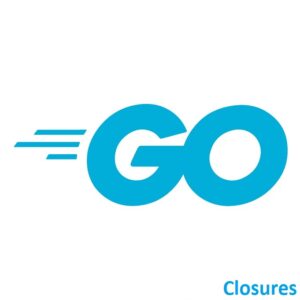
Exploring the Flexibility and Power of Closures
Welcome to the world of Golang! Closures are a powerful and essential concept. A closure is a function that captures and remembers the surrounding context in which it was created, allowing it to access and manipulate variables from that context even after that context is no longer in scope. Closures are often used in Go to create anonymous functions and are particularly useful in situations like callbacks and when working with goroutines (concurrent code).
Closure Best Practices in Golang:
Understanding Closure Scope:
Comprehend how closures capture variables from their enclosing scope. Avoid unexpected behavior by understanding the rules governing variable capture.
Avoiding Side Effects with Loops:
Be cautious when using closures within loops, especially with Goroutines. Capture loop variables explicitly to avoid unexpected behavior due to variable mutation.
Immutable Captured Variables:
Prefer capturing immutable variables to maintain predictability and prevent unintended modifications. Favor readability and avoid unexpected changes in captured variables.
Parameterizing Closures:
Encourage parameterization of closures by passing variables explicitly as arguments to avoid relying heavily on external variables. This promotes reusability and encapsulation.
Memory Management:
Be mindful of memory management when using closures. Avoid capturing large or unnecessary variables, as they may lead to increased memory consumption.
Documentation and Comments:
Document closures and their intended behavior with clear comments to assist other developers in understanding the purpose and usage of the closure, especially in complex scenarios.
Testing and Validation:
Validate closures in various scenarios to ensure they perform as expected. Test edge cases and different input scenarios to verify closure behavior comprehensively.
Encapsulation and Limiting Scope:
Encapsulate behavior and limit the scope of captured variables within closures. Minimize the exposure of captured variables to maintain cleaner code and reduce unexpected interactions.
Consistent Naming Conventions:
Follow consistent naming conventions for variables captured by closures. Use meaningful and descriptive variable names to enhance readability and comprehension.
Performance Optimization:
Optimize closures for better performance. Consider minimizing the number of captured variables and evaluating the lifespan of captured data to optimize memory usage.
Adhering to these best practices ensures that closures are used effectively, avoiding potential pitfalls, unexpected behavior, and memory-related issues. Understanding closure behavior and applying these practices enhances the reliability, readability, and maintainability of code utilizing closures in Golang.
Golang Code Example
Here’s a basic example to illustrate closures in Go:
package main import "fmt" func main() { // Define a function that returns another function (a closure). // The returned closure captures the variable "x" from its surrounding context. closure := outerFunction() // Call the closure. result := closure() fmt.Println(result) // Output: 10 } func outerFunction() func() int { x := 10 // Return a closure that can access the "x" variable. return func() int { return x } }
In this example, outerFunction
defines a variable x
, and it returns an anonymous function that can access and return the value of x
. When you call closure()
, it returns the value of x
, even though x
is no longer in scope within main
.
Conclusion
Closures in Golang offer a powerful way to encapsulate behavior and manage state, enhancing the language’s flexibility and enabling advanced programming techniques. Closures are frequently used for various purposes, such as creating custom iterators, handling callbacks, and managing state within functions. They are a key feature that allows Go code to be concise and expressive. By understanding closure behavior and best practices, developers can leverage closures effectively in their Go programs.
That’s All Folks!
You can find all of our Golang guides here: A Comprehensive Guide to Golang