values() # Returns a list of values
Dictionary Comprehensions:
You can create dictionaries using dictionary comprehensions, which are similar to list comprehensions but for dictionaries.
squares = {x: x*x for x in range(1, 6)} # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
Python dictionaries are highly flexible and efficient for many tasks. They are commonly used in various programming scenarios, such as storing configuration settings, counting occurrences of items, and representing structured data. Understanding how to work with dictionaries is a valuable skill for Python developers.
Conclusion
Congratulations on mastering Python dictionaries! You’ve gained a fundamental understanding of a versatile data structure crucial for data manipulation. Python dictionaries are highly flexible and efficient for many tasks. They are commonly used in various programming scenarios, such as storing configuration settings, counting occurrences of items, and representing structured data. Understanding how to work with dictionaries is a valuable skill for Python developers. As you continue your Python journey, experiment with dictionaries’ various methods, nested structures, and application in different scenarios. With dictionaries, you possess a powerful tool for efficient data mapping and manipulation in Python.
That’s All Folks!
You can explore more of our Python guides here: Python Guides