What is Crypto?
Cryptocurrency is a digital or virtual form of currency that has various use cases and applications. Here are some of the primary purposes and uses of crypto:
Digital Payments: Cryptocurrency can be used as a medium of exchange for goods and services, similar to traditional currencies. Many online and even some physical merchants accept cryptocurrencies as a form of payment. Cryptocurrency transactions can be faster and cheaper than traditional banking methods, especially for international transactions.
Investment: Some people buy and hold cryptocurrencies as an investment, hoping that the value of the cryptocurrency will increase over time. This is often referred to as “HODLing.” Cryptocurrencies have shown the potential for significant price volatility, which can present both opportunities and risks for investors.
Store of Value: Some individuals view cryptocurrencies, particularly Bitcoin, as a store of value similar to gold. They see cryptocurrencies as a hedge against inflation and economic instability. The limited supply of many cryptocurrencies can make them appealing as a store of value.
Remittances: Cryptocurrencies can be used to send remittances or cross-border payments. This can be especially useful for individuals who need to send money internationally, as it can be faster and cheaper than traditional remittance services.
Fundraising (Initial Coin Offerings – ICOs): Companies and projects can raise capital by creating and selling their own cryptocurrencies or tokens through Initial Coin Offerings (ICOs) or token sales. Investors purchase these tokens as an investment in the project or company.
Smart Contracts: Some cryptocurrencies, like Ethereum, have the capability to execute smart contracts. Smart contracts are self-executing contracts with the terms of the agreement directly written into code. They can automate various processes, such as legal agreements, without the need for intermediaries.
Privacy and Anonymity: Certain cryptocurrencies, like Monero and Zcash, are designed to provide enhanced privacy and anonymity for transactions. These coins are often used by individuals who prioritize privacy in their financial transactions.
Gaming and Virtual Goods: Cryptocurrencies are increasingly used in the gaming industry for purchasing virtual goods, in-game assets, and digital collectibles. These assets are often represented as non-fungible tokens (NFTs) on blockchain platforms.
Cross-Border Trade: Cryptocurrencies can facilitate cross-border trade and international business transactions by providing a universal and borderless form of payment.
Financial Inclusion: Cryptocurrencies have the potential to provide financial services to people who are unbanked or underbanked, especially in regions with limited access to traditional banking infrastructure.
It’s important to note that the use and acceptance of cryptocurrencies can vary significantly by region and can be subject to regulatory and legal considerations. Cryptocurrencies also have their own unique risks, including price volatility and security concerns, which individuals should consider when using them for various purposes.
Build Your own Crypto Trackers
We can utilize the power of Python to get real-time updates on the values of different cryptocurrencies.
Basic Crypto Tracker
The Code:
import requests from datetime import datetime import time def trackBtc(): url='https://min-api.cryptocompare.com/data/price?fsym=BTC&tsyms=USD,GBP,EUR' response=requests.get(url).json() print('Bitcoin'+str(response)) def trackXmr(): url='https://min-api.cryptocompare.com/data/price?fsym=XMR&tsyms=USD,GBP,EUR' response=requests.get(url).json() print('Monero'+str(response)) def trackEth(): url='https://min-api.cryptocompare.com/data/price?fsym=ETH&tsyms=USD,GBP,EUR' response=requests.get(url).json() print('Ethereum'+str(response)) def trackDog(): url='https://min-api.cryptocompare.com/data/price?fsym=DOGE&tsyms=USD,GBP,EUR' response=requests.get(url).json() print('Doge Coin'+str(response)) while True: myTime=datetime.now().strftime('%H:%M:%S') print('Crypto Tracker') print('') print('Last Updated at:'+str(myTime)) trackBtc() trackEth() trackXmr() trackDog() print('') time.sleep(4)
The Result:
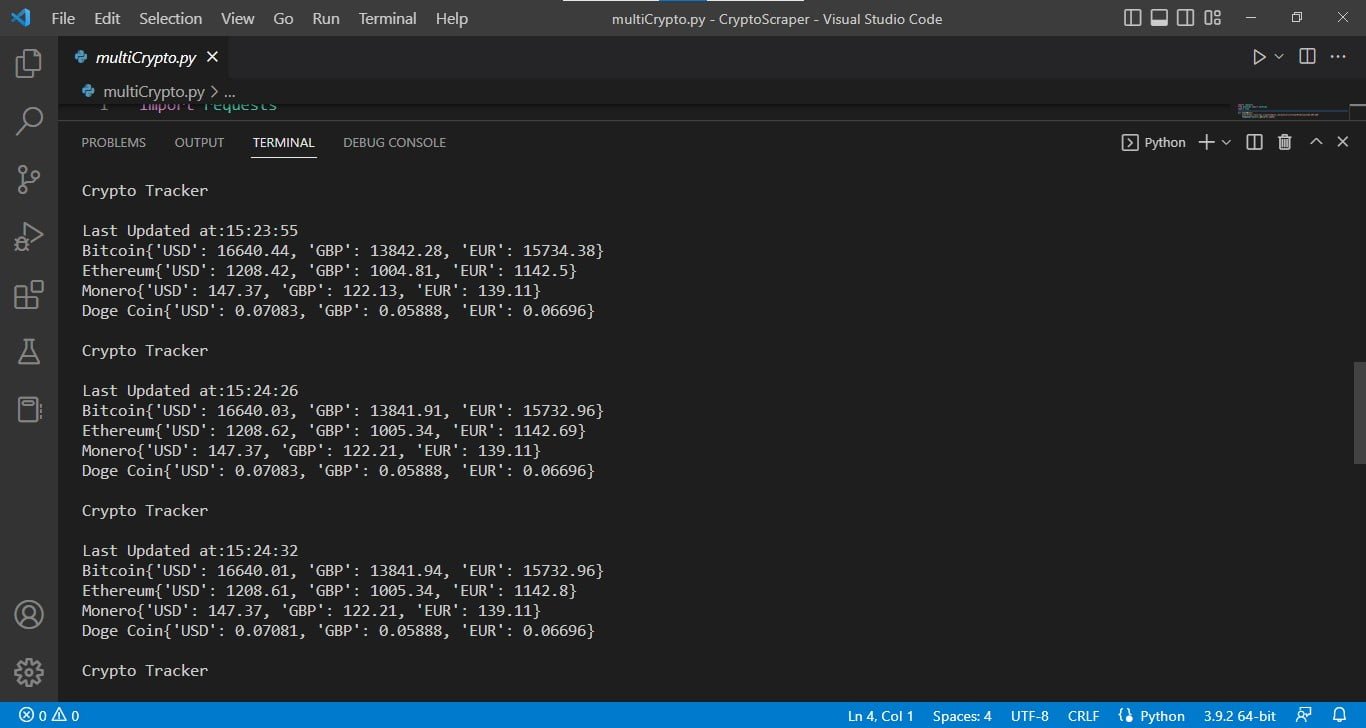
Creating Crypto Trackers with TKinter
Bitcoin (BTC) Tracker:
The Code:
import requests import tkinter as tk from datetime import datetime def trackBtc(): url='https://min-api.cryptocompare.com/data/price? fsym=BTC&tsyms=USD,GBP,EUR' response=requests.get(url).json() print(response) price=response['GBP'] time=datetime.now().strftime('%H:%M:%S') labelPrice.config(text='£'+str(price)) labelTime.config(text='Last Update @ '+time) canvas.after(10000, trackBtc) canvas=tk.Tk() canvas.geometry('400x300') canvas.title('Bitcoin Price') f1=('poppins', 24, 'bold') f2=('poppins', 22, 'bold') f3=('poppins', 18, 'normal') label=tk.Label(canvas, text='Bitcoin Price ', font=f1) label.pack(pady=20) labelPrice=tk.Label(canvas,font=f2) labelPrice.pack(pady=20) labelTime=tk.Label(canvas, font=f3) labelTime.pack(pady=20) trackBtc() canvas.mainloop()
The Result:
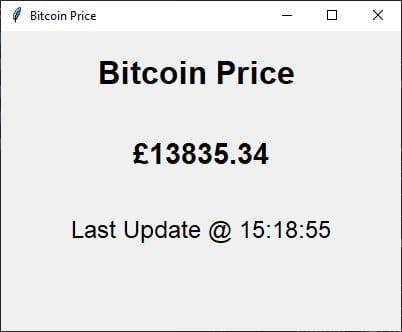
Doge Coin Tracker
The Code:
import requests import tkinter as tk from datetime import datetime def trackDoge(): url='https://min-api.cryptocompare.com/data/price?fsym=DOGE&tsyms=USD,GBP,EUR' response=requests.get(url).json() print(response) price=response['GBP'] time=datetime.now().strftime('%H:%M:%S') labelPrice.config(text='£'+str(price)) labelTime.config(text='Last Update @ '+time) canvas.after(10000, trackDoge) canvas=tk.Tk() canvas.geometry('400x300') canvas.title('Doge Coin Price') f1=('poppins', 24, 'bold') f2=('poppins', 22, 'bold') f3=('poppins', 18, 'normal') label=tk.Label(canvas, text='Doge Coin Price ', font=f1) label.pack(pady=20) labelPrice=tk.Label(canvas,font=f2) labelPrice.pack(pady=20) labelTime=tk.Label(canvas, font=f3) labelTime.pack(pady=20) trackDoge() canvas.mainloop()
The Result:
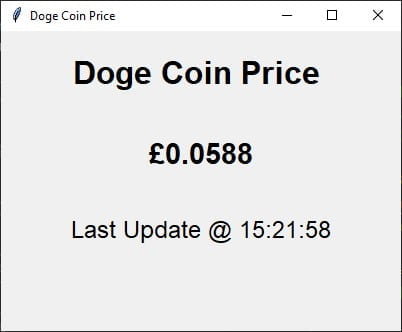
Ethereum Tracker
The Code:
import requests import tkinter as tk from datetime import datetime def trackETH(): url='https://min-api.cryptocompare.com/data/price?fsym=ETH&tsyms=USD,GBP,EUR' response=requests.get(url).json() print(response) price=response['GBP'] time=datetime.now().strftime('%H:%M:%S') labelPrice.config(text='£'+str(price)) labelTime.config(text='Last Update @ '+time) canvas.after(10000, trackETH) canvas=tk.Tk() canvas.geometry('400x300') canvas.title('Ethereum Price') f1=('poppins', 24, 'bold') f2=('poppins', 22, 'bold') f3=('poppins', 18, 'normal') label=tk.Label(canvas, text='Ethereum Price ', font=f1) label.pack(pady=20) labelPrice=tk.Label(canvas,font=f2) labelPrice.pack(pady=20) labelTime=tk.Label(canvas, font=f3) labelTime.pack(pady=20) trackETH() canvas.mainloop()
The Result:
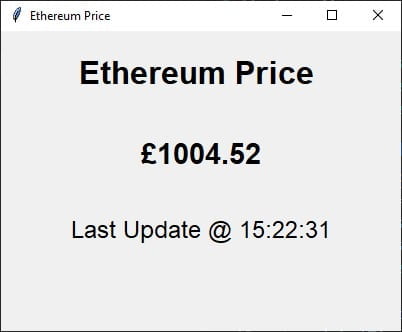
Monero Tracker
The Code:
import requests import tkinter as tk from datetime import datetime def trackMon(): url='https://min-api.cryptocompare.com/data/price?fsym=XMR&tsyms=USD,GBP,EUR' response=requests.get(url).json() print(response) price=response['GBP'] time=datetime.now().strftime('%H:%M:%S') labelPrice.config(text='£'+str(price)) labelTime.config(text='Last Update @ '+time) canvas.after(10000, trackMon) canvas=tk.Tk() canvas.geometry('400x300') canvas.title('Monero Price') f1=('poppins', 24, 'bold') f2=('poppins', 22, 'bold') f3=('poppins', 18, 'normal') label=tk.Label(canvas, text='Monero Price ', font=f1) label.pack(pady=20) labelPrice=tk.Label(canvas,font=f2) labelPrice.pack(pady=20) labelTime=tk.Label(canvas, font=f3) labelTime.pack(pady=20) trackMon() canvas.mainloop()
The Result:
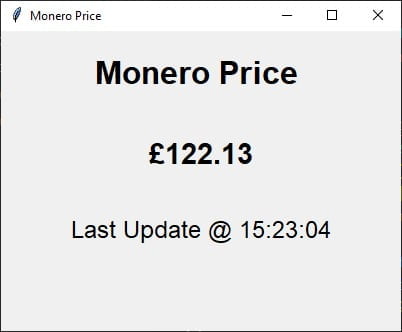
Conclusion
Through this project, we’ve not only gained valuable experience in web scraping and data manipulation but also learned how to create visually appealing and functional applications. With this newfound knowledge, the possibilities are endless. You can customize your crypto tracker further, add features like historical data analysis, or even integrate it with other financial tools.
As the world of cryptocurrencies continues to evolve, having the ability to track and analyze your investments in real-time becomes increasingly important. By building your own crypto tracker, you’ve taken a significant step toward financial empowerment. Whether you’re a seasoned trader or a crypto enthusiast, this project serves as a solid foundation for creating tools that can aid you in your cryptocurrency journey. “HODL onto your dreams”
That’s All Folks!
Recommendation:
The Elegoo Super Starter Kit
If you don’t already own any Arduino hardware, we highly recommend this kit as it has everything you need to start programming with Arduino. You can find out more about this kit, including a list of its components here: Elegoo Super Starter Kit
You can find this kit on Amazon here: Elegoo Super Starter Kit