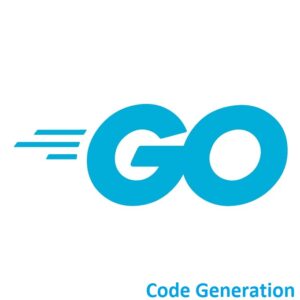
Automated Code Generation Techniques!
Welcome to the world of Golang! Code generation is a technique where you write code to generate other code. This can be useful in various scenarios such as reducing repetitive tasks, improving code maintainability, and automating the generation of boilerplate code.
There are several tools and libraries in the Go ecosystem that can help with code generation:
text/template
The Go standard library includes a package called text/template
that allows you to create templates for generating code. You can use this package to define templates for Go code and then populate them with data to generate code files.
html/template
Similar to text/template
, this package allows you to generate HTML or other text-based files using templates. It’s often used for generating web templates or configuration files.
go generate
Go has a built-in command called go generate
that can be used to run code generation scripts. You can write custom Go code generation scripts and then use the //go:generate
comment in your source code to specify when and how they should be run. text/template
Example
Here’s a simple example of using text/template
for code generation:
package main import ( "os" "text/template" ) type Person struct { Name string Age int } func main() { tmpl := ` package main import "fmt" func main() { p := Person{Name: "{{.Name}}", Age: {{.Age}}} fmt.Printf("Name: %s, Age: %d\n", p.Name, p.Age) } ` data := Person{Name: "John", Age: 30} t := template.Must(template.New("main").Parse(tmpl)) err := t.Execute(os.Stdout, data) if err != nil { panic(err) } }
In this example, we define a Go struct Person
and a template string for generating a Go program that prints a person’s name and age. We then use the text/template
package to fill in the template with data from a Person
struct.
To run this code, save it to a Go file (e.g., main.go
) and then run go run main.go
. It will generate and execute Go code based on the template.
html/template
Example
Here’s a simple example demonstrating the usage of html/template
to render an HTML page:
First, create an HTML template file named index.html:
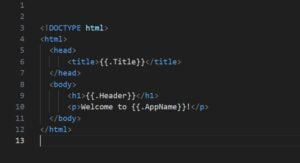
Next, in your Go code, use the html/template
package to parse the HTML template and execute it with specific data:
package main import ( "html/template" "os" ) type PageData struct { Title string Header string AppName string } func main() { // Sample data to pass to the template data := PageData{ Title: "Sample Page", Header: "Welcome Header", AppName: "My Go App", } // Parse the HTML template file tmpl, err := template.ParseFiles("index.html") if err != nil { panic(err) } // Execute the template with the provided data err = tmpl.Execute(os.Stdout, data) if err != nil { panic(err) } }
Ensure that the index.html
file is in the same directory as your Go code. This code parses the index.html
template file, defines sample data using the PageData
struct, and executes the template, replacing the placeholders ({{.Title}}
, {{.Header}}
, {{.AppName}}
) with the corresponding values from the PageData
struct.
When you run this Go program, it will render the HTML page to the console with the provided data.
Modify the HTML template and the data in the PageData
struct as needed for your specific use case or application. Additionally, you can execute the template to generate HTML output to an HTTP response or a file by passing an io.Writer
as the second argument to Execute
.
go generate
Code Example
Let’s say you have a template file that defines a data structure, and you want to generate Go code representing that structure.
First, create a template file named data_template.txt
:
package generated type Data struct { ID int Name string }
Next, create a Go file generate.go
with a go:generate
directive using a template-based code generation approach:
//go:generate go run gen.go package main import ( "io/ioutil" "os" "text/template" ) func main() { // Read the content of the template file templateContent, err := ioutil.ReadFile("data_template.txt") if err != nil { panic(err) } // Create a new Go file to write the generated code outFile, err := os.Create("generated_data.go") if err != nil { panic(err) } defer outFile.Close() // Define the data to be used in the template data := struct { PackageName string }{ PackageName: "generated", } // Parse the template tmpl, err := template.New("dataTemplate").Parse(string(templateContent)) if err != nil { panic(err) } // Execute the template with the provided data and write to the output file err = tmpl.Execute(outFile, data) if err != nil { panic(err) } }
In this example, the generate.go
file contains the code that reads the content of data_template.txt
, parses it as a Go template, and generates a Go file named generated_data.go
based on the provided template and data.
The go:generate
directive in generate.go
specifies that when you run go generate
, it should execute go run gen.go
, where gen.go
is the file containing the code for code generation.
To trigger the code generation, navigate to the directory containing generate.go
and data_template.txt
in your terminal, and execute:
go generate
This will execute the code in generate.go
, which reads the template file, applies the data, and generates a new Go file named generated_data.go
based on the template. The generated file will contain the defined data structure (Data
) in the generated
package.
This is a basic example. In real-world scenarios, you might use more complex templates and data structures to generate entire sets of code, such as API clients, database access layers, or serialization/deserialization logic.
Conclusion
Code generation can be a powerful technique, but it should be used judiciously. Overusing code generation can make your codebase harder to understand and maintain. It’s typically best suited for generating repetitive or boilerplate code, such as serializers, deserializers, or API client libraries.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang
Excellent post. I was checking constantly this blog and I’m impressed! Extremely useful info particularly the last part 🙂 I care for such information much. I was looking for this particular information for a very long time. Thank you and good luck.