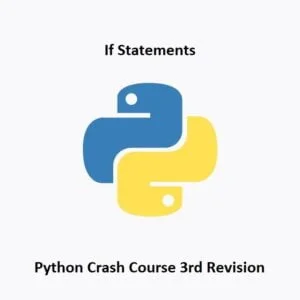
Logic for Smart Programming
Conditional statements in Python allow programs to make decisions based on certain conditions. They form the backbone of control flow, enabling code to execute different actions depending on whether specific conditions are true or false. Let’s delve into conditional statements with this Python crash course guide. Understand their syntax, types, and applications in creating responsive and adaptive programs.
Conditional Statements
If Statement
In Python, if
statements are used for conditional execution. They allow you to control the flow of your program based on certain conditions.
Basic Syntax of an if
Statement:
if condition: # Code to be executed if the condition is True else: # Code to be executed if the condition is False
The condition
is an expression that evaluates to either True
or False
. If the condition is True
, the code block under the if
statement is executed. If the condition is False
, the code block under the else
statement (if present) is executed.
Elif Statement
You can also have multiple conditions using elif
(short for “else if”) statements.
Here’s an Example:
if condition 1: # Code to be executed if the condition is True elif condition 2: # Code to be executed if condition 1 is False and condition 2 is True else: # Code to be executed if both conditions is False
Practical Usage of if
Statements:
age = 25 if age < 18: print("You are a minor") elif age >= 18 and <65: print("You are an adult") else: print("You are a senior citizen")
In the above example, the code checks the value of the age
variable and prints a corresponding message based on the age range.
Logical Operators
Logical operators in Python are essential tools for combining multiple conditions to make complex decisions. These operators work with Boolean values (True or False) and help control the flow of your code based on logical evaluations.
AND Operator (and
):
The and
operator returns True only if both the operands are True; otherwise, it returns False. It’s commonly used to check if multiple conditions are simultaneously true.
x = 5 y = 10 if x > 0 and y < 15: print("Both conditions are true")
OR Operator (or
):
The or
operator returns True if at least one of the operands is True. It evaluates the expressions from left to right and stops when it encounters the first True value.
age = 25 if age < 18 or age >= 65: print("You might be eligible for special discounts")
NOT Operator (not
):
The not
operator is a unary operator that returns the opposite Boolean value. It negates the given condition; if it’s True, not
makes it False, and vice versa.
has_passed_exam = True if not has_passed_exam: print("You need to retake the exam")
Combining Logical Operators:
Logical operators can be combined to create more complex conditions by using parentheses to control the evaluation order.
x = 10 y = 20 z = 30 if (x > y) and (y < z) or (x == z): print("Complex condition met")
Short-Circuit Evaluation:
Python uses short-circuit evaluation with and
and or
. With and
, if the first expression is False, Python doesn’t evaluate the second (as the overall result will be False regardless). Similarly, with or
, if the first expression is True, Python doesn’t evaluate the second.
a = 5 b = 0 if b != 0 and a/b > 2: print("This won't get executed due to short-circuiting")
Logical operators provide powerful tools to create sophisticated conditions in Python. Understanding how to combine and
, or
, and not
allows developers to build complex decision-making structures within their code, facilitating more intelligent and adaptable applications.
If Statements Quiz
Test Completed
Your final score: 0 / 0
Conclusion
Logical operators serve as crucial building blocks within conditional statements, empowering programmers to create dynamic decision-making structures in Python. Through the use of and
, or
, and not
operators, developers can combine multiple conditions, forming intricate logical evaluations within if, else, and elif statements. This fusion of logical operators with conditional statements unlocks the ability to craft responsive, adaptable, and sophisticated programs. Mastery of these tools not only facilitates code that intelligently responds to diverse scenarios but also lays the groundwork for creating robust, logic-driven applications in Python.
In the next installment of this Python Crash Course, we will be learning about Lists
That’s All Folks!
You can explore more of our Python guides here: Python Guides