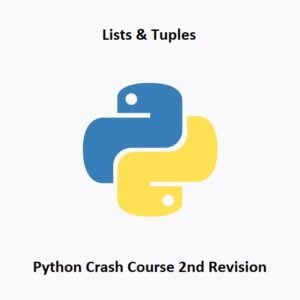
Python Data Structures: Lists, Dictionaries, and More
Data structures are the backbone of any programming language, allowing you to store, organize, and manipulate data efficiently. Python provides a rich collection of built-in data structures, each with its own unique features. In this part of our Python crash course, we’ll dive into some of the most commonly used data structures in Python.
Lists
Lists are one of the most versatile and frequently used data structures in Python. They are ordered collections of items and are defined by enclosing elements in square brackets []
. Here’s how you can create and work with lists:
fruits = ["apple", "banana", "cherry"] fruits.append("date") print(fruits)
In this example, we create a list of fruits, add an item using append()
, and print the modified list.
Dictionaries
Dictionaries are key-value pairs that allow you to store and access data using unique keys. They are defined using curly braces {}
and consist of keys and their corresponding values. Here’s how to work with dictionaries:
person = { "name": "Alice", "age": 30, "city": "New York" } print(person["name"])
This code defines a dictionary with information about a person and retrieves the value associated with the “name” key.
Sets
Sets are unordered collections of unique elements. They are defined using curly braces {}
or the set()
constructor. Sets are excellent for performing mathematical set operations like union, intersection, and difference. Here’s an example:
fruits = {"apple", "banana", "cherry"} fruits.add("date") print(fruits)
In this example, we create a set of fruits and add an item using the add()
method.
Tuples
Tuples are similar to lists but are immutable, meaning their elements cannot be changed after creation. They are defined using parentheses ()
. Tuples are useful for data that should not be modified. Here’s a simple tuple example:
coordinates = (3, 4) x, y = coordinates print(f"The x-coordinate is {x} and the y-coordinate is {y}")
In this code, we define a tuple for coordinates and unpack its values into x
and y
.
More Data Structures
Python offers additional data structures like queues, stacks, and linked lists through libraries and modules. These structures cater to specific needs, such as implementing advanced data storage and retrieval.
A bit of Fun!
I have written a Python script to show you a fun way you can use Python lists, there will be some stuff in there you haven’t learnt here yet or is even covered in this course but still, it’s a fun script and I want to give you inspiration to make your own projects. Go check out My Star Wars Trumps game here: Star Wars Top Trumps Game
Conclusion
By mastering Python’s diverse data structures, you can efficiently manage and manipulate data for a wide range of applications, from simple lists to complex, real-world scenarios.
In our next installment of this Python crash course, we’ll explore Functions and how they can help modularize your code and improve its maintainability. Stay tuned for more Python programming knowledge!
That’s All Folks!
You can explore more of our Python guides here: Python Guides