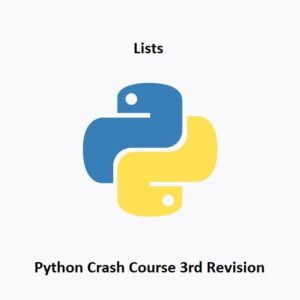
Versatile Arrays for Data Handling
Lists in Python are versatile and dynamic containers used to store collections of items. They offer a flexible way to organize and manipulate data, allowing for easy access, insertion, deletion, and modification of elements. Let’s delve into the world of lists with this Python crash course guide, learning their properties, methods, and applications in handling diverse datasets within programming.
Python Lists
Python lists are a fundamental data structure in Python used to store a collection of items. Lists are mutable, which means you can modify them by adding, removing, or changing elements. They are defined by enclosing comma-separated values within square brackets.
Basic Example of Creating a List:
my_list = [1,2,3,4,5]
In this case, my_list
is a list containing the numbers 1, 2, 3, 4, and 5.
Lists can contain elements of different types, such as numbers, strings, or even other lists.
For Example:
mixed_list = [1, "apple", True, 3.14]
nested_list = [[1,2],[3,4,5],[6,7,8,9]]
You can access individual elements of a list using indexing. The index starts from 0 for the first element, 1 for the second element, and so on.
Negative indices can be used to access elements from the end of the list.
For Example:
my_list = [1,2,3,4,5]
print(my_list[0]) # Output: 1
print(my_list[-1]) # Ouput: 5
You can also slice a list to get a subset of its elements. Slicing allows you to specify a range of indices to extract multiple elements.
For Example:
my_list = [1,2,3,4,5]
print(my_list[1:4]) # Ouput: [2, 3, 4]
List Manipulation
Lists support various operations and methods to manipulate their contents. Some commonly used operations include:
Adding Elements:
You can append an element to the end of a list using the append()
method or concatenate two lists using the +
operator.
Removing Elements:
You can remove elements from a list using the remove()
method or delete elements by their index using the del
statement.
Modifying Elements:
You can assign new values to individual elements by indexing.
Length and Membership:
You can find the length of a list using the len()
function and check if an element is present in a list using the in
operator.
Sorting Elements:
You can sort the elements of a list in order using the sort()
function.
For Example:
my_list.sort()
Lists Quiz
Test Completed
Your final score: 0 / 0
Conclusion
These are just a few basic concepts related to Python lists. There are many more advanced operations and methods available to work with lists, such as reversing, or copying etc… Lists stand as foundational data structures in Python, offering a dynamic means to store, organize, and manipulate data. Mastery of lists allows programmers to efficiently handle collections of information, enabling the creation of robust and flexible applications. With their versatility and extensive functionalities, Python lists serve as invaluable tools for managing diverse datasets and streamlining data-centric operations within programming tasks.
In the next installment of this Python Crash Course, we will be learning about Tuples
That’s All Folks!
You can explore more of our Python guides here: Python Guides