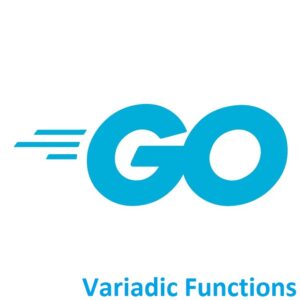
Enhancing Functionality and Adaptability
Welcome to the world of Go programming! Variadic functions enable developers to work with a variable number of arguments, providing adaptability and versatility in function design. In this comprehensive guide, we’ll explore variadic functions in Golang, showcasing their usage and advantages in handling varying argument lists. By the end, you’ll wield the tools to create functions that adapt to different argument counts seamlessly.
What is a Variadic Function?
In Go, a variadic function is a function that can accept a variable number of arguments. This is achieved by using the ellipsis (...
) before the type of the last parameter in the function signature.
Here’s the basic syntax for defining a variadic function:
func functionName(arg1 Type1, arg2 Type2, ...argN TypeN) ReturnType { // Function body }
In this syntax:
functionName
is the name of the function.arg1
,arg2
, etc., are regular function parameters....argN TypeN
indicates that the function can accept a variable number ofTypeN
arguments. You can use any valid data type forTypeN
.
Golang Code
Here’s an example of a variadic function in Go:
package main import "fmt" func sum(nums ...int) int { result := 0 for _, num := range nums { result += num } return result } func main() { fmt.Println(sum(1, 2, 3)) // Output: 6 fmt.Println(sum(4, 5, 6, 7, 8)) // Output: 30 fmt.Println(sum()) // Output: 0 }
In the sum
function, nums
is a variadic parameter of type int
. It can accept a variable number of integer arguments, and the function calculates the sum of all the provided integers.
Conclusion
Congratulations on delving into variadic functions in Go! You’ve gained insight into a powerful feature that enhances adaptability in function design. Variadic functions are a flexible way to work with functions that need to handle a different number of arguments. Inside the function, nums
is treated as a slice, allowing you to iterate over the provided values. As you continue your programming journey, experiment with variadic functions in various scenarios, exploring their flexibility in handling different argument counts efficiently. With variadic functions, you have a versatile tool at your disposal to optimize your Go code.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang