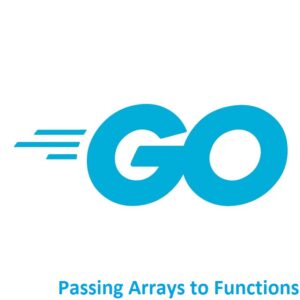
Exploring Efficient Functionality with Arrays
Welcome to the world of Go programming! Arrays are fundamental data structures, and understanding their passing mechanisms in functions is crucial for efficient code in Go. In this beginner-friendly guide, we’ll explore how arrays are passed to functions in Go, showcasing their efficiency and impact on code optimization. By the end, you’ll have a deeper grasp of handling arrays within functions in the Golang programming language.
Passing Arrays to Functions
In Go, arrays are fixed-size sequences of elements with a specific data type. You can pass arrays to functions just like you pass any other data type. When you pass an array to a function, you are actually passing a copy of the array.
Here’s how you can pass an array to a function in Go:
package main import "fmt" // Function that takes an array as a parameter func processArray(arr [5]int) { // Modify the array for i := 0; i < len(arr); i++ { arr[i] *= 2 } }
func main() { // Declare and initialize an array myArray := [5]int{1, 2, 3, 4, 5}
fmt.Println("Before:", myArray)
// Call the function with the array as an argument processArray(myArray)
fmt.Println("After:", myArray) }
In this example, the processArray
function takes an array of integers as a parameter. When you call processArray(myArray)
, a copy of myArray
is made and passed to the function. Inside the function, you can modify the elements of the array, but those changes will not affect the original array outside the function.
Modifying the Array Inside a Function
If you want to modify the original array inside the function, you can pass a pointer to the array.
Here’s how you can do that:
package main import "fmt" // Function that takes a pointer to an array as a parameter func processArray(arr *[5]int) { // Modify the array for i := 0; i < len(*arr); i++ { (*arr)[i] *= 2 } } func main() { // Declare and initialize an array myArray := [5]int{1, 2, 3, 4, 5} fmt.Println("Before:", myArray) // Call the function with a pointer to the array processArray(&myArray) fmt.Println("After:", myArray) }
In this example, processArray
takes a pointer to an array as a parameter. When you call processArray(&myArray)
, you are passing a pointer to the original array, so any changes made inside the function will affect the original array as well.
Conclusion
Congratulations on exploring the nuances of passing arrays to functions in Go! You’ve gained insight into a crucial aspect of Go programming, equipped to optimize your code through efficient array handling. As you continue your journey, experiment with different array sizes and types, exploring their behavior when passed as function parameters. With this knowledge, you’re better equipped to leverage arrays effectively in your Go projects.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang